In Python, Tuples are an immutable data structure of the sequence type that store a collection of data. Just like with a list, Tuples stores Python objects, but it is immutable (can’t be changed), which makes it much faster than a list.
Tuples are represented by objects separated by commas inside parentheses
t = (1, 'hello', True)
Examples of Python Tuples
Python tuples examples range from tuples containing zero to multiple values from one or multiple data types.
Here are a few examples of tuples in Python:
()
– Empty Tuple(1,)
– Tuple with a single value(1,2,3)
– Tuple containing numeric objects('hello', 'world')
– Tuple containing string objects(True, [1,2], (3,4), 'hello')
– Tuple containing multiple objects with different data types
Characteristics of a Python Tuple
Python Tuples have these 5 characteristics.
Here are the characteristics of Python Tuples:
- ordered
- unchangeable
- immutable
- allow duplicate value
- allow values with multiple data types
What Does it Mean When we Say a Tuple is Immutable?
Immutable in Python means that once we have declared the content of the Tuple it cannot be changed. Mutable means that they can be changed.
Tuples are immutable and lists are mutable.
# list
ls = [1,2,3]
ls[1] = 3
ls
# [1, 3, 3]
# tuple
t = (1,2,3)
t[1] = 3
# TypeError: 'tuple' object does not support item assignment
That being said, it is possible to change the values inside a Tuple:
t = (1, [1,2])
t[1].append(3)
t
# (1, [1, 2, 3])
What is important is that these two elements don’t change:
- The Objects IDs
- The order of the IDs
And in this case, the ID and the order of the IDs stayed the same.
t = (1, [1,2])
print(id(t[0]), id(t[1]))
t[1].append(3)
print(id(t[0]), id(t[1]))
4319241024 11583874304
4319241024 11583874304
What are Sequence Types in Python
Sequences types in Python are data types that follow a certain set of characteristics that are unique to sequences. There are three basic sequence types: lists, tuples, and range.
Create a Tuple in Python
There are three ways to create a tuple in Python:
- Using Parentheses
- Using tuple() constructor
- Using Comma-separated values
To create a Python tuple, use the parentheses () with each value separated by a comma.
# Example tuple
t = (1, 2, 3)
t
(1, 2, 3)
A tuple can also be created using the tuple() constructor.
# Tuple constructor
tuple([1,2,3])
(1, 2, 3)
By default, comma-separated values will also return a tuple.
t = 1,2,3
t
(1, 2, 3)
Create a Tuples with Constructor VS Parentheses VS Commas
When creating a tuple it is better to initialize with the parentheses than simple comma-separated values since it makes the code more understandable. Parentheses are more efficient than tuple constructors while constructors are better when creating tuples from iterables.
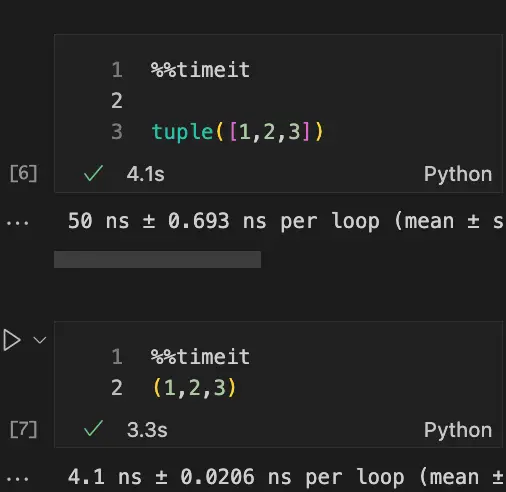
Show if the Object is a Tuple
To show if a Python object is of the tuple
data type, use the type() function on the object.
# Showing the type of a Tuple
t = (1, 2, 3)
type(t)
tuple
Indexing a Tuple
Here is an example of indexing a tuple in Python. To access an item in a Tuple use the index operator [] starting from 0.
letters = ('a','b','c','d','e')
print(letters[0])
'a'
Slicing a Tuple
To access multiple objects in a Tuple use the index operator [] and the slicing operator :
.
letters = ('a','b','c','d','e')
print(letters[:3])
print(letters[1:4])
('a', 'b', 'c')
('b', 'c', 'd')
Python Tuples are Ordered
Python tuples are ordered. Being ordered does not mean that the order is directional (e.g. 1,2,3,…), but simply that the order of the element can’t be changed.
# Simply says that the order will not change
t = (3, 1, 2)
type(t)
tuple
Python Tuples are Immutable
Python tuples are immutable, meaning they can’t be changed.
Can’t Reassign a Value on Tuple
Python tuples are unchangeable. Thus, values of the tuples can’t be reassigned.
# While you can reassign a value on lists
ls = [1, 2, 3]
ls[0] = 2
ls
[2, 2, 3]
# Can't reassign a value on a Tuple
t = (1, 2, 3)
t[0] = 2
TypeError: 'tuple' object does not support item assignment
Tuples Can’t be Sorted
Python tuples don’t have the sort method like lists do.
# While you can sort lists
ls = [3, 1, 2]
ls.sort()
ls
[1, 2, 3]
# You can't sort Tuples
t = (3, 1, 2)
t.sort()
AttributeError: 'tuple' object has no attribute 'sort'
You can use the sorted() function on the Tuple, but it will not be returned as a tuple, but as a sorted list.
t = (3, 1, 2)
sorted(t)
[1, 2, 3]
Python Tuples can Have Multiple Data Types
Python tuples can contain various data types.
# Multiple data types
t = (
1,
'hello',
['a', 'b'],
dict(a=1,b=2),
False,
(1, 2, 3),
)
print(type(t))
t
<class 'tuple'>
(1, 'hello', ['a', 'b'], {'a': 1, 'b': 2}, False, (1, 2, 3))
How to Create a Tuple with a Single Item
To create a tuple with a single item, open the parentheses, add your item, a comma and close the parenteses (item,)
.
# Tuple
t = ('hello',)
type(t)
tuple
If you don’t add the comma, you will not create a tuple.
# Not a Tuple
nt = ('hello')
type(nt)
str
Specify a Tuple with a Tuple Constructor
To specify a tuple, use the tuple()
constructor function.
# Tuple from list
t = tuple(['hello','world'])
print(type(t))
t
<class 'tuple'>
('hello', 'world')
Specify a Tuple from a String
You can specify a tuple from a string by passing a string to the tuple()
constructor function.
# Tuple from string
t = tuple('hello')
t
('h', 'e', 'l', 'l', 'o')
Specify a Tuple from a Dictionary
You can specify a tuple from a dictionary by passing a dictionary object to the tuple()
constructor function.
# Tuple from dictionary
d = dict(a=1, b=2, c=3)
t = tuple(d)
t
('a', 'b', 'c')
Create an Empty Python Tuple
You can create an empty tuple by passing no argument to the tuple()
constructor function.
# Empty Tuple
t = tuple()
t
()
This is rarely useful, but still can be when you want to always return a tuple even if it were to be empty.
# Almost not useful
def positive_tuples_less_than(x):
if x <= 0:
return ()
else:
return tuple(range(x))
positive_tuples_less_than(5)
(0, 1, 2, 3, 4)
Iterating Through a Tuple
To iterate through a Python tuple, use a for loop.
letters = ('a','b','c','d','e')
for letter in letters:
print(letter)
a
b
c
d
e
Check if Item Exist in a Tuple
To check if a specific object exists in a Tuple, use the in
operator.
letters = ('a','b','c','d','e')
print('d' in letters)
True
How to Create a Tuple from a Range of Values
It is possible to create a tuple from a range of integers by using the tuple()
constructor.
tuple(range(1, 50))
How to Increment Elements of a Tuple
To Increment elements inside of a tuple, use a list comprehension and rebuild the tuple using the tuple()
constructor.
tup = (1,2,3)
tuple([x+1 for x in tup])
(2,3,4)
Python Tuples Unpacking
Tuples unpacking is the process of re-assigning tuples values back to Python variables.
# Python tuples unpacking
numbers = (1,2,3)
(a,b,c) = numbers
print(a)
print(b)
print(c)
1
2
3
Tuples Unpacking with the Asterisk (*
)
The asterisk next to a tuple variable name is used in for unpacking Python tuples when number of variables is less than the number of values.
# Tuple unpacking using the asterisk
numbers = (1,2,3,4,5)
(one, two, *more_than_three) = numbers
print(one)
print(two)
print(more_than_three)
1
2
[3, 4, 5]
Unpacking Start or Middle Values with the Asterisk
The asterisk can also be used to unpack multiple values in a single variable on variables other than the last.
# Tuple unpacking using the asterisk
numbers = (1,2,3,4,5)
(one, *two_to_last, last) = numbers
print(one)
print(two_to_last)
print(last)
1
[2, 3, 4]
5
How to Zip Tuples Together
Use the zip()
function to zip multiple tuples together.
numbers = (1,2,3,4)
letters = ('a','b','c','d')
z_object = zip(numbers, letters)
tuple(z_object)
((1, 'a'), (2, 'b'), (3, 'c'), (4, 'd'))
Python Tuple Methods
There are two methods that can be used on tuples: count() and index().
Count()
# Counting Values
t = (1, 2, 3, 3, 3, 4, 3, 4, 5)
t.count(3)
4
Index()
# Getting the index of an element
t = ('a','b','c','d')
t.index('c')
2
# Scanning the tuple from start_index to end_index
t = ('a','b','c','a','c','d')
t.index('c', 3, 5)
4
Functions that can be Used on a Tuple
There are 9 built-in Python functions that can be used on a tuple.
- all() – Return true if all elements of tuples are true or tuple is empty
- any() – Return true if any elements of tuples are true and False when tuple is empty
- enumerate() – Return an enumerate object from the tuple
- len() – Return the length of the tuple
- max() – Return the maximum value from the tuple
- min() – Return the minimum value from the tuple
- sum() – Return the sum of all values of the tuple
- sorted() – Return a sorted list of the values of the tuple
- tuple() – Converts a sequence to a tuple
Difference Between Tuples and Lists in Python
The two main differences between tuples and lists in Python is that tuples are immutable and lists are mutable, and tuples use parentheses instead of square brackets. They are both a sequence of objects separated by a colon.
Why use Tuples Instead of Lists in Python
Tuples are more memory efficient than lists in Python. Since tuples can’t be modified and lists can, lists need to allocate more memory than tuples in case it has to be changed after it has been created.
Why do Tuples Take Less Space in Memory Than Lists?
Tuples take less space in memory than lists because lists are variable-sized, they over-allocate to enable to append to full lists and they need a layer of indirection to store pointers to the elements instead of directly storing elements in a fixed-sized tuple structure.
The example below shows what we mean by tuples taking less memory than lists.
ls = [1,2,3]
print(ls.__sizeof__()) # 72
tup = (1,2,3)
print(tup.__sizeof__()) # 48
When to Use Tuples, When to Use Lists in Python
You should use tuples when you don’t need your object to be mutable. You should use lists when you need your object to be mutable (e.g. changeable). Tuples will be more efficient in terms of storage and computing, but will not allow you to change them after their creation.
Advanced Tuples Concepts
How does Tuple Comparison Work in Python?
Tuples in Python are compared position by position.
Take this Tuple comparison for example.
(1,2) < (2,1)
True
The reason why the above is True is because the first item of the first tuple is compared to the first item of the second tuple. If the condition is met on the first item, then the result is returned, otherwise it moves to the other.
(1,2,3) < (1,2,4)
True
Can Tuples Contain Mutable Items?
Yes, tuples can contain mutable items inside them. For example, the tuple (t) below contains a list (a). When we change an item of the list a, the tuple seem to change too.
Does it mean that the tuple is mutable? No
a = [1,2,3]
t = (a, )
print(t)
a[1] = 5
print(t)
The reason for this is that the Python container for the tuple only contains references to the item, when the list inside the tuple changed, the references did not change, and the tuple was did not get notified.
The tuple contains a reference to the list. When you call the tuple, the list gets referred, but the list does not “know” if it is a tuple, a dictionary or any other object that refers to it.
Tuples Swapping
Because Tuples contains references to elements, elements inside them can be swapped, as long as they are external variables.
x = 'a'
y = 1
print('x:', x, 'y:', y)
# Swapping
(x, y) = (y, x)
print('x:', x, 'y:', y)
x: a y: 1
x: 1 y: a
Can you Add to a Tuple?
Since tuples are immutable, by definition, you can’t add elements to it, but why does using the +=
sign on a tuple allows us to end up with a tuple with combined values?
a = (1,2)
a += (3,4)
a
# (1, 2, 3, 4)
What happens here is that we reassign a new tuple to where the variable a
points to. By looking at the variable id()
, we can see that variable points to a different location before and after the operation. All we do here is use variable a
, to create a new extended variable a
: a = a + (3, 4)
.
a = (1, 2)
a1_id = id(a)
print(a1_id) # 5791700736
a += (3, 4)
a2_id = id(a)
print(a2_id) # 5791370592
a1_id == a2_id # False
How to Change the Position of Elements in a Tuple?
It is not possible to change the position of elements inside a tuple since tuples are immutable. To rearrange a tuple in Python, the only option is to create a new tuple.
a_tuple = (1, 2)
print(a_tuple, id(a_tuple))
a_tuple = a_tuple[1], a_tuple[0]
print(a_tuple, id(a_tuple))
Below, you will see that the tuple looks like it has been rearranged, but that the id where the tuple variable points to is different.
(1, 2) 5791708544
(2, 1) 5790218496
How Many Elements can a Tuple Store?
A tuple is a finite ordered list that can store any number of elements. Any number of non-negative integers is valid. We can name tuples according to their number of elements (n-tuples).
What are N-Tuples
The n-tuple in Python, or in any mathematical language, represents the number of elements stored in the data structure. The prefix represents the number of items in the tuple, each with its on name.
Name for Tuples of Specific Lengths
- 0-tuple: null tuple, empty tuple, empty sequence, unit, zero n-tuple, empty function
- 1-tuple: single tuple, monad, singleton
- 2-tuple: ordered pair, couple, twin
- 3-tuple: triple, triplet or triad
- 4-tuple: quadruple, tetrad, quartet, quadruplet
- 5-tuple: quintuple, quintuplet
- 6-tuple: sextuple, hexatuple, sextuplet
- 7-tuple: septuple, septuplet
- 8-tuple: octuple, octuplet
- 9-tuple: nontuple
- 10-tuple: decuple
- 20-tuple: vigintuple
- 30-tuple: tringintuple
- 100-tuple: centuple
Conclusion
In a nutshell, Python tuples are an immutable data type that can be used to store a collection of values. They are used for data integrity and performance. Tuples also offer indexing and slicing operations, making them very useful in Python programming.
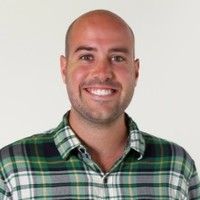
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.