In this tutorial, you will learn how to list every Python packages installed in your environment, whether you are using pip
, conda
or pipenv
package management systems for Python.
Follow this article for those interested in learning how to use pip to install and manage Python packages. For those interested in how to use the command prompt.
Cheatsheet on how to List Installed Packages in Python
Package Manager | Command to list installed Package |
---|---|
pip | $ pip list |
Anaconda | $ conda list |
Pipenv | $ pipenv lock -r |
Python Console | >>> import pkg_resources OR >>> help("modules") |
How to List Installed Python Packages with Pip
List Installed Python Packages (pip list)
To view all the packages installed in a Python environment, use the pip list
command.
$ pip list
Package Version
------------------------ ------------
advertools 0.13.2
pandas 2.0.1
pip 23.2.1
...
List Installed Python Packages (pip freeze)
To list the installed packages without including the package management tools such as pip
and setuptools
by using the pip freeze
command.
$ pip freeze
The output will show installed packages that are not package management tools.
advertools==0.13.2
pandas==2.0.1
Difference Between pip list and pip freeze
The main difference between pip list
and pip freeze
is that pip freeze
does not include packages used for package management, such as pip
and setuptools
.
For example, here are the outputs of pip list
and pip freeze
for the same Python environment.
Pip list
Package Version
------------------------ ------------
advertools 0.13.2
pandas 2.0.1
pip 23.2.1
...
Pip freeze
advertools==0.13.2
pandas==2.0.1
You can however, use the pip freeze –all flag to show all the versions including packages managers.
$ pip freeze --all
advertools==0.13.2
pandas==2.0.1
pip=23.2.1
The other big difference is that pip freeze can be used to create a requirements.txt file.
$ pip freeze > requirements.txt
$ pip install -r requirements.txt
Here’s the official documentations for both commands:
Select the output format (pip list –format)
To select the output format, use the --format
flag with the pip list
command.
How to show the pip list output in columns
$ pip list --format columns
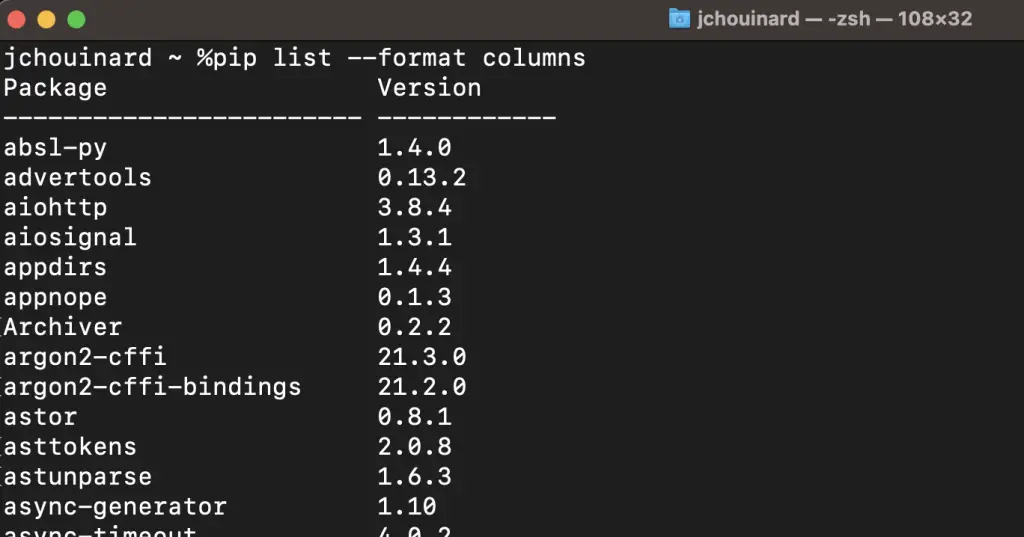
How to Show the pip list Output in the JSON format
$ pip list --format json
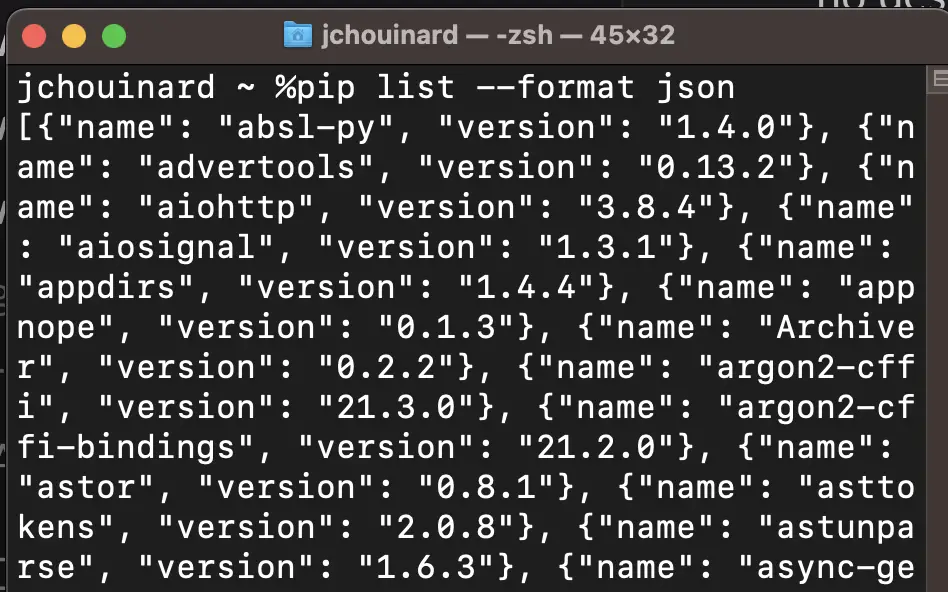
How to Show the pip list Output in the pip freeze format
$ pip list --format freeze
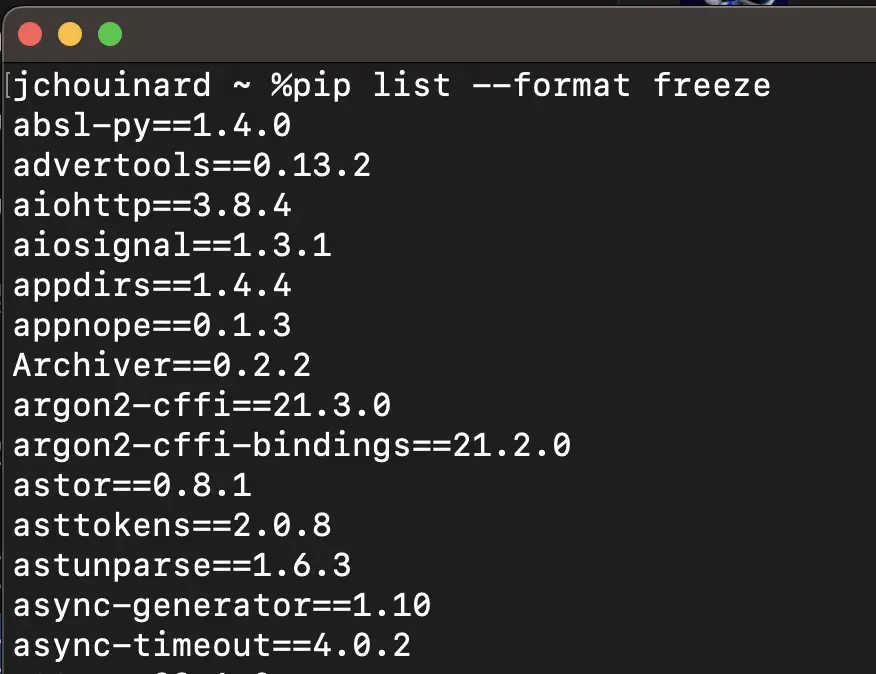
Pip list up-to-date packages (–uptodate)
To list only the up-to-date Python packages, use the pip list -u
or --uptodate
command flags.
$ pip list -u
$ pip list --uptodate
Pip list outdated packages (–outdated)
To list only the outdated Python packages, use the pip list --outdated
or -o
command flags.
$ pip list -o
$ pip list --outdated
Note that you cannot use the --outdated
flag along with the freeze format.
ERROR: List format 'freeze' cannot be used with the --outdated option.
Pip list packages not required by other packages (–not-required)
To list only the Python packages that are not required by other packages, use the pip list --not-required
command flags.
$ pip list --not-required
The --not-required
flag is useful to know if it is safe to uninstall a package without breaking the dependencies for other packages.
How to List Installed Python Packages without Pip
It is possible that you are not using the pip
Python package manager in your project. You could be using Anaconda or Pipenv for example.
How to List Installed Python Packages with Console
To list all the packages installed using pip
from the Python Interpreter console, import the pkg_resources
library.
import pkg_resources
packages = pkg_resources.working_set
packages_list = ["%s==%s" % (i.key, i.version) for i in packages]
print(packages_list)
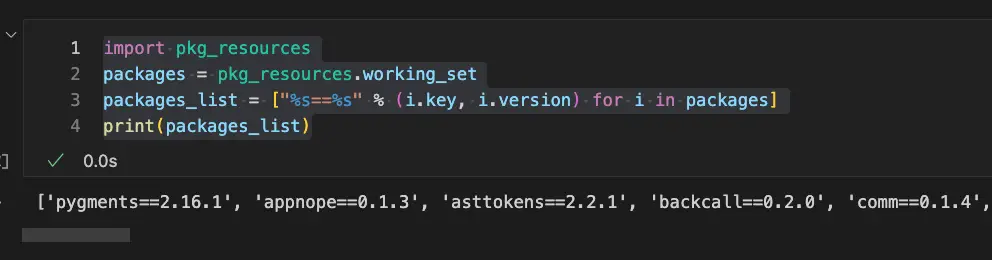
To list all the packages installed without pip
from the Python Interpreter console use the help("modules")
function. Beware this function can take a long time and also risk infinite loops in some cases.
>>> help("modules")
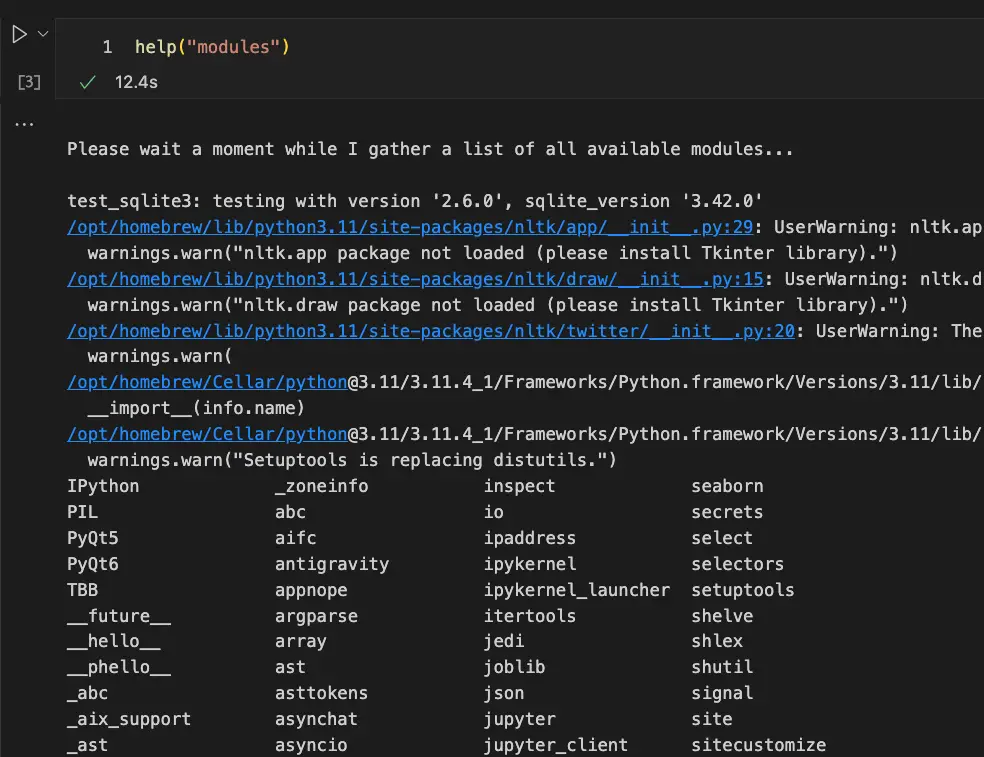
How to List Installed Python Packages with Conda
To list all the packages installed using the Anaconda, use the conda list
command in the Anaconda Navigator.
$ conda list
How to List Installed Python Packages with Pipenv
To list all the Python packages installed, including all dependencies, in a pipenv
environment, used the pipenv lock -r command.
$ pipenv lock -r
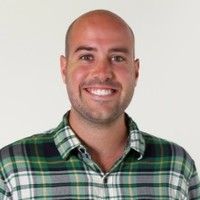
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.