In this Python for Beginners tutorial you will learn what Python functions are and how to create and use them.
What is a Python Function
A Python function is a portion of reusable code that can be used by calling its name with parentheses and arguments:
function_name(arguments)
Types of Functions in Python
There are two types of functions in Python:
- Built-in Functions (standard functions in the Python library)
- User-Defined Functions (created by the user inside code, often declared using def)
Python Built-in Functions
Python has a series of built-in functions that can be used. Built-in functions is a functions that are built-into the Python interpreter and that are always available without the need for importing them. Example of Python builtin functions are the sum(), int(), dict(), dir() and type().
Python Functions List
Here is a full list of Built-in Functions from Python.org
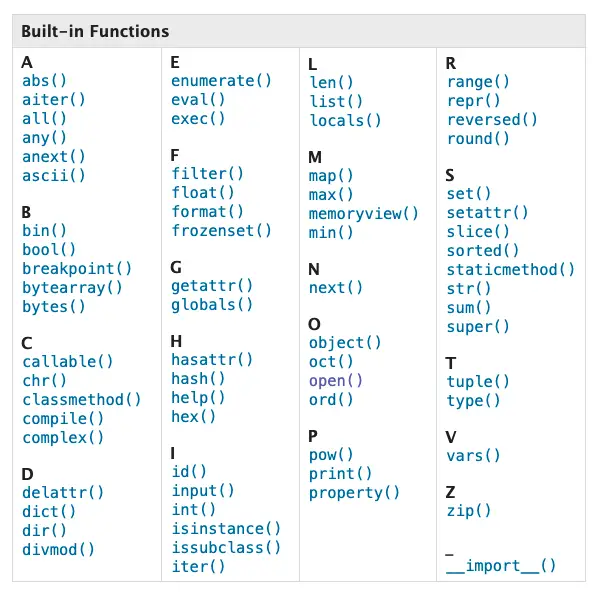
User-Defined Functions in Python
In Python, user-defined functions are functions created by the programmer using the def keyword just like in the pseudo-code below:
# User Defined Function
def user_defined_function(argument1, argument2, ...):
# statement
return [expression]
How to Use a Python Function (Example)
To use a function in Python, call the function by its name with parentheses containing the required arguments of the function. Additionally, any optional argument can be added to the function.
function_name(required_arguments, optional_arguments)
Example
# Using a function
ls = [1,2,3,4]
sum(ls)
10
Investigate How to use a Function
Pass the function name to the built-in the help() function to investigate what a function does and how to use it.
# Investigate a function
help(sum)
Help on built-in function sum in module builtins:
sum(iterable, /, start=0)
Return the sum of a 'start' value (default: 0) plus an iterable of numbers
When the iterable is empty, return the start value.
This function is intended specifically for use with numeric values and may
reject non-numeric types.
Assign the Result of a Function
Use the equal (=) operator to assign the result of a function to a variable in Python.
# Assign the result
total = sum(ls)
total
10
How to Create and Define a Function
To define a function in Python, use the def keyword, along with the function name followed by parentheses and a colon.
# Creating a simple Function
def function_name():
print('hello!')
Syntax of a Function Declaration
The syntax of function declarations in Python contain a number of things:
- def keyword: Keyword used to declare a function
- function name: Any name to give to the function
- function parameters (optional): Parameters to be used inside the function
- colon (:): Operator is the required character to begin a block of code in Python
- function body: What the code should do.
- function return (optional): Keyword used to declare the return statement
- return expression (optional): What the code should return
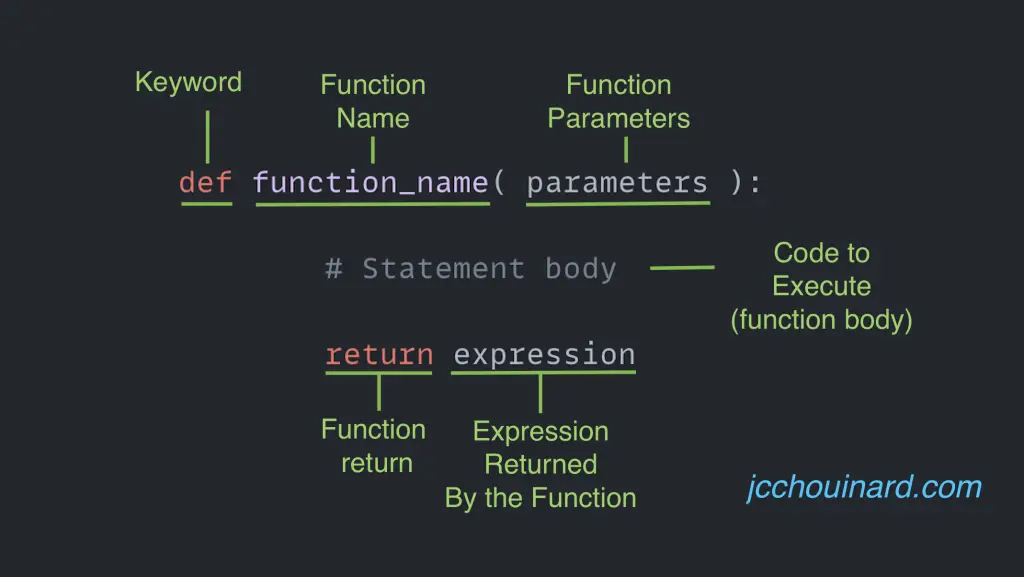
How to Call a Function in Python
To call a Python function, use the function name followed by parentheses and any required or optional arguments:
# Calling the Function
def function_name():
print('hello!')
function_name()
How to Add Arguments to a Python Function
To add arguments to a Python function, add the parameter name inside the parentheses of the function and use the same name to use it within the function.
# Function arguments
def say_something(arg):
print(arg)
say_something('hello')
hello
How to Add Multiple Arguments to a Python Function
To add multiple arguments to a Python function, add the parameter names, separated by commas between the parentheses of the function.
# multiple arguments
def count_this(num1, num2):
print(num1 + num2)
count_this(1, 3)
4
TypeError When Function is Missing Parameters
A function must be called with the correct number of arguments. Thus, if a function has 3 arguments, it must be called using 3 arguments. If not the function call will return the following error:
TypeError: function_name() missing 1 required positional argument: 'argument_name'
Just like in this code:
def say_something(arg):
print(arg)
say_something() # Throws a TypeError
How to Add Default Parameter Values to a Function
To add default parameter values to a function, call the variable name and use the equal operator to assign its value inside the parentheses:
# Default Parameter Values
def aussie_greeting(arg='Hi Mate!'):
print(arg)
aussie_greeting() # Uses default value
aussie_greeting("How's it Going, Mate?")
Hi Mate!
How's it Going, Mate?
How to Return Values In a Python Function
Use the return statement to end the execution of the function and return the result of the value of the expression stated after the return statement.
# return statement
def return_something():
result = 1 + 4
return result
return_something()
5
The return statement can’t be used outside a function.
What is the Default Expression of the Return Statement
If a there is no expression after the return statement, a value of None will be returned.
# return statement
def return_something():
result = 1 + 4
return
a = return_something()
type(a)
NoneType
How to Return Multiple Values in a Python Function
In Python, to return multiple values from a function separate each expression values in the return
statement by a comma. The output will be a Python Tuple.
def return_multiple():
return 'hello', 'world'
return_multiple()
('hello', 'world')
Keyword Arguments
It is possible to use keyword arguments when calling upon a Python function using the key = value syntax. The advantage of using the keyword argument notation is that the order of the arguments does not matter when calling the function this way.
# keyword argument
def divide_by(arg1, arg2):
return arg1 / arg2
divide_by(arg2 = 10, arg1=0)
Python Docstring
In Python, the documentation string, also known as docstring, is descriptive text used to tell what a block of code does.
A docstring can be used after a module, class, function or method definition.
It can be accessed by calling the object’s .__doc__
attribute.
print(function_name.__doc__)
In a Python function, the docstring is declared using triple quotes:
- triple single-quotes (
''' '''
) - triple double-quotes (
""" """
)
# Function with Docstring
def my_docstring():
"""This function returns 'hello'"""
return 'hello'
# access doc string
print(my_docstring.__doc__)
Python Function Pass
By definition, a Python function has to contain a function body or a return statement. Without it, the function will return SyntaxError: incomplete input
.
# Function that does nothing
def do_nothing():
# Throws SyntaxError
The pass statement can be used as a placeholder to the Python function so that the code does not break.
# Pass Statement
def do_nothing():
pass
How to Create Python Nested Functions
To define a function inside another function (nested or inner function) is similar to defining a regular function, but is indented within the body of another function. Nested functions are protected from thing happening outside the function and can access variables of the enclosing scope.
# nested functions
def parent_function():
s = 'Hello world'
def nested_function(s):
print(s)
nested_function(s)
# Calling the function
parent_function()
Hello world
Arbitrary Keyword Arguments
In Python, *args and **kwargs are arbitrary keyword arguments that passes a variable number of arguments to a function.
*Args in Python: Non-Keyword Arguments
The *args
parameter allows a function to accept an variable number of non-keyword positional arguments. Elements are collected inside a tuple called args:
def show_args(*args):
print(args)
show_args(1,2,3)
(1, 2, 3) # tuple
In this example, any number of argument can be added to the function, as long as they don’t break the code inside the function.
# *args
def count_stuff(*args):
total = sum(args)
return total
# Example
result = count_stuff(1, 2, 3)
print(result)
**Kwargs in Python: Keyword Arguments
The **kwargs
parameter allows the function to accept an variable number of keyword arguments. These arguments are collected into a dictionary named kwargs
.
# **kwargs
def show_kwargs(**kwargs):
print(kwargs)
# Usage example
show_kwargs(name='jc', surname='chouinard', age=12)
{'name': 'jc', 'surname': 'chouinard', 'age': 12}
Python Function Recursion
Python allows function recursion where a function can call itself. The example below shows how the countdown() function calls itself until n is less than or equals to zero.
# Function recursion
def countdown(n):
if n <= 0:
print('End')
else:
print(n)
countdown(n - 1)
countdown(5)
Anonymous Functions
An anonymous function in Python is a function that is defined without a name. While the def keyword defines a function by its name, the lambda function defines an anonymous function.
Lambda Functions in Python
In Python, a lambda function is used to create anonymous functions. Lambda functions are defined using the lambda
keyword and are generally used for small and simple functions.
The syntax of a lambda function is as follows:
lambda arguments: expressions
# Example lambda function
double = lambda x: x * 2
result = double(5)
print(result)
10
Scope of Variables: Local VS Global Variables
The scopes of variables in Python functions determine their accessibility and visibility within the program. There are two kinds of variables:
- Local Variables: Variables defined within a function, accessible only inside the function.
- Global Variables: Variables defined outside any function, accessible throughout the program.
Local Variables
# Example of a local variable
def my_function():
local_var = 1
print(local_var)
my_function()
Global Variables
# Example of a Global variable
global_var = 10
def my_function():
print(global_var)
my_function()
print(global_var)
What “->” Means in Python Function definitions?
The -> symbol in a Python function definition represents a function annotation.
What is a Function Annotation in Python
A Python function annotation is a way to specify the expected types of function parameters and the return value. It is a way to attach metadata to functions to describe their parameters and return the values.
Below, the function takes a single parameter name
annotated with the type str
, indicating that it should be a string. The -> str
annotation after the parameter list indicates that the function will return a string.
def say_hello(name: str) -> str:
"""
Takes a name as input and returns a message.
"""
return "Hello, " + name
result = say_hello('JC')
print(result)
Hello, JC
We can print the annotations using the __annotations__
attribute on the function name.
print(say_hello.__annotations__)
{'name': <class 'str'>, 'return': <class 'str'>}
What is the Difference Between Parameters and Arguments in Python?
The difference between parameters and arguments in Python functions is that the parameter is the variable listed inside the parentheses and the argument is the value sent to the function when it is called. Both terms are often used interchangeably when we talk about Python functions.
What are the Naming Conventions for Python Functions
The Python naming conventions for functions are:
- Function name can only contain alpha-numeric characters and underscores
- Function name must start with a letter or an underscore
- Function name can’t start with a number
- Function name should not be the same as a built-in or existing module function name
- Avoid single-letter names for functions.
- Use descriptive names
def a():
# bad function name
# Not descriptive enough
def print():
# bad function name
# Don't use the names of built-in functions
pass
def 2ab():
# bad function name
# Throws SyntaxError: invalid decimal literal
def ab+():
# bad function name
# Throws SyntaxError: invalid syntax
What is the Difference Between a Function and a Method?
A Python method is similar to a Python function, but specifically belong to a Python object. While the function is called using its function name, the method is called using the .method_name() notation on the object that it is related to.
my_function() # Python Function
obj.my_method() # Python method
Conclusion
This is the end of this tutorial on Python functions. We have covered Python built-in and user-defined functions, how to create a function, and have described the various components of Python functions in detail. Next, we will look at Python methods.
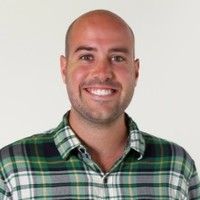
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.