In this tutorial, we will learn what pip is and how to install, update, upgrade and uninstall Python packages using pip
.
The following tutorial shows example working with the command-line prompt.
What is Pip
Pip is a package manager for Python. Pip can be used to install, update, and uninstall Python libraries (packages).
How to Install Pip
Most of the time, pip
is automatically installed when you install Python.
So. if you install Python using the python.org installer, using Anaconda, or if you have installed Python with XCode or Homebrew (on Mac), pip
will be automatically installed.
If pip is not installed on your computer, the official pip documentation v23.2.1 provides instructions on how to install pip using ensurepip
or get-pip.py
.
Install Pip with Ensurepip
One of the supported methods to install pip is ensurepip
.
To install pip with ensurepip on MacOS:
$ python -m ensurepip --upgrade
On Windows
C:> py -m ensurepip --upgrade
Install Pip with get-pip.py
The other supported method to install pip is using get-pip.py
.
To install pip with ensurepip on MacOS:
$ python get-pip.py
On Windows
C:> py get-pip.py
Pip Versions (pip, pip2 and pip3)
If you have multiple versions of Python installed in a virtual environment (Python2 and Python3), then many pip commands may be available: pip
, pip2
and pip3
.
These aliases are associated with a version of the Python executable. If pip
is associated with Python2
, it will not work with Python3
.
Check Pip Version (pip –version)
To check if pip is installed, or which version of pip is installed, use the pip --version
command in the command prompt.
$ pip --version
$ pip3 --version
Depending on whether you have multiple pip commands installed, you may choose pip
or pip3
with their arguments. For the rest of the tutorial, I will assume that you have the pip alias pointing to Python3.
$ pip 23.2.1 from /opt/homebrew/lib/python3.11/site-packages/pip (python 3.11)
Upgrade pip
$ pip install --upgrade pip
Check Installed Packages (pip show)
Use pip show
to verify the details of a Python packages installed with pip
$ pip show <package>
For example, if you show the information of the installed pandas package, you will see information about the license, the homepage of the package and the version and the location of the Python package installed.
$ pip show pandas
Name: pandas
Version: 2.1.0
Summary: Powerful data structures for data analysis, time series, and statistics
Home-page: https://pandas.pydata.org
Author:
Author-email: The Pandas Development Team <pandas-dev@python.org>
License: BSD 3-Clause License
Location: /opt/homebrew/lib/python3.11/site-packages
....
List Installed Packages (pip list)
To view all the packages installed in a Python environment, use the pip list
command.
$ pip list
Package Version
------------------------ ------------
advertools 0.13.2
pandas 2.0.1
pip 23.2.1
...
Read the following article to learn more about how to list installed packages in Python.
List Installed Packages without Pkg Management (pip freeze)
It is possible to list the installed packages without including the package management tools such as pip
and setuptools
by using the pip freeze
command.
$ pip freeze
The output below will show installed packages that are not package management tools.
advertools==0.13.2
pandas==2.0.1
You can use pip freeze to create a requirements.txt file that can be used to install a series of packages in one go.
$ pip freeze > requirements.txt
Install a Python Package (pip install)
To install a Package in Python use the pip install
command. For this to work, the package name needs to have been registered to the Python Package Index (PyPI).
$ pip install <package>
For example, we can install the python requests package with the following command.
$ pip install requests
Install Multiple Python Packages
To install multiple Python packages at once with pip
, use the pip install
command and add the packages names one after the other, separated by spaces.
$ pip install <package> <package> <package>
Here is an example where we install requests
and statsmodel
in a single line command.
$ pip install requests statsmodel
Alternatively, you can install multiple packages if you have a requirements.txt file like this:
$ pip install -r requirements.txt
Install Specific Version of Python Package with Pip
To install a specific version of a Python package using pip, run the pip install <package>==<version>
command.
$ pip install <package>==<version>
For example, to install the version 4.10.0 of BeautifulSoup, execute the following command:
$ pip install beautifulsoup4==4.10.0
Install a Min-Max Range of a Package with Pip
To install a Python package with min and max version range, use the pip install command with the package range this way:
$ pip install "package>=1.2,< 2.0"
Pip will select the best match that is at least 1.2 and less than 2.0.
Install Package From Local with Pip
If a Package was not registered with PyPI, you can download the file to your local computer and then install the package by specifying the location of the directory that contains the setup.py
file.
$ pip install path/to/setup.py
To install a zip file with pip, simply provide the location of the zipped package.
$ pip install path/to/filename.zip
Install Package From Git Repository with Pip
To install a package from a git repository, use the pip install git+<repo>
command.
$ pip install git+<repository-url>
Install Package From Github with Pip
To install a Python package from Github, use the pip install git+<url>
command along with the url of the github repository.
$ pip install git+https://github.com/<username>/<repository>
Here is an example where we install the bert package from the Google research team Github repository.
$ pip install git+https://github.com/google-research/bert.git
Install to Github Including Branch with pip
To specify the branch of the repository to be installed with pip
, add the @branch
at the end of the repository url.
$ pip install git+https://github.com/<username>/<repository>@<branch>
Here is an example where I specify which branch of the urllib3
python package that I want to install.
$ pip install git+https://github.com/urllib3/urllib3@1.26.x
Pip install in a Jupyter Notebook
there are two ways to install a package inline from a Jupyter Notebook. The first is to add the exclamation mark (!
) before the pip install
command. The exclamation is used in Jupyter to run shell commands.
!pip install requests
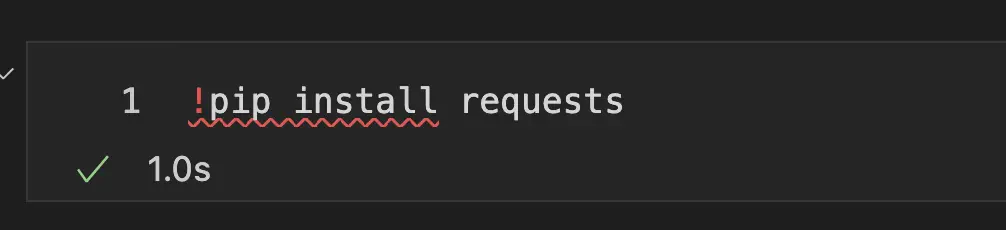
The second is to use the sys
package, and run the following command in the Jupyter kernel:
import sys
!{sys.executable} -m pip install requests
Pip install in Google Colab
To install a Python package in Google Colab, add the exclamation mark (!
) before the pip install
command just like you would do on a regular Jupyter Notebook.
!pip install advertools
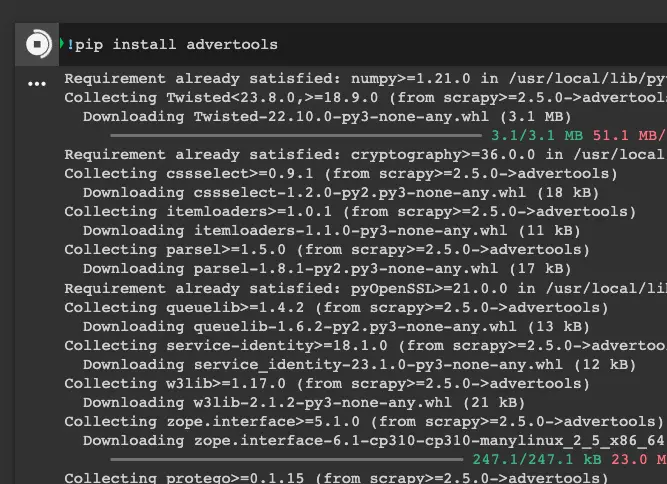
Pip install VS Python -m pip install
Use the python -m pip install
when you want to make sure to use pip
from a particular Python interpreter or virtual environment. Most of the time, however, the simpler pip install
command is enough to install Python packages.
$ pip install # default
$ python -m pip install # ensure pip is associated with python
Update a Python Package (pip install –upgrade)
To upgrade a Python package to its latest version with pip
, use pip install
with the --upgrade
flag, or its shorted -U
equivalent.
$ pip install --upgrade <package>
$ pip install -U <package>
Uninstall a Python Package (pip uninstall)
To uninstall a Python package using pip, use the pip uninstall package_name
command.
$ pip uninstall <package>
You can also uninstall multiple Python packages at once.
$ pip uninstall <package> <package> <package>
And even override the confirmation prompt by specifying the --yes
flag.
$ pip uninstall --yes <package>
$ pip uninstall -y <package>
You can even uninstall pip itself:
$ pip uninstall pip
What is Pip Install -e (–editable)
The -e
flag, short for --editable
, in pip
is used to install a project in setuptools development mode. It creates a reference or symlink to the package’s source code directory in your system’s site-packages directory.
Use this pip flag when you would installing a package locally, but need the changes to the original package to be reflected directly in your environment. This command will simply link the downloaded package to the original location.
This command will install the setuptools dependencies:
$ pip install -e <path/url>
Example use case
$ git clone <path/url>
$ cd project
$ pip install -e .
Check for Dependencies (pip check)
To verify compatible dependencies of installed packages, use pip check.
$ pip check
If there are no problems, you will get:
No broken requirements found.
If there is a missing required package, you will get:
pyramid 1.5.2 requires WebOb, which is not installed.
If the required packages exist, but the version is not the right one, you will get:
pyramid 1.5.2 has requirement WebOb>=1.3.1, but you have WebOb 0.8.
Inspect a Python Environment (pip inspect)
The pip inspect
command is used to get a detailed JSON report of the Python environment. The report includes the full description of the packages and their metadata.
$ pip inspect
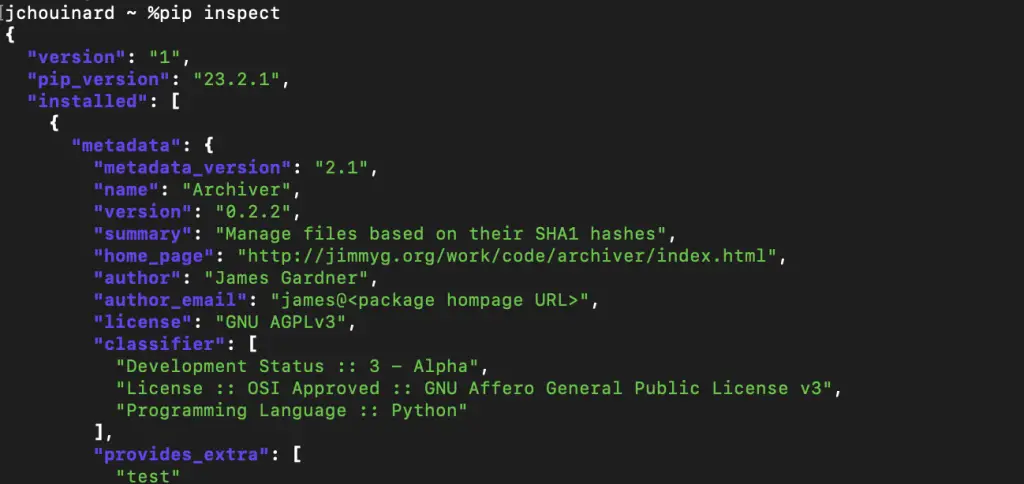
$ pip inspect | grep 'pandas'
What is Pip Download
pip download
is similar as pip install
, but instead of installing the dependencies, it collects the downloaded distributions into the directory provided. It replaces the deprecated pip install --download
command.
It will try to build the package and fallback on the source package if it can’t.
Use pip download
in a similar way as pip install
.
$ pip download -r requirements.txt
What is Pip Wheel
The pip wheel
command builds a binary distribution packages called “wheels” from Python packages.
The command will fetch the specified package and its dependencies, compile them if needed, and create a wheel package in the current directory.
$ pip wheel requests
pip install vs pip download vs pip wheel
Here is an HTML table showing you when to use pip install
, pip download
and pip wheel
.
Action | Description |
---|---|
| Install Python packages and their dependencies |
pip download | Download package distributions without installing them. Useful for acquiring packages for offline or manual installation. |
pip wheel | Create wheel archives (.whl) from source distributions or project directories. Wheels are binary distribution formats that can be quickly installed, making package distribution faster and more efficient. |
Modify the pip Alias to Point to Python3
To change the pip3
command to use the pip
alias by adding alias to your .bashrc
file.
Use the vim editor to modify the .bashrc
file on MacOS:
$ vim ~/.bashrc
Then, at the end of the file add the alias line to define the pip alias to be pip3
. This will ensure that whenever you type pip
in the command prompt, it will reference the pip3
command.
$ alias pip="pip3"
Close the file and finally source the file to refresh the settings and configurations in the Terminal:
$ source ~/.bashrc
Improve Security with Pip Hash
The pip hash
command is used to perform security verification on a package file, providing a cryptographic hash value for a file and compare it to the expected hash.
This will ensure that the file hasn’t been tampered with during download or transfer.
$ pip hash mypackage.whl
What is Pip Search
The pip search
command searches for PyPI packages whose name or summary contains some query, but is not supported by PyPI anymore.
What is Pip Cache
The pip cache
command locates the storage where pip stores downloaded packages to avoid redundant downloads when reinstalling packages.
You can configure the cache location using the --cache-dir
option.
$ pip install --cache-dir=/path/to/cache-dir SomePackage
What is Pip Config
The pip config
command is used to view and modify pip’s configuration settings. It can be used to configure options such as the default index URL.
$ pip config set global.index-url https://example.org/
What is Pip Debug
The pip debug
command is used to diagnose issues with pip’s behaviour. This command will return information about your pip setup (versions, locations, and configuration settings).
$ pip debug
What is Pip Help
The pip help
command is used to view the official documentation for a specific pip command.
$ pip help install
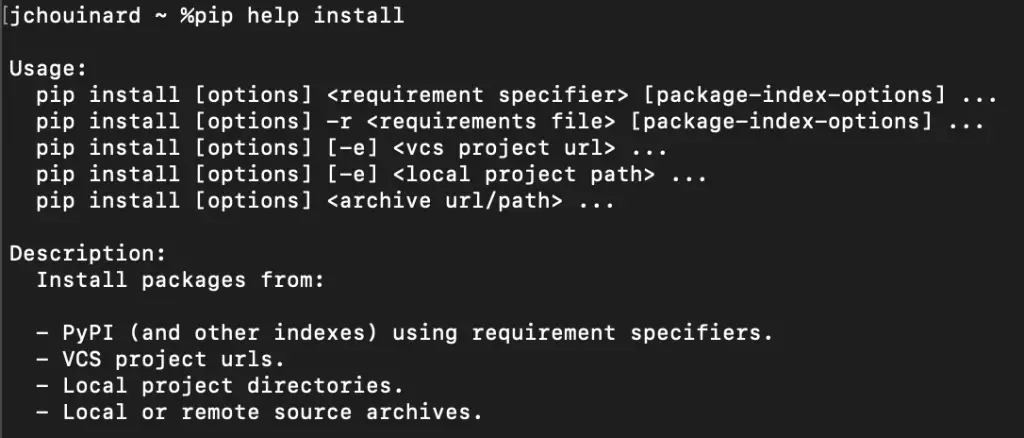
Official Pip Documentation
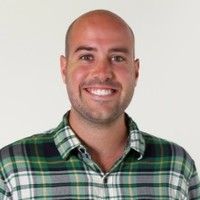
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.