In Python programming, a variable is a container that stores data values.
Creating a Variable in Python
# Assign variables
x = 10
# print
print(x)
Output
10
Variables Can Have Multiple Data Types
# Types
x = 10
y = "hello"
# print
print(type(x))
print(type(y))
Output
<class 'int'>
<class 'str'>
Reassign a Variable in Python
## Reassign variables
x = 1
print(x, type(x))
x = "hello"
print(x, type(x))
Output
1 <class 'int'>
hello <class 'str'>
Casting Variables in Python
You can modify the variable data type in Python.
Casting an Integer to a String
For example, casting can be used to convert an integer into a string.
# Casting variables
x = 3 # integer
print(type(x))
x = str(x)
print(type(x))
Output
<class 'int'>
<class 'str'>
Casting a String to Integer
# Int
int('3')
Output
3
Casting a Integer to Float
# float
float(3)
Output
3.0
How to Name Python Variables?
Choosing a good name for your variables makes your code more understandable and easy to read.
Python has standard variable naming conventions. Some are required, and others are optional.
Required rules for variable names
- Variable name can only contain alpha-numeric characters and underscores
- Variable name must start with a letter or an underscore
- Variable name can’t start with a number
2ab = 'bad variable name'
ab# = 'bad variable name'
var_1 = 'good variable name'
Best Practices in Naming Variables
A good variable name is:
- Follows Python best practices
- Short
- Descriptive
- Case sensitive
- Consistent across your code
- Never uses lowercase “l” or uppercase “O” as single letter variable
Python Variables Naming Conventions
Python naming standards tell us that we should prefer short all-lowercase names, preferably without underscores. For longer names, use underscores to write variable names in snake case. Example naming conventions, for:
- Variables, functions, methods and modules: Snake case (snake_case)
- Classes: Pascal Case (PascalCase) also known as CapWords
- Constants: Capitalized Snake Case (CAPITALIZED_SNAKE_CASE’)
# Standards
# variables, functions and modules
snake_case = 'my variable'
# classes
class MyFirstClass:
x = 'PascalCase'
# Constants
MY_CONSTANT = 'CAPITALIZED_SNAKE_CASE'
# other possible types of casing
camelCase = 'camel case is often used in JavaScript'
Using Short Variables
Short variable names with single descriptive words are preferred over longer variable names with multiple words separated by underscores.
# Example
"df" is better than "my_dataframe"
Using Descriptive Variables
Descriptive variable names are preferred over vague names.
# Example
"df" is better than "x"
Using Case Sensitive Variables
Differently cased variables are different variables.
# Case sensitive
var = 1
VAR = 2
var == VAR
False
Being Consistent
Being consistent across your code is important.
It is better to use slightly-wrong variable names and be consistent than break consistency to start following a standard.
# Not perfect
myLongVar = 'variable 1'
myVeryLongVar = 'variable 2'
myVeryVeryLongVar = 'variable 3'
# Worse
myLongVar = 'variable 1'
myVeryLongVar = 'variable 2'
my_var = 'variable 3'
Avoiding Confusing Letters
Never use lowercase “l” or uppercase “O” as single letter variable.
They may easily be confused with the number 0 and the number 1.
# Confusing variable names
l = 'Easy to confuse with 1'
O = 'Easy to confuse with 0'
Assign Multiple Variables
Python allows to assign multiple variables in a single line.
Assign many values to multiple variables
# Assign many values to multiple variables
x, y = 1, 2
print(x, y)
1 2
Assign 1 value to multiple variables
# One to multiple
x = y = 1
print(x, y)
1 1
Unpack Arrays to multiple variables
# Unpack
numbers = [1, 2, 3]
x, y, z = numbers
print(x, y, z)
1 2 3
What are Global Variables
Global variables are variables created outside of a function.
Created outside and used inside
# Created outside and used inside
x = 'hello'
def say_something():
print(x)
say_something()
hello
Created inside and used outside
# Created inside and outside
x = 'hello'
def say_something():
x = 'I am inside'
print(x)
print('x when not running the function:', x)
# Running the function
say_something()
x when not running the function: hello
I am inside
Conclusion
In this Python for Beginner tutorial, we have learn what variables are, how to assign values to variables, how to cast variable data types, how to name variables, and how to use global variables.
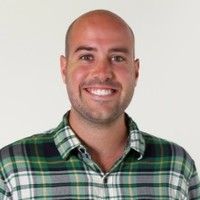
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.