In Python, the if
, elif
, else
statements are control flow statements used to apply conditions for the Python code execution.
(If condition, do something, else do something else.)
The if
, elif
, and else
keywords are used as a sequence if... elif... elif... else...
.
In this tutorial, we will learn how the if, elif and else statements work in Python.
How if/elif/else Statements Work?
The if
, elif
and else
keywords are used to apply conditions for the Python code execution.
(If condition, do something, else do something else.)
if condition:
# Do something if condition is true
else:
# Do something else if condition is false
If Statement
The if
statement is the statement that starts the control flow and defines the condition to be tested on.
The if statement can be used by itself.
# If statement
if True:
print('This is True')
This is True
Else Statement
The else
statement is the optional control flow statement that defines the code to be executed when the prior condition(s) is not met.
# If/Else Statement
i = 1
if i > 10:
print('i is greater than 10')
else:
print('i is smaller than 10')
i is smaller than 10
Elif Statement
The elif
statement is the optional control flow statement that defines additional conditions to be evaluated and the code to be executed in the conditional statement.
# Simple Elif Example
i = 12
if i < 2:
print('i is less than 2')
elif i > 10:
print('i is greater than 10')
else:
print('i is a number between 2 and 10')
i is greater than 10
Python Conditions and Logical Operators
The conditions that can be used in Python with if… elif… else statements are described in the table below.
Python Condition | Description |
---|---|
x == y | x is equal to y |
x != y | x is not equal to y |
x < y | x is less than y |
x > y | x is greater than y |
x <= y | x is less than or equal to y |
x >= y | x is greater than or equal to y |
x in sequence | x is present in the given sequence |
x not in sequence | x is not present in the given sequence |
How to Combine Multiple Conditional Statements with And… Or Keywords
The and
and or
keywords are logical operators used to combine conditional statements.
And Python Keyword
# Example and
a = 10
b = 1
c = 100
if a > b and a < c:
print('a is greater than b and smaller than c')
a is greater than b and smaller than c
Or Python Keyword
# Example OR
a = 0
b = 10
if a == 0 or a > b:
print('a is either 0 or greater than b')
a is either 0 or greater than b
How to Reverse a Python Conditional Statement with “Not”
The not
keyword is a logical operators used to reverse the conditional statements.
# Not operator
i = 10
if not i == 10:
print('i != 10')
else:
print('i equals 10')
i equals 10
The code below is equivalent to the code above that that uses the not operator.
# Equivalent to
# Not operator
i = 10
if i != 10:
print('i != 10')
else:
print('i equals 10')
i equals 10
The not operator is useful in loops to make the code somewhat more readable.
# Useful in loops
ls = [1,2,3,4]
for i in ls:
if not i == 2:
print(i)
1
3
4
Check if a Value is Present in a Sequence with “In”
The Python in
keyword can be used in two situations:
- used
in
aif
conditional to check if a value is present in a sequence - iterate through a sequence in a
for
loop
How to Use the “In” Keyword in an “If… Else” Conditional
In a if… else conditional, the in keyword is used to check if a value is present in a sequence.
# Check if a value is present
ls = [1,2,3]
i = 1
if i in ls:
print('i is present in the list')
i is present in the list
How to Create Nested If Statements
Nested if
statements are if
statements that are added inside other if
statements.
# Example Nested If Statement
s = 'hello'
if isinstance(s, str):
if 'e' in s:
print(s)
hello
How to Make Single-Line If Else Statements
A single-line if
statement in Python means that you are deleting the newline and the indentation.
The code is the same, but on one line instead of two.
# Short Hand If
i = 10
if i > 2: print("i is greater than 2")
i is greater than 2
What are Ternary Operators in Python
The if-else ternary operator in Python is a concise way to write conditional expressions in a single line of code.
Ternary Operator Syntax
The syntax of the One Line if…else Ternary Operator is:
val_true if condition else val_false
- value if true
- condition
- value if false
Below are examples of a multi-line if… else statement converted in a single-line if… else ternary operator.
Example of a Multi-line If… Else
# Multi-line if-else
i = 12
if i < 10:
print('i is less than 10')
else:
print('i is greater than 10')
Example of One-line if else with Ternary Operator
# One-line if else with ternary operator
i = 12
print('i is less than 10') if i < 10 else print('i is greater than 10')
SyntaxError With Ternary Operator
The one-line if statement must have a single line of code in the condition. The example below throws a SyntaxError.
# SyntaxError with One-line
i = 10
if i > 2: print("i is greater than 2") print('hello')
Python Pass in the If… Else Block
An if
statement can’t be empty. Use the pass
statement when creating an if
statement with no value.
The example below will throw a SyntaxError.
# SyntaxError: incomplete input
if True:
The pass statement can be used as a placeholder to the Python if... else...
block so that the code does not break.
# pass
if True:
pass
What is if __name__ == ‘__main__’?
The if name equals main statement is a control flow statement that allows you to execute code only when the Python script is run and not when it is imported as a module.
The function below will only execute if the script is executed directly, and not imported.
def my_funct():
print('hello')
if __name__ == '__main__':
my_funct()
Conclusion
We have covered how to use Python if… elif… else conditional statements.
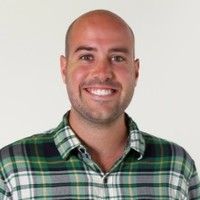
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.