This post will show you how to schedule a Python Script execution using Windows Task Scheduler. This will help you automate tasks using Python on Windows.
Use crontab for python script automation on Mac.
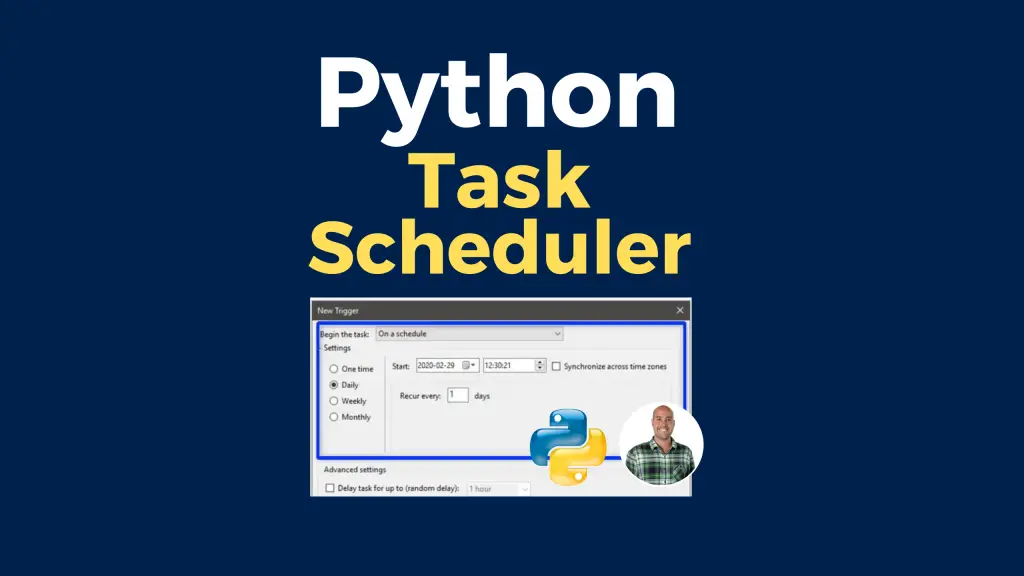
Before we can cover all that, we need to learn how to use Windows Task Scheduler.
What is Windows Task Scheduler?
Windows Task Scheduler is a component that gives the ability to schedule and automate tasks in Windows by running scripts or programs automatically at a given moment.
Get Started Using Windows Task Scheduler
To run your python scheduler you will need to create a task, create an action, add the path to your python executable file and to your python script and add a trigger to schedule your script.
1. Create Your First Task
Search for “Task Scheduler”.
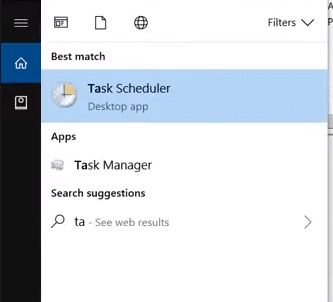
This will open the Windows Task Scheduler GUI.
Go to Actions > Create Task…
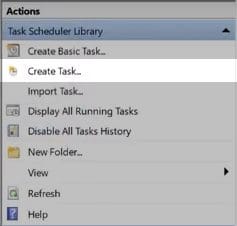
Give a name
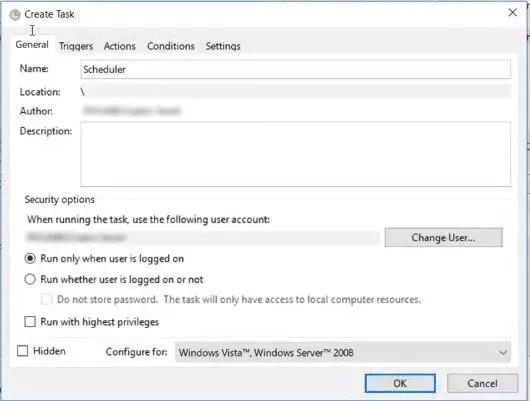
2. Create an Action
Go to Actions > New
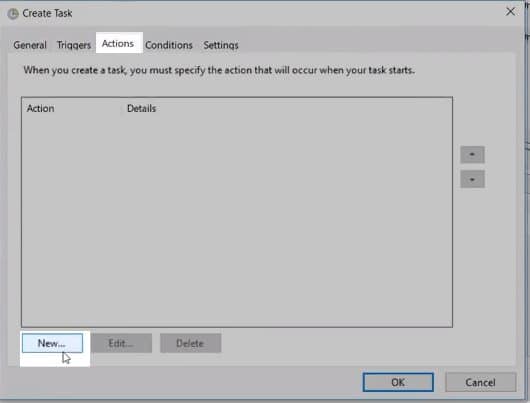
3. Add the Python Executable File to the Program Script
Find the Python Path using where python
in the command line.
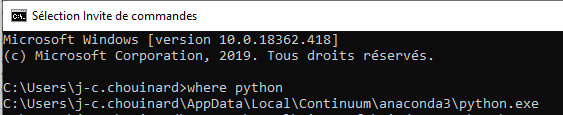
From the command prompt copy the script to use in the action.
C:\yourpath\python.exe
or in my case
C:\Users\j-c.chouinard\AppData\Local\Continuum\anaconda3\python.exe
In Program/Script, add the path that you have copied from the command line.
4. Add the Path to Your Python Script in the Arguments
Go to the folder where your Python script is located. Right-click on the file and select Copy as path
.
If you have a file located at this location.
C:\user\your_python_project_path\yourFile.py
In the "Add arguments (optional)
” box, you will add the name of your python file.
yourFile.py
In the "Start in (optional)"
box, you will add the location of your python file.
C:\user\your_python_project_path
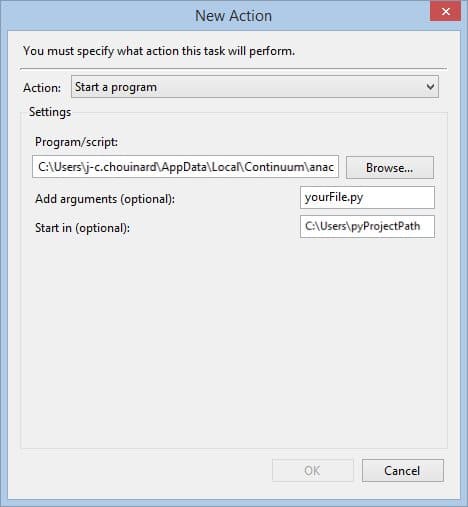
Click “OK”.
Note: Alternatively, you could create a batch file combining your Python script and Python executable file in a
.bat
file.
5. Trigger Your Script Execution
Go to “Triggers” > New
Choose the repetition that you want. Here you can schedule python scripts to run daily, weekly, monthly or just one time.
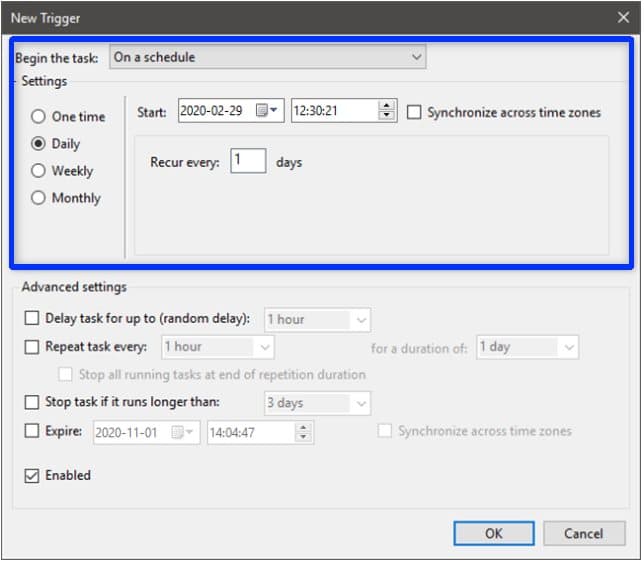
Click “OK”
Once, you have set this up, your trigger is now active and your python script will run automatically every day.
Whether you decide to repeat the task every week or every hour, you may use the task scheduler wizard.
This is the best way to schedule a function to run at a specific time of the day without using CRON job. However, there are alternatives to run it from within your code using Advanced Python Scheduler, but I don’t like that option as it requires the code to keep running.
Example Python Script
from datetime import datetime
import os
def write_file(filename, data):
if os.path.isfile(filename):
with open(filename, 'a') as f:
f.write('\n' + data)
else:
with open(filename, 'w') as f:
f.write(data)
def print_time():
now = datetime.now()
current_time = now.strftime("%H:%M:%S")
data = "Current Time = " + current_time
return data
write_file('test.txt' , print_time())
Task Scheduler Python Script Automation FAQs
Can Task Scheduler run a Python script?
Yes, you can execute a Python script with Windows Task Scheduler. If your script works using the command prompt, you can schedule your script to run at a specific time and date.
How to schedule a Python script with Task Scheduler?
To schedule a Python script with Task scheduler, create an action and add the path to your Python executable file, add the path to the script in the “Start in” box and add the name of the Python file ase an argument. Then, create a trigger to schedule the execution of your script.
Does Task Scheduler work when computer is sleeping?
It does not work by default, but you could enable it in the conditions tab. To run your script while your computer is sleeping, just select the option: “Wake the computer to run this task”.
About Windows Task Scheduler
Task scheduler triggers | Time-based or event-based triggers to start a task |
Task scheduler actions | Actions to be performed by the task |
Task scheduler conditions | Rules that define if a task can run after being triggered |
Task Scheduler settings | Settings that define how a task is run, is deleted, is stopped |
Conclusion
That’s it, you now know how to run a Python script automatically using Windows Task Scheduler.
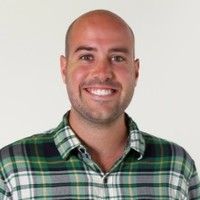
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.