In this article, we will talk about the different kinds of loops in Python, their syntax, and how to use conditional statements to improve Python iterations.
What are Python Loops?
A loop in Python is an instruction that repeats as long as a condition is met.
Python Loops Examples
# while loop
j = 0
while j < 3:
print(j)
j+=1
# For loop
for i in [0,1,2]:
print(i)
Python Loops Syntax
The syntax of a Python loop includes the keyword that creates the loop (e.g. for
, while
), the condition and the body of the loop. You will see in the syntax the colon character (:) after the condition that signifies the start of the loop block and a required indentation for each line inside the entire loop block.
# Python loop syntax
for elem in condition:
# body of the loop (indented)
while condition:
# body of the loop (indented)
Types of Loops in Python
There are two types of loops in Python: for
loops and while
loops.
The for
loop and the while
loops are control flow statements used to repeat a block of code following certain conditions.
while
loop: loop recursively as long as the condition is Truefor
loop: loop each value of a sequence
When to Use For Loops and While Loops
The for
loop should be used when you need to repeat a block of code for a fixed number of times, or when you want to loop through an iterable object (such as a list).
The while
loop should be used when you need to run a block of code or check a condition at each iteration forever.
Python While Loop
The while
loop in Python executes a block of code for as long as the condition is True
.
Similar to a if... else...
block that would be repeated over and over.
while condition:
# do something
Create a Simple While Loop
To create a simple while
loop in Python, use the while
keyword, provide a condition to define when the loop should stop and add the indented code to be executed at each iteration right after the colon character.
# Simple while loop
i = 0
while i < 5:
print(i)
i += 1
0
1
2
3
4
Infinite While Loops
By definition, while
loops will iterate until a condition is met. If the condition is always True
, then the loop will go on infinitely.
# Infinite Loop
import time
while True:
print('This is an infinite loop')
time.sleep(1)
Breaking a While Loop in Python
Because the while
loop can run infinitely, it is important to provide conditions where the loop should stop. This can be done by providing conditions, such as i < 5
, and increment i
until it reaches 5, or by using the break
control statement.
# Simple while loop
i = 0
while i < 5:
print(i)
if i == 2:
break
i += 1
0
1
2
While… Else in Python
A while
loop works similarly as an if/else statement (if condition is True, start again). Thus, use the else
keyword to define what to do when the while
loop stops iterating.
# While Else
i = 0
while i < 5:
print(i)
i += 1
else:
print('i is greater than 5')
0
1
2
3
4
i is greater than 5
Python For Loops
The for
loop in Python iterates over each element of a sequence in order and executes a block of code at each iteration.
Example: For each value in a sequence, do something.
Simple For Loop
To create a simple for
loop in Python, use the for
keyword, the element to iterate over, the iterable and add the indented code to be executed at each iteration right after the colon character.
# Simple for loop
for i in [1,2,3]:
print(i)
1
2
3
How to Loop Through a String in Python
To loop through a string in Python, use the for loop and provide the Python string as the iterable in the for statement.
# Looping other types
for letter in 'python':
print(letter)
p
y
t
h
o
n
Loop Control Statements
Control statements in Python are used in loops to control the flow of execution based on certain conditions. There are three control flow statements in Python loops: break
, continue
and pass
.
What is the Break Statement in a Python Loop?
The break
statement is used in a Python loop to stop the execution of the loop when a condition is met.
# Break
for letter in 'python':
print(letter)
if letter == 'h':
break
p
y
t
h
What is the Continue Statement in a Python Loop?
The continue
statement is used in a Python loop to skip the execution of the current iteration and move to the next iteration in the loop.
# Continue
for letter in 'python':
if letter == 'h':
continue
print(letter)
p
y
t
o
n
What is the Pass Statement in a Loop
The pass
statement is used as a null operator in Python loops and functions to tell the program to do nothing when a condition is met. The pass statement does not stop or skip iterations in loops, but simply does nothing for the current iteration.
# pass
for i in [1,2,3]:
pass
Looping Through the Range() Function
The range() function is often used in Python for
loops to provide a sequence of integers for the for
statement to iterate over. It is most often used in for
loops to iterate over integer for a defined number of time.
The Python built-in range() function returns a sequence of numbers, starting from 0 by default.
Simple Python Range() Loop
To loop and execute code through a specified number of times, use the range() function in a for
loop.
# Looping a Range
for i in range(5):
print(i)
0
1
2
3
4
Use Range() Parameters in a Python For Loop
To modify the starting, end and increment value of the range
function in a Python loop, just set each argument of the function in the specified order, and then loop through each element with a for
loop.
# Range Parameters
rg = range(
2, # Start
20, # End
2 # Increment
)
for x in rg:
print(x)
2
4
6
8
10
12
14
16
18
How to Create Nested For Loops
To create a nested for loop in Python, simply add an indentation inside a for loop block and specify the additional loop to be made. There are no limits to the number of nested loops you can make in Python.
Simple Nested For Loop in Python
To create a simple nested for
loop, start with a regular for
loop by providing a sequence to iterate over, and inside that loop, create an additional for
loop with another sequence to iterate upon.
By convention, increment the letters used as the identifier of each element of the iteration when creating a nested loop.
# Nested loop
for i in ['a','b','c']:
for j in ['d','e','f']:
print(i, j)
a d
a e
a f
b d
b e
b f
c d
c e
c f
Loop Through Nested Lists in Python
To loop through a nested list in Python, use nested for
loops.
# Loop a nested list
ls = [
[1,2,3],
[4,5,6]
]
for nst in ls:
for i in nst:
print(i)
1
2
3
4
5
6
How to Loop Through a Dictionary in Python
To loop through a dictionary in Python, use a for
loop and give the dictionary as the sequence of the loop. You can also use the .keys()
, .values()
and .items()
method on the dictionary to loop through the sequences that the dictionary methods return.
Here is an example dictionary on which we will iterate over with a for
loop.
# Create dict
my_dict = dict(
name='JC',
last_name='Chouinard',
domain='jcchouinard.com',
twitter='ChouinardJC'
)
my_dict
{'name': 'JC',
'last_name': 'Chouinard',
'domain': 'jcchouinard.com',
'twitter': 'ChouinardJC'}
How to Use Dictionary Methods in For Loops
Give the dictionary methods’ results as the sequence of the for
loop in order to iterate through the keys, the values or the key-value pairs of the Python dictionary.
my_dict.keys()
: Shows a list-likedict_keys()
object of your dictionary keysmy_dict.values()
: Shows a list-likedict_values()
object of your dictionary valuesmy_dict.items()
: Shows a list-likedict_items()
object with tuples containing dictionary (keys, values)
How to Loop Through Dictionary Keys
To loop through Python dictionary keys, use the dictionary as the sequence or use the .keys()
method to create the sequence for the for
loop to iterate over.
# Show dictionary keys
my_dict.keys()
dict_keys(['name', 'last_name', 'domain', 'twitter'])
Example of Looping Through Dictionary Keys
# Print Keys
for x in my_dict.keys():
print(x)
name
last_name
domain
twitter
How to Loop Through Dictionary Values
To loop through Python dictionary values, use the .values()
method to create the sequence for the for
loop to iterate over.
# Values method
my_dict.values()
dict_values(['JC', 'Chouinard', 'jcchouinard.com', 'ChouinardJC'])
Example of Looping Through Dictionary Values
# print values
for x in my_dict.values():
print(x)
JC
Chouinard
jcchouinard.com
ChouinardJC
Example of Accessing Dictionary Values without the .values()
method.
# Print values
for x in my_dict:
print(my_dict[x])
JC
Chouinard
jcchouinard.com
ChouinardJC
How to Loop Through Dictionary Items
To loop through a Python dictionary key-value pairs, use the .item()
method to create the sequence for the for
loop to iterate over.
# Items method
my_dict.items()
dict_items([('name', 'JC'), ('last_name', 'Chouinard'), ('domain', 'jcchouinard.com'), ('twitter', 'ChouinardJC')])
Example 1: Looping Through Dictionary Packed Key-Value Pairs
# Print Keys and values
for x in my_dict.items():
print(x[0], x[1])
name JC
last_name Chouinard
domain jcchouinard.com
twitter ChouinardJC
Example 2: Looping Through Dictionary Unpacked Key-Value Pairs
# Print Keys and values
for key, value in my_dict.items():
print(key, value)
name JC
last_name Chouinard
domain jcchouinard.com
twitter ChouinardJC
How to Loop Through Iterators in Python
There are 2 ways to loop through and iterator in Python: using the next()
function or using a looping technique such as a for
loop or a while
loop.
A Python iterator is an object that can be iterated upon.
# next()
ls = ['a','b','c']
en_object = iter(ls)
next(en_object)
(0, 'a')
# Looping an iterator
ls = ['a','b','c']
for letter in iter(ls):
print(letter)
a
b
c
How to Loop Through a Pandas DataFrame in Python
To loop through a Pandas DataFrame in Python, you can use various methods, such as iterrows()
, itertuples()
, or simply accessing the DataFrame columns and pass them as the sequence of the for
loop.
# Create DataFrame
import pandas as pd
df = pd.DataFrame({
'column_1': [1, 2, 3],
'column_2': [4, 5, 6],
'column_3': [7, 8, 9]
})
df
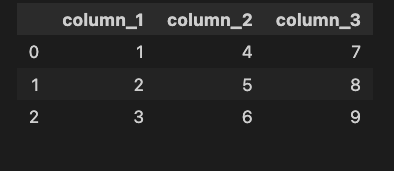
How to Loop Through Pandas DataFrame Column Names
To loop through the column names of a Pandas DataFrame in Python, you can use the DataFrame or its columns
attribute and iterate over it. Here’s an example:
# Loop each column name
for column in df:
print(column)
# Or
for column in df.columns:
print(column)
column_1
column_2
column_3
How to Loop Through Rows of a DataFrame with iterrows()
To loop through the rows of a Pandas DataFrame in Python, you can use the iterrows()
function to create the sequence to be used in a for
loop.
The example below shows an example iterator made from a Pandas DataFrame.
# Iterrows
df.iterrows()
<generator object DataFrame.iterrows at 0x29ff0a730>
# Generator object
next(df.iterrows())
(0,
column_1 1
column_2 4
column_3 7
Name: 0, dtype: int64)
Use the format for index,row in df.iterrows()
to loop through the rows of a Pandas DataFrame.
# Looping rows of a Pandas DataFrame
for index, row in df.iterrows():
print(f'Index: {index}')
print(f'Row: {row}')
print(f'Row Type: {type(row)}')
Index: 0
Row: column_1 1
column_2 4
column_3 7
Name: 0, dtype: int64
Row Type: <class 'pandas.core.series.Series'>
Index: 1
Row: column_1 2
column_2 5
column_3 8
Name: 1, dtype: int64
Row Type: <class 'pandas.core.series.Series'>
Index: 2
Row: column_1 3
column_2 6
column_3 9
Name: 2, dtype: int64
Row Type: <class 'pandas.core.series.Series'>
Access the Value of a Row and Column of a Pandas DataFrame
# Looping rows of a Pandas DataFrame
for index, row in df.iterrows():
print(f'Index: {index}')
print(f'Row for column 1: {row["column_1"]}')
Index: 0
Row for column 1: 1
Index: 1
Row for column 1: 2
Index: 2
Row for column 1: 3
How to Access the Index in a for Loop
Python has the built-in enumerate()
function that can be used to access the index in a for
loop.
# Accessing the index in a for loop
ls = ['a','b','c']
for index, elem in enumerate(ls):
print(index, elem)
0 a
1 b
2 c
How to Break Multiple Loops in Python
There are two options to break out multiple loops in Python:
- using one
break
statement per loop and complex conditional statements, - using the loop inside a function and use the
return
statement to break all loops.
Here is an example where the break
statement does not break all loops.
# Breaking out all loops
for i in range(10):
for j in ['a','b','c']:
print(i, j)
if j == 'b':
break
If it had broken all loop, only two rows would have been printed. Instead, it broke before the third value ('c'
) of the nested loop was printed.
0 a
0 b
1 a
1 b
...
9 a
9 b
To break all running loops, run the for
loop inside a Python function and use return
immediately exits the enclosing function. In the process, all of the loops will be stopped.
# Breaking out of multiple for loops
def break_out():
for i in range(10):
for j in ['a','b','c']:
print(i, j)
if j == 'b':
return
break_out()
The result is the two rows that we want to be printed in this case.
0 a
0 b
What is the Underscore in Python for Loop?
The underscore (_
) is a convention used in a Python for
loop to signify that an element will not be used by the code block inside the loop.
# understand the underscore in loops
t = [('a',1), ('b',2), ('c',3)]
ls = []
for letter, _ in t:
ls.append(letter)
ls
['a', 'b', 'c']
How to Avoid For Loops in Python
Python for
loops are very useful to iterate over a sequence, but there are upsides to minimize the usage of for
loops:
- Reducing the number of lines of code
- Making code more readable
3 Ways to Prevent Using For Loops
There are three ways to prevent using for
loops inside Python code.
- List comprehensions
- Map + Lambda
- Filter + Lambda
Looping with List Comprehensions
List comprehensions are a good alternative to replace simple Python for
loops and make code more readable. To create a list comprehension, use the square brackets ([]
) with the following format:
[element for element in sequence]
The list comprehension will return a list object.
The example below converts a for
loop using a more concise list comprehension.
# For loop
ls = []
for i in range(10):
ls.append(i)
# List Comprehension equivalent
ls2 = [i for i in range(10)]
print(ls) # for
print(ls2) # List comprehension
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Looping with Map + Lambda
The lambda function can be used inside the map()
function to map a sequence to another.
The example below converts a for
loop into a more concise map + lambda result.
# Doubling a list with a for loop
ls = []
for i in range(10):
i *= 2
ls.append(i)
# Doubling a list with Map + Lambda
ls2 = map(lambda x: x * 2, range(10))
print(ls)
print(list(ls2))
[0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
[0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
Looping with Filter + Lambda
The lambda function can be used inside the filter()
function to filter a sequence using conditionals.
The example below converts a for
loop into a more concise filter + lambda result.
# Get even numbers with for loop
even = []
for i in range(10):
if i % 2 == 0: # Even numbers
even.append(i)
# Get even numbers Filter + Lambda
even2 = filter(lambda n: n % 2 == 0, range(10))
print(even)
print(list(even2))
[0, 2, 4, 6, 8]
[0, 2, 4, 6, 8]
Conclusion
This is the end of this tutorial on Python loops.
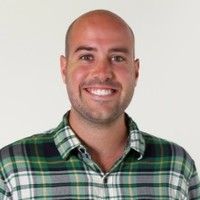
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.