In Python programming, a data type is the classification of data that defines the possible operations that can be done on the object.
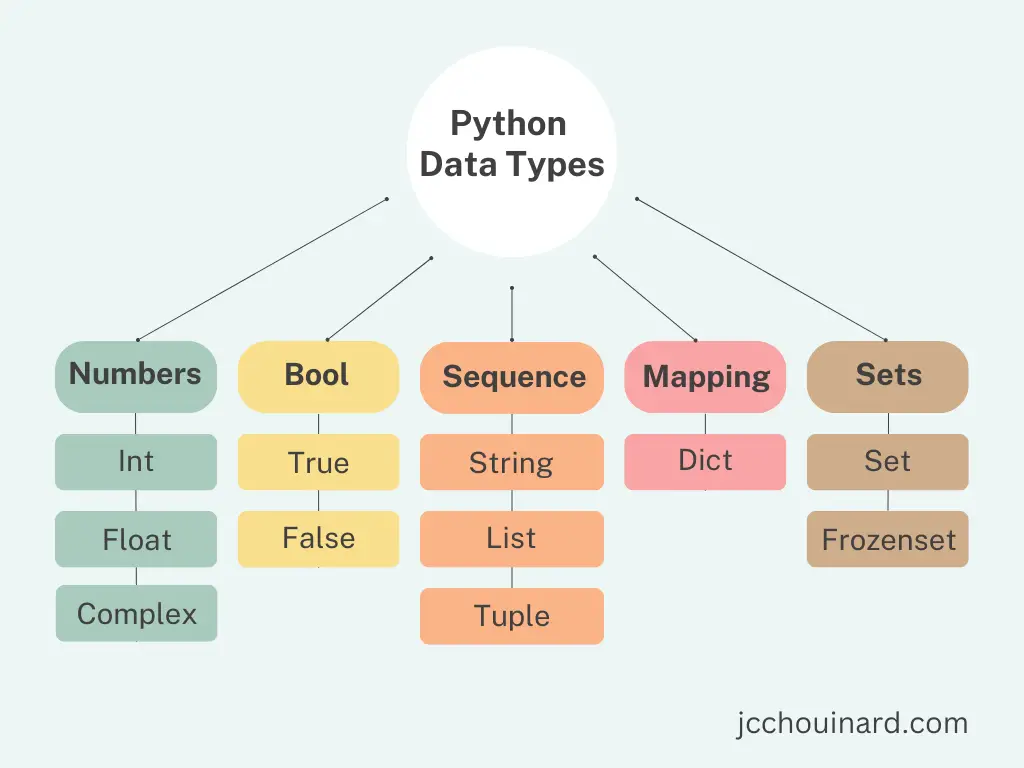
Python has various built-in types in the interpreter: numerics, sequences, mappings, classes, instances and exceptions.
This tutorial will show you all the Python data types and each of their characteristics.
Main Python Data Types
There are 5 types of main data types categories available in Python:
- Numbers: Int, Float, Complex
- Booleans
- Sequences: String, List, Tuple
- Mapping: Dict
- Sets: Set, Frozenset
Showing the Data Type of a Variable
To show the data type of a variable or an object in Python, use the type()
function.
x = 1
print(type(x))
To verify if an object belongs to a particular class, use the isinstance()
function.
x = 1
print(isinstance(x, int))
Python Data Types by Category
Built-in Python data types can be categorized based on their sets of characteristics: mutable, possible arithmetic operations, allowing indexing, etc.
Category | Type |
---|---|
text type | str |
numeric types | int, float, complex |
sequence types | tuple, list, range |
mapping type | dict |
sets type | set, frozenset |
binary types | bytes, bytearray, memoryview |
boolean type | bool |
date and time types | date, time, datetime, timedelta, tzinfo |
class type | class (user-defined type) |
instances types | object (base for all objects), instance |
exception types | Exception (base for all built-in exceptions) |
NoneType | NoneType |
Python Data Types
Bool Data Type
In Python, the bool
data type is used to represent boolean values (True
, False
). Booleans are used to evaluate expressions and return the boolean True
or False
based on the result of the expression.
Python Bool example
# Boolean expression
x = 10
y = 5
result = x > y
print(result) # True
print(type(result)) # <class 'bool'>
Str Data Type
In Python, the str
data type is used to define text component enclosing a sequence of characters within single-quotes or double-quotes. Python strings can contain letters, numbers or special characters.
Python Str example
# String data type
platform = "JC Chouinard"
print(type(platform)) # <class 'str'>
# Print string
print(platform) # JC Chouinard
List Data Type
In Python, the list
data type is used to store ordered sequence of elements. Python lists are ordered collections that can contain elements of various data types (str, list, dicts, …). List elements can be accessed, iterated, and removed.
Slicing or accessing elements of a list is done using the square brackets ([]
) notation.
Creating a list is done using the square brackets ([]
) or the list()
constructor function
Python list example
# List data type
ls = ['hello', 1, True, [1, 2]]
# prin list
print(ls) # ['hello', 1, True, [1, 2]]
print(type(ls)) # <class 'list'>
# Accessing first element of a list (zero-based indexing)
print(ls[0]) # hello
# slicing list elements
print(ls[2:4]) # [True, [1, 2]]
# update elements of a list
ls[1] = 20
print(ls[1]) # 20
# list constructor
ls2 = list((1,2,3))
print(ls2) # [1, 2, 3]
Tuple Data Type
In Python, Tuples are a data structure of the sequence type that store a collection of data. Python Tuples have these 5 characteristics.
- ordered
- unchangeable
- immutable
- allow duplicate value
- allow values with multiple data types
Creating a tuple is done using the parentheses (()
) or the tuple()
constructor function.
Python Tuple example
# tuple data type
t = (1, 2, 3, 4, 5)
print(t) # (1, 2, 3, 4, 5)
print(type(t)) # <class 'tuple'>
# Indexing a Tuple
print(t[0]) # 1
# Slicing a Tuple
print(t[1:4]) # (2, 3, 4)
# tuple constructor
t2 = tuple([1,2,3])
print(t2) # (1, 2, 3)
Range Data Type
In Python, the range data type comes from the range()
built-in function used to generate a sequence of numbers. The range()
function returns an iterator object generated by defining start, stop and increment numbers.
Python Range() example
# Range()
rg = range(5)
print('Range:', rg) # Range: range(0, 5)
print(type(rg)) # <class 'range'>
# Looping a Range
for i in rg:
print(i) # 0 1 2 3 4
# Range Parameters
rg2 = range(
4, # Start
14, # End
2 # Increment
)
print('Incremented range:', rg2) # Incremented range: range(2, 20, 2)
for x in rg2:
print(x) # 4 6 8 10 12
Dict Data Type
In Python, dictionaries are an unordered collection of key-value pairs stored as a dict
data type. Python dictionaries are a mappings data type used to store collections of data.
Creating a dictionary is done adding key, value pairs inside curly brackets ({}
) or using the dict()
constructor function.
Values in dictionaries can be selected passing the key string inside the square brackets ([]
) of the dictionary object.
Python dict example
# Dict data type
d = {
'name': 'JC',
'last_name': 'Chouinard'
}
# print dictionary
print(d) # {'name': 'JC', 'last_name': 'Chouinard'}
print(type(d)) # <class 'dict'>
# access dictionary values
print(d['name']) # JC
# dict() constructor
d2 = dict(name='Jean-Christophe',last_name='Chouinard')
print(d2) # {'name': 'Jean-Christophe', 'last_name': 'Chouinard'}
Set Data Type
In Python, sets are an unordered collection unique elements (no duplicate values). A Python set
is a mutable object where you can add, remove, or modify elements after creating it.
Creating a set is done adding comma-separated values inside curly brackets ({}
) or using the set()
constructor function.
Python Sets have a multiple characteristics.
- Duplicates are not allowed
- They can have Multiple data Types
- Sets Can’t Be Accessed with the Index
- Not Subscriptable
Python set example
# Python sets data type
s = {1,2,3}
# print set
print(s) # {1, 2, 3}
print(type(s)) # <class 'set'>
# set() constructor
s2 = set([1,2,3])
print(s2) # {1, 2, 3}
# Using the set() constructor
# allows to deduplicate a list
ls = [1, 2, 3, 4, 3, 3, 4, 5, 5, 5]
print(set(ls)) {1, 2, 3, 4, 5}
# not subscriptable
s3 = set((1,2,3))
s3[0] # TypeError: 'set' object is not subscriptable
Frozenset Data Type
In Python, the frozenset
data type represents an immutable set.
Creating a frozenset
is done using the frozenset()
constructor function. Once created, you cannot add, remove, or modify elements to the frozenset
.
The difference between a set
and a frozenset
in Python is that the set
is mutable and the frozenset
is not. You can change a set by adding, removing or modifying its elements, but doing so with a frozenset
would result in an error.
Python frozenset example
# Python set VS frozenset
# set
my_set = {1, 2, 3, 4}
my_set.add(5)
my_set.remove(2)
print(my_set) # {1, 3, 4, 5}
# frozenset
my_frozenset = frozenset([1, 2, 3, 4])
# Adding or removing elements from frozenset returns an error
# my_frozenset.add(5) # AttributeError
# my_frozenset.remove(2) # AttributeError
Int Data Type
In Python, the int
data type is a numeric type used to represent whole integer number as opposition with float
number.
Creating a int
is done by using the whole integer number without quotes or using the int()
constructor function.
Python int example
# Python float data type
i = 3
# print set
print(i) # 3
print(type(i)) # <class 'int'>
# int() constructor on string
i2 = int('5')
print(i2) # 5
# int() constructor on float
i3 = int(1.6) # rounds to the lower bound integer
print(i3) # 1
# Rounding floats
i4 = round(1.6)
print(i4) # 2
print(type(i4)) # <class 'int'>
# Int allows arithmetics
print(i * (i2 + i3)) # 18
Float Data Type
In Python, the float
data type is a numeric type that is used to represent number with floating point values as opposition with whole int
number.
Creating a float
is done by using the number using the decimal point (.
) without quotes or using the float()
constructor function.
Python float example
# Python float data type
f = 3.1
# print set
print(f) # 3.1
print(type(f)) # <class 'float'>
# float() constructor on string
f2 = float('5.1')
print(f2) # 5.1
# float() constructor on int
f3 = float(1)
print(f3) # 1.0
Complex Data Type
In Python, the complex
data type represents complex numbers that contain a real part and an imaginary part. In complex numbers, the real part and the imaginary part are floating-point numbers denoted by the suffix “j” or “J”.
Complex numbers are used often in mathematical and scientific calculations.
Python complex example
# Creating complex numbers
z1 = 2 + 5j # Real part: 2, imaginary part: 5
z2 = -1.2 + 3.2j # Real part: -1.2, imaginary part: 3.2
# Print complex numbers
print(z1) # (2+5j)
print(z2) # (-1.2+3.2j)
# Print complex type
print(type(z1)) # <class 'complex'>
print(type(z2)) # <class 'complex'>
# Accessing real and imaginary parts
print(z1.real) # 2.0
print(z1.imag) # 5.0
# Arithmetic operations with complex numbers
z3 = z1 + z2 # Addition
z4 = z1 * z2 # Multiplication
print(z3) # (0.8+8.2j)
print(z4) # (-18.4+0.40000000000000036j)
Bytes Data Type
In Python, the bytes
data type is a binary type that is used to represent sequences of bytes (0s and 1s). A byte is a sequence of 8 bits, each bit being a 0 or a 1. Python bytes are used to manipulate binary data.
The bytes
data type is useful when working with file formats such as images, videos, audio files or when you need efficient storage an processing.
Bytes object can be created in Python using the string.encode()
method, using the b''
byte notation or using the bytes()
constructor function.
Python bytes example
# Convert string to bytes
s = "Hello"
byte = s.encode()
print(byte) # b'Hello'
print(type(byte)) # <class 'bytes'>
# Convert bytes to string
decoded = byte.decode()
print(decoded) # Hello
# Create a bytes from literal representation
byte2 = b'\x48\x65\x6c\x6c\x6f'
print(byte2) # b'Hello'
print(type(byte)) # <class 'bytes'>
# Create a bytes from integers
byte3 = bytes([72, 101, 108, 108, 111]) # Represents "Hello"
print(byte3) # b'Hello'
# Accessing individual bytes
print(byte3[0]) # 72
# Iterating over bytes
for byte in byte3:
print(byte) # 72 101 108 108 111
# Bytes can't be modified
byte3[0] = 10 # TypeError: 'bytes' object does not support item assignment
Bytearray Data Type
In Python, the bytearray
data type represents a mutable sequence of bytes. Python bytearray
objects are Similar to the bytes
data type, but that can be modified after their creation.
Python bytearray
objects can be created using the bytearray()
constructor function.
Python bytearray
example
# Creating a bytearray object
data = bytearray(b'Hello')
print(data) # bytearray(b'Hello')
# Modifying the bytearray
data[0] = 72 # Modifying the first byte
data.append(33) # Appending a new byte
print(data) # bytearray(b'Hello!')
# Converting bytearray to bytes
bytes_data = bytes(data)
print(bytes_data) # b'Hello!'
Memoryview Data Type
In Python, the memoryview
data type is used to access and manipulate the underlying memory of an object. It enables to view the internal data of an object that support the buffer protocol (bytes, bytearrays, etc) without copying it. With memoryview
, you can perform operations on the memory directly, without making unnecessary copies.
Python
objects can be created using the memoryview
constructor function.memoryview
()
Python memoryview
example
# Creating a bytes object
data = b'jcchouinard'
# Creating a memoryview object
mv = memoryview(data)
print(mv) # <memory at 0x299beb880>
print(type(mv)) # <class 'memoryview'>
# Accessing and modifying the memoryview
print(mv[0]) # 106 (the ASCII value for 'j')
Python Data Types Characteristics
Each data type in Python has its own set of characteristics. Here are the characteristics of the most often used data types.
For example, certain data type can be mutable( e.g. lists), others immutable (e.g. sets). Similarly, some allow indexing and others not.
Data Type | Data Type Category | Immutable | Allow Arithmetic Operations | Allow Indexing | Allow Slicing |
---|---|---|---|---|---|
list | Sequence | No | Yes | Yes | Yes |
tuple | Sequence | Yes | No | Yes | Yes |
range | Sequence | Yes | No | Yes | Yes |
dict | Mapping | No | No | Yes | No |
set | Set | No | No | No | No |
frozenset | Set | Yes | No | No | No |
int | Numeric | Yes | Yes | Yes | Yes |
float | Numeric | Yes | Yes | No | No |
complex | Numeric | Yes | Yes | No | No |
str | Text | Yes | No | Yes | Yes |
bytes | Byte | Yes | No | Yes | Yes |
bytearray | Byte | No | Yes | Yes | Yes |
memoryview | Byte | No | Yes | Yes | Yes |
bool | Boolean | Yes | Yes | No | No |
Python Data Types Methods
Python Data Types come built-in with various sets of methods that can be used on their objects. Below are examples of Python methods that can be used on each data type’s object.
Data Type | Example Built-in Methods |
---|---|
list | append(), insert(), pop() |
tuple | count(), index() |
range | start, stop, step |
dict | clear(), keys(), values() |
set | add(), remove(), union() |
frozenset | intersection(), isdisjoint() |
int | bit_length(), to_bytes() |
float | as_integer_ratio() |
complex | real, imag |
str | lower(), upper(), strip() |
bytes | decode(), hex() |
bytearray | append(), pop() |
memoryview | cast() |
date | today() |
When To Use Each Data Type?
Each data type in Python has its own set of rules and use. Below is a table showing when to use each data type and how to set it up in Python.
Data Type | When to Use It | Setting the Data Type |
---|---|---|
list | When you need a mutable ordered sequence of elements | [1, 2, 3] |
tuple | When you need an immutable ordered sequence of elements | (1, 2, 3) |
range | When you need an immutable sequence of numbers | range(10) |
dict | When you need to map keys to values, and you need to be able to change the mappings | {‘key1’: ‘value1’, ‘key2’: ‘value2’} |
set | When you need a mutable set of unique elements | {1, 2, 3} |
frozenset | When you need an immutable set of unique elements | frozenset({1, 2, 3}) |
int | When you need a whole number with no decimal point | 42 |
float | When you need a number with a decimal point | 3.14 |
complex | When you need a number with a real and imaginary part | 2 + 3j |
str | When you need a string of characters | “hello world” |
bytes | When you need a sequence of bytes | b”hello” |
bytearray | When you need a mutable sequence of bytes | bytearray(b”hello”) |
memoryview | When you need to access the internal memory of an object | memoryview(b”hello”) |
bool | When you need a boolean value (True or False) | True or False |
Data Types Constructors
To specify data types in Python, you can use Python constructor functions:
Data Type | Constructor Example |
---|---|
list | my_list = list([1, 2, 3, 4]) |
tuple | my_tuple = tuple((1, 2, 3, 4)) |
range | my_range = range(10) |
dict | my_dict = dict({‘key1’: ‘value1’, ‘key2’: ‘value2’}) |
set | my_set = set([1, 2, 3, 4]) |
frozenset | my_frozenset = frozenset([1, 2, 3, 4]) |
int | my_int = int(10) |
float | my_float = float(3.14) |
complex | my_complex = complex(2, 3) |
str | my_str = str(‘Hello, World!’) |
bytes | my_bytes = bytes([0x41, 0x42, 0x43, 0x44]) |
bytearray | my_bytearray = bytearray([0x41, 0x42, 0x43, 0x44]) |
memoryview | my_memoryview = memoryview(b’Hello, World!’) |
bool | my_bool = bool(True) |
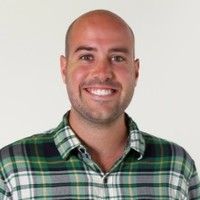
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.