Sets in Python are one of the 4 data types used to store collections of data: dictionaries, lists, sets, tuples.
Sets can be defined using the curly brackets {}
:
this_set = {1, 2, 3}
What is a Set in Python?
A set in Python is a data type used to store an unordered collection of unique elements. Python sets must be:
- Unordered
- Unchangeable (Immutable)
- Have no duplicate values
Why Use Sets in Python
Use Python sets when you want to create Python objects that should not be changed later in the code and when you want to make sure there are no duplicate values within the object.
Sets are often used for:
- Web Crawlers where URLs should not be added twice to the queue
- Form submissions where you want to discard duplicate submissions
- ChatBots where you may want to give different answers if the user keeps submitting the same input.
How to Create a Set in Python
To create a set in Python, use the curly brackets {}
or use the set()
constructor.
# create a set with curly brackets
s = {1,2,3}
s
{1, 2, 3}
List to Set in Python
You can also create a set using the set()
function with an iterator as its argument.
# create a set with set() constructor
s = set([1,2,3])
s
{1, 2, 3}
Characteristics of Python Sets
Python Sets have a multiple characteristics.
- Duplicates are not allowed
- They can have Multiple data Types
- Sets Can’t Be Accessed with the Index
- Not Subscriptable
Duplicates are not Allowed in Sets
Sets don’t allow duplicated values. This makes them useful to create objects where no duplicate values are found.
# Using the set() constructor
# allows to deduplicate a list
ls = [1, 2, 3, 4, 3, 3, 4, 5, 5, 5]
set(ls)
{1, 2, 3, 4, 5}
Sets can Have Multiple Data Types
A set can be composed of multiple data types such as a str
, a int
, a bool
, etc.
# Creating a set
s = {'hello',1,True}
s
{1, 'hello'}
Sets are Not Subscriptable (Can’t Be Accessed with the Index)
Sets in Python are not subscriptable. This means that you can’t access each element of the set with its index.
If you do, Python will return a TypeError
.
# not subscriptable
s = set((1,2,3))
s[0]
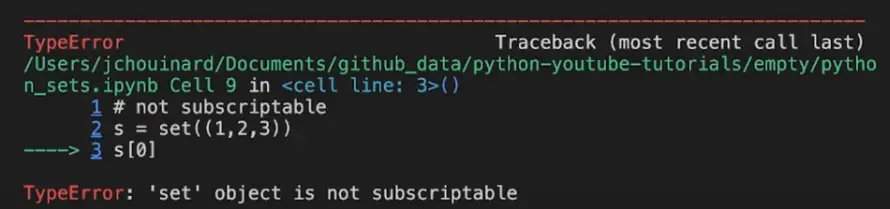
Prevent the TypeError: 'set' object is not subscriptable
in one of two ways: use a for
loop to access each element of the set, convert to a list.
# Need a loop
s = set((1,2,3))
for i in s:
print(i)
1
2
3
Avoid the TypeError
by converting the set to a list.
# Or a constructor
list({1,2,3})[0]
1
Check the Length of a Python Set: len()
Use the len() function to check the length of a Python set.
# Length function
s = {1, 2, 3}
len(s)
3
Should You Use Set Methods or Set Operators?
In Python, many operations on sets can be done by using operators or by using methods. For example, the s1.difference(s2)
method is equivalent to using the minus operator of s1 - s2
.
# method vs operator
s1 = {1, 2, 3, 6}
s2 = {1, 2, 3, 4, 5}
print(s1.difference(s2))
print(s1 - s2)
{6}
{6}
To choose a set method instead a set operator depends on the context, readability and performance considerations of your project.
Here are some of the reasons why you would choose either set methods or set operators:
- Set methods: Readable, Flexible and allow Method Chaining
- Set Operators: Compact, Understandable Across languages, Performant
Check if a Value Exists in a Set: (not) in
Use the in operator on the set object to check if a value exists inside of it.
# In
s = {1, 2, 3}
2 in s
True
# Not In
s = {1, 2, 3}
5 not in s
True
How to Add Items to a Set: add()
To add Items to a Python set, use the add()
method.
# Add method
s = {'learn','python'}
s.add('fun')
s
{'fun', 'learn', 'python'}
Updating a Set: update()
Add a Set to Another Set
To add a set to another Python set, use the update()
method.
# Adding a set with update
s1 = {1,2}
s2 ={2,3,4}
s1.update(s2)
s1
{1, 2, 3, 4}
The operator equivalent of the update()
method is the |=
operator.
# Adding a set with |=
s1 = {1,2}
s2 ={2,3,4}
s1 |= s2
s1
{1, 2, 3, 4}
Add Any Iterable to a Set
You can add any iterable that you want to a set using the update() method.
# Any iterable
s1 = {1,2}
s2 = [2,3,4]
s1.update(s2)
s1
{1, 2, 3, 4}
Why the + Operator Doesn Work With Sets
The + operator is not understood by sets because, in order to differentiate intersections and unions. Thus, Python uses the | for set union and & for set intersection. See the stackoverflow thread.
Keep The Intersections Between Two Sets: intersection_update()
To update a set, keeping the intersection it has with another one, use the intersection_update()
method.
# Adding a set with intersection_update
s1 = {1,2,3}
s2 = {2,3,4}
s1.intersection_update(s2)
s1
{2, 3}
The operator equivalent of the intersection_update()
method is the &=
operator.
# Adding a set with &=
s1 = {1,2,3}
s2 = {2,3,4}
s1 &= s2
s1
{2, 3}
Find Differences From another Set: difference_update()
To update a set, keeping the differences it has with another one, use the difference_update()
method.
# Adding a set with difference_update
s1 = {1,2,3}
s2 = {2,3,4}
s1.difference_update(s2)
s1
{1}
The equivalent operator to the
method is the difference_update
()-=
operator.
# Adding a set with -=
s1 = {1,2}
s2 = {2,3,4}
s1 -= s2
s1
{1}
Keep All Differences Between Two Sets: difference_update()
To update a set, keeping all the differences between the two sets, use the symmetric_difference_update()
method.
# symmetric_difference_update
s1 = {1,2,3}
s2 = {2,3,4}
s1.symmetric_difference_update(s2)
s1
{1, 4}
The equivalent operator to the
method is the
()symmetric_difference_update
^=
operator.
# Symmetric difference with the ^= operator
s1 = {1,2,3}
s2 = {2,3,4}
s1 ^= s2
s1
{1, 4}
Remove a First Item from a Set: pop()
To remove the first item from a set in Python, use the pop()
method.
# Remove first item
s = {1, 2, 3, 4}
s.pop()
s
{2, 3, 4}
Remove Elements from a Set: remove()
To remove an element from a set in Python, use the remove()
method with the element to be removed as an argument.
# Remove method
s = {1, 2, 3, 4}
s.remove(1)
s
{2, 3, 4}
How to Fix the KeyError with Set Remove(): discard()
To fix the KeyError returned when using the remove() method with a value that does not exist in a set, either change the value or use the discard() method.
# KeyError with Remove() method
s = {1, 2, 3, 4}
s.remove(5) # Throws an error
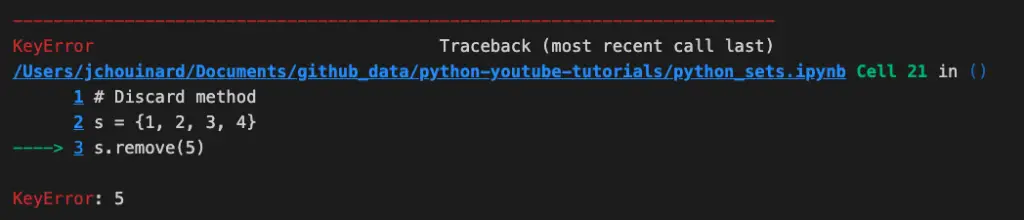
# Fix KeyError with discard() method
s = {1, 2, 3, 4}
s.discard(5)
s
{1, 2, 3, 4}
How to Join Python Sets: Union()
To join Python sets together, use the union() method on the first set and assign the second set as the argument of the method.
# When update changes the set
# Union needs to be assigned
s1 = {1,2,3}
s2 = {4,5,6}
s3 = s1.union(s2)
s3
{1, 2, 3, 4, 5, 6}
Check if Values of a Set are in Another Set: issubset()
To check if values of a set are all contained within another set, use the issubset()
method on the set object. Equivalent to s <= t.
# issubset
s = {1, 2, 3}
t = {1, 2, 3, 4, 5}
s.issubset(t)
True
# issubset
s = {1, 2, 3}
t = {1, 2, 4, 5}
s.issubset(t)
False
Check if a Set Contains Another Set: issuperset()
To check if a set contains all the values of another set, use the issuperset()
method on the set object. Equivalent to s >= t.
# issuperset
s = {1, 2, 3}
t = {1, 2, 3, 4, 5}
t.issuperset(s)
True
Get Similar Elements From Two Sets: intersection()
To create a new set from similar elements that are contained in two different sets, use the intersection()
method on the first set and provide the second set as an argument of the method.
# intersection
s = {1, 2, 3, 6}
t = {1, 2, 3, 4, 5}
t.intersection(s)
{1, 2, 3}
Get Differences Between Sets
To show values that are different between sets, use the difference() method or the symmetric_difference() method.
Difference(): Show additional values in a Set
To show values not contained in an another set, use the difference() method on the set for which you want to expose the additional values not contained in another one.
# difference
s1 = {1, 2, 3, 6}
s2 = {1, 2, 3, 4, 5}
# Values in s1 but not in s2
print(s1.difference(s2))
# Values in s2 but not in s1
print(s2.difference(s1))
{6}
{4, 5}
Symmetric_difference(): Show values that are not in both sets
To show values that are either in one set or the other, but not in both, use the symmetric_difference()
method. This time, the order of the object or the argument does not matter.
# symmetric_difference
s1 = {1, 2, 3, 6}
s2 = {1, 2, 3, 4, 5}
s1.symmetric_difference(s2)
{4, 5, 6}
Check if Sets Have Intersection: isdisjoint()
Use the isdisjoint() method to check if two sets have an intersection or not.
# isdisjoint()
s1 = {1,2,3}
s2 = {3,4} # Intersection, isdisjoint = False
s3 = {5,6} # No intersection, isdisjoint = True
print(s1.isdisjoint(s2))
print(s1.isdisjoint(s3))
False
True
Make a Copy of a Set in Python: copy()
Use the copy() method to return a shallow copy of the set in python.
# copying a set
s1 = {1, 2, 3}
s2 = s1.copy()
s2
{1, 2, 3}
Remove all Elements from a Set
To remove all elements from a set, use the clear() method on the set object.
# clear
s = {1, 2, 3}
s.clear()
s
set()
Python Sets Cheatsheet
Python.org provides the following table explaining all the operations that can be applied to Python sets:
Functions, Methods and Operations | Equivalent | Result |
---|---|---|
len(s) | number of elements in set s (cardinality) | |
x in s | test x for membership in s | |
x not in s | test x for non-membership in s | |
s.issubset(t) | s <= t | test whether every element in s is in t |
s.issuperset(t) | s >= t | test whether every element in t is in s |
s.union(t) | s | t | new set with elements from both s and t |
s.intersection(t) | s & t | new set with elements common to s and t |
s.difference(t) | s - t | new set with elements in s but not in t |
s.symmetric_difference(t) | s ^ t | new set with elements in either s or t but not both |
s.isdisjoint(t) | boolean telling if sets have intersection | |
s.copy() | new set with a shallow copy of s | |
| s |= t | return set s with elements added from t |
| s &= t | return set s keeping only elements also found in t |
| s -= t | return set s after removing elements found in t |
| s ^= t | return set s with elements from s or t but not both |
| add element x to set s | |
| remove x from set s; raises | |
| removes x from set s if present | |
| remove and return an arbitrary element from s; raises | |
| remove all elements from set s |
Frozenset
In Python, a frozenset
is an immutable set, meaning its elements cannot be modified. It is similar to a set
. The key difference is that a frozenset
is immutable, while a set
is mutable.
This means that you cannot add, remove, or modify elements in a frozenset
after it is created. The immutability of frozenset
makes it useful in situations where you need to use sets as keys in dictionaries or as elements in other sets.
# Creating a set
s1 = {1, 2, 3}
s1.add(4)
print(s1)
# Creating a frozenset
fzset = frozenset({1, 2, 3})
fzset.add(4) # Raises AttributeError
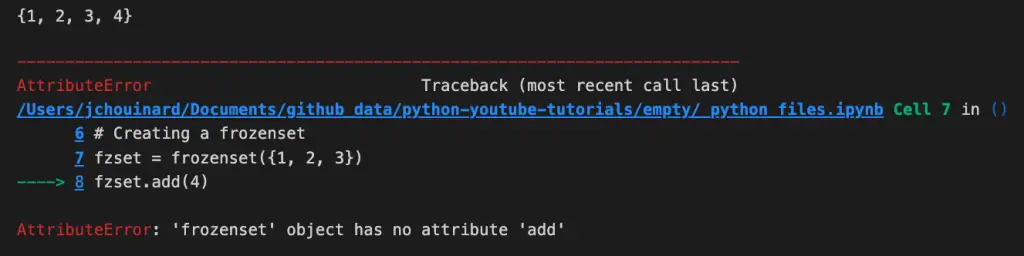
Since frozenset
is immutable, it is hashable and can be used as a key in dictionaries or as an element in other sets. Regular set
objects are not hashable.
# Creating a set
s1 = {1, 2, 3}
fzset = frozenset({1, 2, 3})
# Using a frozenset as a key in a dictionary
my_dict = {fzset: 'Value'}
print(my_dict)
# Using a set as a key in a dictionary
my_dict = {s1: 'Value'} # Raises TypeErrorL unashable type
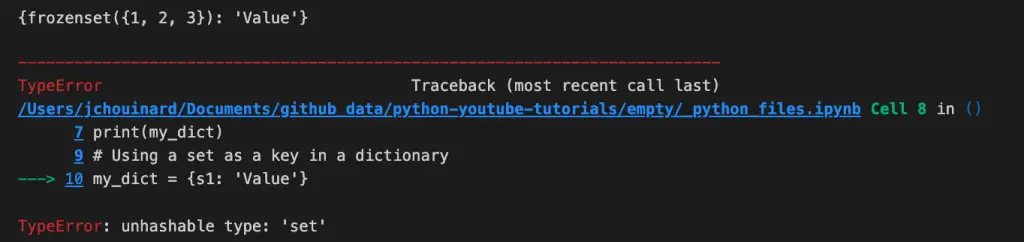
How to Create a Set of Sets in Python
To create a set of sets in Python, use a frozenset inside your regular set.
If you try to create a set that contains another set, you are likely to run into the TypeError: unhashable type: 'set'
.
# set of sets
s = {1,2, {3, 4}}
s
The TypeError: unhashable type: 'set'
error is caused because regular set objects are not hashable and cannot be used in other sets because they are mutable.
To create a set of sets in Python and fix the TypeError: unhashable type: 'set'
, use a frozenset.
s = {1,2, frozenset({3, 4})}
s
{1, 2, frozenset({3, 4})}
Creating an Ordered Set in Python
To create an ordered set in Python, you need to install ordered-set.
$ pip3 install ordered-set
from ordered_set import OrderedSet
s1 = OrderedSet([1,2,2,3])
print(s1)
OrderedSet([1,2,3])
Conclusion
We have learned about Python sets objects, how to create them, and the most important sets methods.
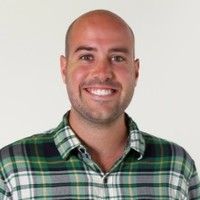
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.