Python Operators can be used to perform arithmetics or assignments of variables and values. Python has 21 tokens that serve as operators in its syntax.
What is a Python Operator?
In Python, an operator is a special symbol used to perform operations on variables and values. For example, the +
operator is used to perform additions on Python objects (e.g. 1 + 5
), while the ==
operator is used to verify if to values are equal.
Python Operators
Here is a quick guide to help you master Python operators.
Operators | Example |
Additions (+) | >>> 1+5 |
Substractions ( – ) | >>> 6-5 ##1 |
Multiplications (*) | >>> 2*5 10 |
Exponentiation (**) : Performs Exponential Calculation | >>> 4**2 |
Division ( / ) | >>> 10 / 2 5 |
Floor Division ( // ) | >>> 15 // 4 3 |
Modulo (%) : Returns the remainder | >>> 18 % 7 |
== : If x is equal y (x==y), returns true | >>> x == 7 True |
!= : If x is not equal to y (x!=y), returns true | >>> x != 7 True |
> : Left is greater than right, returns True | >>> 8 > 7 True |
< : Left is lower than right, returns True | >>> 8 < 7 False |
>= : Left is greater than or equal to right, returns True | >>> 8 >= 7 True |
<= : Left is lower than or equal to right, returns True | >>> <= 7 True |
:= (walrus operator) Assigns values to variables as part of the larger expression | if (n := len(a)) > 10: print(f”List is too long ({n} elements, expected <= 10)”) |
List of Python Operators
There are additional Python operators possible, here is the entire list of the Python Operators.
+ - * ** / // % @
<< >> & | ^ ~ :=
< > <= >= == !=
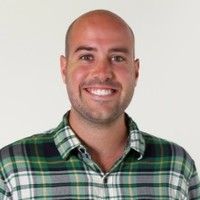
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.