When you start working with other people’s code you will run into the if __name__ == '__main__'
statement at the end of the Python code. What does it mean?
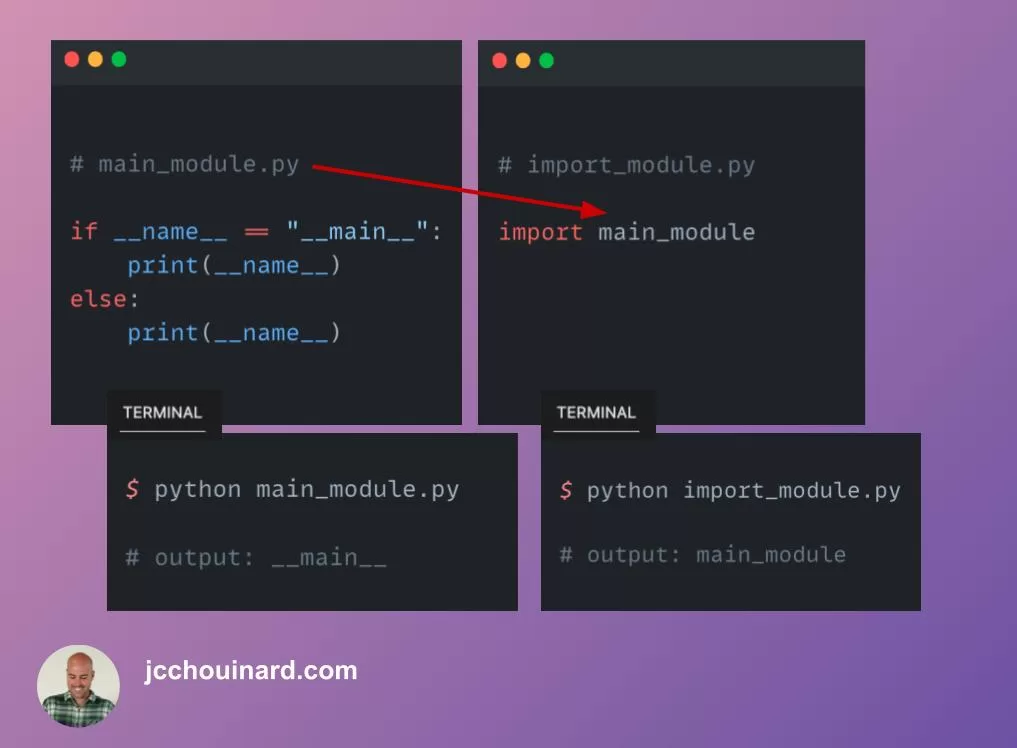
if name equals main is a construct that uses a control flow statement to allow you to execute code only when the Python script is run and not when it is imported as a module.
if __name__ == ‘__main__’ Example
What the __name__
== "__main__"
construct does is that it uses the if conditional statement to check whether you run the module (or script) directly, or if you import the module from another one.
def my_funct():
print('hello')
if __name__ == '__main__':
my_funct()
What it says is this:
If you run the module directly, execute the function, if you import the module, don’t run it.
In short, the code inside the control flow statement (if name equals main) is executed only when the script is run from the command line or through an IDE. It is not executed when it is used as a module by another Python script. With this idiom, you can ensure that your Python script behaves as intended when run as a standalone program or as a module in a larger project.
Let’s break it out.
How Does __name__ == "__main__"
Works?
The if __name__ == ‘__main__’ conditional statement is a Python programming construct that controls the execution of a script. When a Python script is run, the interpreter sets the name variable to the string ‘__main__
‘ if the script is the main program being executed. If the file is being imported as a module, then name variable is set to the name of the module instead.
By checking the value of name using the if __name__ == '__main__'
condition, you can control which code is executed in different scenarios. If the condition is True, then the indented code block following the conditional statement is executed, while if it is False, the code block is skipped. This allows you to create scripts that can be used both as standalone programs and as modules in larger projects.
Basics on how Python runs a file
Whenever the Python reads a script, it does two actions:
- it sets a few special variables like
__name__
; - it executes the code found in the script.
What __name__ Does When Running a Script
The __name__
attribute is a Python built-in variable that represents the name of the current module or script.
In simple words, when you run a Python script, Python sets the value of __name__
. If you run the script directly, the value will be set to "__main__"
.
Let’s build a main_module.py
file as an example.
The script will print the __name__
variable.
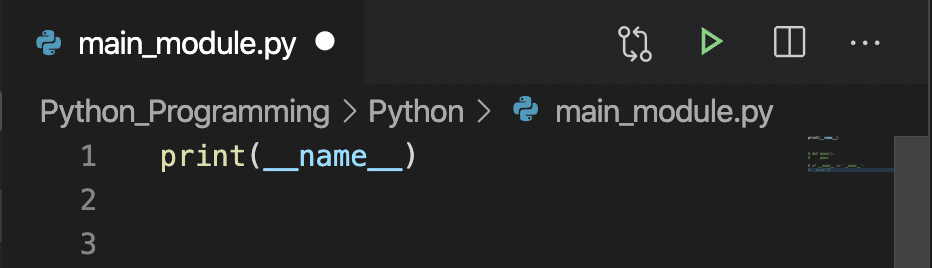
When I execute the code using python main_module.py
in the Terminal, the script will return __main__
.

What __name__ does When Importing a Script
Now, let’s see what happens when I try to import the module.
I created a import_main.py
python script that the only purpose is to import main_module.py
.
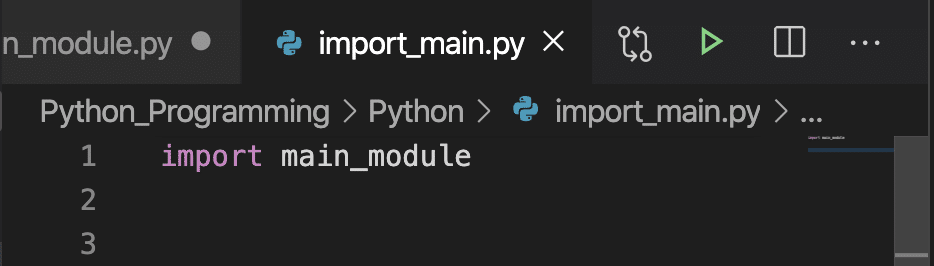
If you are not familiar with how import works, all it does is that it executes the script that you import.
When I run python import_main.py
, it runs the print(__name__)
function that we wrote in main_module.py
.
This time, however, the Terminal returns the name of the imported module instead of __main__
as it did earlier.

Wrap-up: if __name__ == ‘__main__’
Coming back to the main_module.py
, let’s make this clearer with a complete example.
def main():
print('The Main() Function Executed')
if __name__ == '__main__':
main()
else:
print('The main() function did not execute')
What the main_module.py
will now do is that:
If I execute main_module.py
, print The Main() Function Executed
, else if I import main_module.py
print The main() function did not execute
.

Run the Main() Function From Import_main
Now, If you want, you could run any function available in the main_module.py from import_main.py.
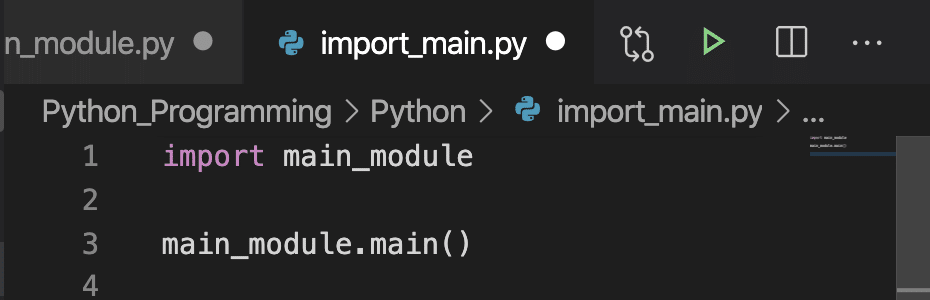

This is it, if __name__ == '__main__'
is useful to import a Module to use the functions inside of it, without running the script.
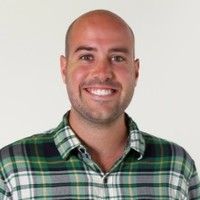
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.