Python is a programming language created by Guido Van Rossum that was designed for code readability and released in 1991.
This is a comprehensive guide to Python programming.
Here is what you are going to learn.
What is Python?
Python is an open-source interpreted programming language that was created by Guido Van Rossum that can be used as a general-purpose to build almost any piece of software.
Python has built its popularity by being free to use, its easily shareable packages, and its ease of integration with C and C++.
What are the Python Versions
The current Python version is the version 3. Python 0.9.0 was released in 1991, Python 2.0 was released in 2000 and Python 3.0 was released in 2008.
Version 2 will not be maintained past 2020.
Why people still use version 2 of Python?
This is mainly because the Python Software Foundation wanted to start over by creating Version 3 of Python. So, if you are working with V3, you can’t work with files that are using V2. So, a lot of packages can’t be called using Python V3.
What is Python Used For
Python is a programming language used for web development, data analysis and visualization, machine learning, artificial intelligence, automation, and many other tasks. Python’s extensive libraries and frameworks make it suitable for a wide range of users and applications.
How to Install Python?
To install Python, the preferred solution is to install the Python executable file through the Python’s foundation website. However, there is no single solution to help you set-up Python and the required packages.
I have crafted multiple articles to show you how to install Python 3 on MacOS and Windows.
How to Use Python
To use Python, it is required to have a version of the Python executable file installed in your working environment.
The simplest way to start using Python instantly is to use Google Colab where Python, and some of the most important libraries are already pre-installed.
Python is a programming language like C or C++. It is not software that you can start using instantly like Microsoft Word or Excel.
Understand The Python Tools
In order to run a Python code or Python Script, you will need an interpreter to write, test and debug your Python commands.
You can either use a simple IPython Shell with a text editor, an Integrated Development Environments (IDEs), or a notebook like Jupyter to do this task. IDEs, like Spyder, are easy to use interface for beginners in Python.
You can use either one of the 4 solutions, but since, I prefer to use VSCode.
IPython Shell
The Python Shell, or Python Interactive Shell, is a place where you can type Python code and immediately see the results. IPython is basically a shell, with more features.
The IPython Shell runs line-by-line. So, to run 10 lines, you will need to make 10 commands of a single line.
Combined with a text editor like notepad++, it can be a powerful tool. If you have installed Python using Anaconda, you can open the IPython Shell using Anaconda Prompt, if not using the Command Prompt native to Windows, and typing these commands.
## Install IPython with Pip (only the first time)
pip install ipython
## Install IPython with Conda (only the first time)
conda install ipython
## Start using IPython
ipython
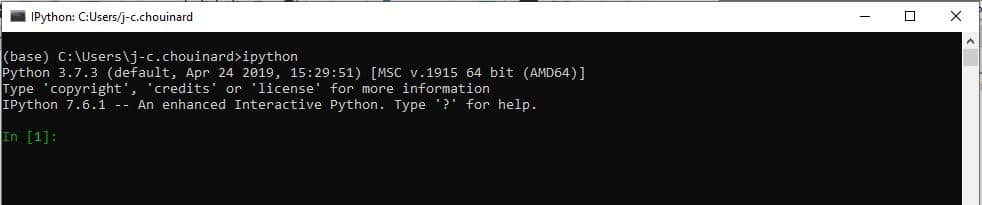
To learn more about using the IPython Shell, just look at this great guide by Codecademy.
Python Integrated Development Environments (IDEs)
You might have heard of applications like Python Tools for Visual Studio Code, PyCharm, Spyder, Komodo IDE. These are Integrated Development Environments or IDEs.
Basically, an IDE is an application, like word, that lets you build, compile and run Python Scripts from a single interface.
My favourite Python tool is VSCode.
Python Notebooks
A Python Notebook, like Jupyter Notebook or IPython Notebook, is free and open-source, browser-based environments that let you write, read and share your Python code for data analysis.
If you are interested to learn more about Jupyter Notebook, you can read this complete guide on How to use Jupyter Notebook.
What Are Python Scripts?
A Python script is a text file, with the extension .py (scripts.py
), with a list of Python commands that are executed. You can add multiple code lines in a Python Script and execute them in a single command.
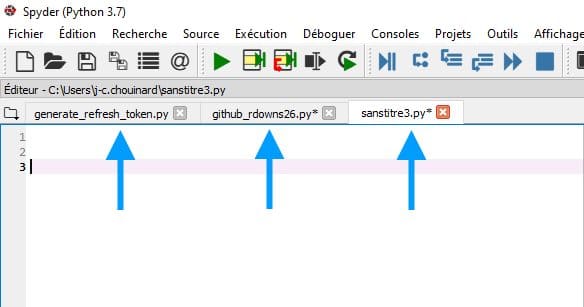
Let’s say you have a Python code that needs 10 lines to be executed.
Unlike the Python Shell, a Python Script will run the code in 10 lines and 1 command, and execute it line-by-line.
Why Use Python Scripts?
Python scripts will help you to keep a structure. When you need to make a change to your code, instead of manually retyping every step, as you would do in the shell, you simply make the change in the script and rerun the entire code.
In this guide, I will use Spyder IDE, since it is so convenient to use, and so closely resemble RStudio, that it will be easy if you want to learn R Programming later on.
Note that if you have installed Python using Anaconda as we have seen earlier, Spyder IDE will be installed on your PC.
Don’t worry if you don’t know how to use them, I have made a simple guide on Spyder IDE for Python.
Learn Python
Learning python is useful in web development, machine learning, automation, web scraping, use apis. To get you started, I will show you some basic concepts like doing arithmetics with Python and understanding variables, types, functions, and methods.
But wait.
Don’t leave before you have seen what you can do with Python packages. I promise you are absolutely going to love this.
First, let’s run over the basic concepts.
Python Syntax
Python has an extensive language reference manual that describes the core semantics of the Python programming language including the line structure, the identifiers, literals, operators, delimiters, etc.
Python Operators
Python Operators can be used to perform arithmetics or assignments of variables and values. Python has 21 tokens that serve as operators in its syntax.
1 + 5 # additions
5 - 1 # Substractions
2 * 5 # multiplications
4 ** 2 # Exponentiation
10 / 2 # Division
10 % 2 # Modulo
1 == 2 # check if equal
Here is a quick guide to help you master Python operators.
Read the following article to view the full list of Python operators additional Python operators possible.
Python Delimiters
Python delimiters are tokens, or sequence of tokens, used in the Python grammar that defines the boundaries between sections of the code. Examples of Python delimiters are the commas (,), the braces ({}) or the parentheses (()).
Some of the ASCII characters have special meaning important for the lexical analyzer:
' " # \
Here is the entire list of Python delimiters
( ) [ ] { }
, : . ; @ = ->
+= -= *= /= //= %= @=
&= |= ^= >>= <<= **=
Python Keywords
Python Keywords are reserved words of the python programming language that have a specific meaning and can’t be used outside of there context. Examples of keywords are the if
keyword (used to define conditionals) and the import
keyword (used to import packages and modules).
Python keywords must be spelled with this exact casing:
False await else import pass
None break except in raise
True class finally is return
and continue for lambda try
as def from nonlocal while
assert del global not with
async elif if or yield
Python Built-in Types
The 6 Python built-in types are numerics, sequences, mappings, classes, instances and exceptions.
- Boolean Operations — and, or, not
- Numeric types: int, float, complex
- Sequence Types: list, tuple, range
- Mapping Types: dict
- Iterator Types
- Text Sequence Type: str
- Binary Sequence Types: bytes, bytearray, memoryview
- Set Types: set, frozenset
- Context Manager Types
- Type Annotation Types” Generic Alias, Union
Make a Comment Using The Hashtag (#)
Comments in Python begin with the hashtag character (#). This way, Python does not execute anything that follows a hashtag on a line.
This is why hashtags are so important in Python. They let you add annotations to your code that humans can understand, but that your computer will pass over.
For the remainder of this guide, I will use hashtags to display the output of Python code, just like this:
1+5
## 6
A single hashtag (#) will be used to add my own comments.
A double hashtag (##) will be used to display the results of code.
I will avoid using the “>>>” and “In [1]” unless I need it.
Python Variables
Python variables are containers that store data values.
With Python, you can make simple arithmetics like 3 + 5 = 8, but you can also make more complex calculations.
As you go along those complex equations, you might want to store some values, along with a specific name, to help you move along your code. To “save” those values in Python, you need to create variables.
Declaring a Variable
By declaring a variable, you basically attribute a value to a variable, that you can later call using the variable name. The name is case-sensitive.
If you now type the name of the variable, Python looks for the variable name, retrieves its value, and prints it out.
Example
Let’s say you want to calculate the average score in each of your classes. You might want to create variables for each of your classes.
maths = 89.6
french = 75.9
science = 81.3
maths
## 89.6
To find your average score, you would need to add up your classes’ scores and divide them by the number of classes. You could do this in two ways:
average = (89.6 + 75.9 + 81.3) / 3
average
## 82.26
or
average = (maths + french + science) / 3
average
## 82.26
Modify or Delete a Variable
Anytime that you declare a variable, it stays stored on your computer. If you want to delete a variable, use the del()
function.
variable = [1,2,3,4]
print(variable)
##[1, 2, 3, 4]
#Change a variable
variable = [2,5,6,8]
print(variable)
##[2,5,6,8]
#Delete variable
del(variable)
print(variable)
"""
Traceback (most recent call last):
File "<ipython-input-245-ad7925581c6b>",line 2, in <module>
print(variable)
NameError: name 'variable' is not defined
"""
Understand and use Python Data Types
The example above only attribute numerical values to variables. However, there are many types that can be used in Python. To know what type is your value, you just need to write type(value) in your shell.
If you want to know the type our variable average that we just created, just input type(average)
type(average)
## float
What Are The Python Types?
There are many types in Python. The most commonly used are:
Float: Real number that includes all decimal points. Example: 26.26666667
Int: Integer. Example: 26
Str: Strings. Example: “Hello World”
Bool: Booleans that can only be answered by True or False Example: y = True
Note: Python Data Types Can Behave Differently
Python data types will react differently to different operators. For example, if you sum integers, the result you will get is the sum of your integers. However, if you sum strings, the result you will get is the strings pasted together.
Integers
1+1
## 2
Strings
“I am” + “ a child”
## “I am a child”
This is really important.
How the code behaves depends on the type that you are working with.
Different type = different behavior
Convert Data Types
If you want to do operations between data types, you will need to convert all data types to the same data type. To do so, refer to the guide below.
str() = Convert any type to a string
int() = Convert any type to an integer
float() = Convert any type to a floating point number
bool() = Convert any type to a boolean (true or false)
Python Lists
Python lists are one of the 4 data types used to store collections of data.
Like other programming languages, you can use lists to store multiple data points in Python.
Easiest Way to Build a List in Python
Let’s say that you would like to store all your Maths results as variables in Python. You could store them like this:
maths1 = 89.5
maths2 = 92.1
maths3= 32.5
maths4 = 75.6
Or, alternatively, you could use square brackets [ ] to store your data into a list.
maths = [89.5 , 92.1 , 32.5, 75.6]
maths
## [89.5 , 92.1 , 32.5 , 75.6]
How to Name Lists in Python
To name a list variable in Python, just assign the list to a variable in square brackets.
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
maths
## ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
Make sure that your name is
- Short
- Descriptive
- Start with letter or underscore
- Alphanumeric
- Case sensitive
Preferably use snake_case format to name your variables.
How to Create Lists Into Lists in Python
You could add lists within a list. To create those sublists
mathssublist = [["Q1",89.5],["Q2",92.1],["Q3",32.5], ["Q4",75.6]]
mathssublist
## [["Q1",89.5],["Q2",92.1],["Q3",32.5],["Q4",75.6]]
Python Indexing and Lists Subsetting
In Python, subsetting is the process of retrieving just the parts of a list. Python does this by using integers. This indexing allows you to get, change or delete only a single cell of a list.
Each element of a list has its own integer attributed to.

In our list, to get the result 32.5, all I would do is call it by its integer.
maths[5]
##32.5
or by calling it with its negative index.

maths[-3]
##32.5
Now, let’s look at how we can select data from a list using list slicing.
Basics of List Slicing
This is truly a beginner Python move to learn.

To select multiple indexes, all you have to do is to use the colon “:” within the brackets. Just understand that the first number is inclusive and the last number is non-inclusive.
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5"Q4",75.6]
maths[2:4]
##["Q2",92.1]
In our example, the fourth value will not figure in the output.
What Happens If You Leave Out a Value?
You can leave out either the value before or after the colon. What will happen is that the index will be considered to have a value of “0”.
Like this:
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5"Q4",75.6]
maths[:4]
##["Q1",89.5,"Q2",92.1]
Or like this:
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5"Q4",75.6]
maths[4:]
##["Q3",32.5"Q4",75.6]
You now know how to select data from a list.
So what?
Now let’s dive into the cool stuff.
6 Useful Manipulations You Can Do With Lists
Okay.
We understand what indexing and list subsetting are, and we know how to select some data from a list using list slicing.
Let’s see what manipulations we can do to modify lists using Python Indexes.
1. Change a Single Value in a List With Python
To replace values in a list using python, you need to use the square brackets that we used for subsetting a list and assign new elements to it.
Let’s say we re-took our Q3 maths exam and want to change the score.
maths=["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
maths[5] = 96.4
maths
## maths=["Q1",89.5,"Q2",92.1,"Q3",96.4,"Q4",75.6]
Easy.
Now, let’s replace more than one value in our list.
2. Change Multiple Values of a List With Slice Notation
To change multiple items in a list at one time in Python, we will use the same strategy as before. All we have to do is subset vectors and assign new values.
We retried on Q3 and Q4 exams.
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
maths[4:8] = ["Q3",100,"Q4",100]
maths
## maths = ["Q1",89.5,"Q2",92.1,"Q3", 100 ,"Q4",100]
Congrats!
Believing this Python Course, you are a pro in maths.
3. Create a sublist from a list using slicing
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
mathssublist = maths[2:4]
mathssublist
##["Q2",92.1]
4. Subset and Calculate Values
maths = [89.5,92.1,32.5,75.6]
mathsavg = (maths[0]+maths[1]+maths[2]+maths[3])/4
print(mathsavg)
##72.425
or
maths = [89.5,92.1,32.5,75.6]
mathsavg = sum(maths[0:4])/4
print(mathsavg)
##72.425
5. Add And Remove Values From a List
To add a new element to a list you can use the plus “+” operator.
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
Q5 = maths + ["Q1",86.0]
Q5
## ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6, "Q5",86.0 ]
To delete an element in a list, simply use del().
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
del(maths[2:])
maths
## ["Q1",89.5]
6. How to sort a list
Python lists have a built-in sort() method to modify the list in place and a sorted() function to build a new sorted list from an iterable.
List.sort()
ls = [5, 2, 3, 1, 4]
ls.sort()
ls
[1, 2, 3, 4, 5]
sorted()
ls = [5, 2, 3, 1, 4]
sorted(ls)
[1, 2, 3, 4, 5]
Python Dictionaries
In Python, a dictionary is an unordered mapping of keys matching values. This dictionary can be used for later reference, just like a real dictionary.
Create a Dictionary in Python3
A Python dictionary is created using curly brackets {}
.
dictionary = {'key': 'value'}
seo_dict = {
"SEO":"Search Engine Optimization",
"SEM":"Search Engine Marketing",
"NumPy":"Numerical Python",
"SERP":"Search Engine Result Page",
"JS":"JavaScript",
"ccTLD":"country code top-level domain",
"DA":"Domain Authority",
"HTML":"Hypertext Markup Language",
"HTTPS":"Hypertext Transfer Protocol",
"PPC":"Pay Per Click"
}
seo_dict["HTTPS"]
## "Hypertext Transfer Protocol"
Update Dictionaries
In our previous example, there is an error. HTTPs doesn’t stand for “Hypertext Transfer Protocol”, but for “Hypertext Transfer Protocol Secure”.
To modify an entry, you can replace it simply by selecting the element using the square brackets [].
seo_dict
##{'SEO': 'Search Engine Optimization','SEM': 'Search Engine Marketing','NumPy': 'Numerical Python','SERP': 'Search Engine Result Page','JS': 'JavaScript','ccTLD': 'country code top-level domain','DA': 'Domain Authority','HTML': 'Hypertext Markup Language','HTTPS': 'Hypertext Transfer Protocol','PPC': 'Pay Per Click'}
#Select the element
seo_dict["HTTPS"]
## "Hypertext Transfer Protocol"
#Select the element and replace the value.
seo_dict["HTTPS"] = "Hypertext Transfer Protocol Secure"
seo_dict["HTTPS"]
## "Hypertext Transfer Protocol Secure"
You could also replace a value by using the .update
method.
seo_dict = {
"SEO":"Search Engine Optimization",
"SEM":"Search Engine Marketing",
"NumPy":"Numerical Python",
"HTTPS":"Hypertext Transfer Protocol"
}
#Update the value
seo_dict.update({"SEO":"Search Engine Optimization","SEM":"Search Engine Marketing","NumPy":"Numerical Python","HTTPS":"Hypertext Transfer Protocol Secure"})
#Result
seo_dict["HTTPS"]
##'Hypertext Transfer Protocol Secure'
To remove a value, use .pop()
.
seo_dict.pop("HTTPS")
seo_dict
##{'SEO': 'Search Engine Optimization','SEM': 'Search Engine Marketing','NumPy': 'Numerical Python'}
Conditions (If/Elif/Else)
Programming was originally base on a simple idea. If this happens, then do this, if not do that. This is what we call a boolean operation that is used in all programming languages. Everything can be coded with bits of zeros and ones, hence the weird code we often see “10011…”.
To code these booleans in Python, we use the if
, elif
and else
conditions.
If Statement
Let’s see how that works. This condition can be read like this: “If this is true, then do this”.
When a condition is true:
x = 200
y = 155
if x > y:
print("x is greater than y")
## x is greater than y
When a condition is false:
x = 200
y = 255
if x > y:
print("x is greater than y")
## Error
When you make a program, you want to remove those errors by completing your code. Here comes the elif
statement.
Elif Statement
The elif
statement goes like this: “if the previous conditions were not true, then try this condition”.
When a condition is True:
x = 200
y = 200
if x > y:
print("x is greater than y")
elif x == y:
print("x and y are equal")
## x and y are equal
When a condition is False:
x = 200
y = 255
if x > y:
print("x is greater than y")
elif x == y:
print("x and y are equal")
## Error
See.
We still get an error. We are going to close the loop using the else
statement.
Else Statement
The else
statement goes like this: “if the if
condition is false, and all the elif
conditions are also false, then do this condition”.
x = 200
y = 255
if x > y:
print("x is greater than y")
elif x == y:
print("x and y are equal")
else:
print("x is greater than y")
## x is greater than y
Ternary Operators. Perform and If/Else on a Single Line
The ternary operator is a simple way to convert an if / else conditional statement into a one-liner.
condition_if_true if condition else contition_if_false
Let’s take our earlier example.
x, y = 200, 255
"x and y are equal" if x==y else "x and y are not equal"
## 'x and y are not equal'
Nested Ternary Operator
You can create nested ternary operators using parentheses ()
.
z = 200
"Less than 10" if z<=10 else ("11-100" if z<=100
else ("101-500" if z<=500 else "Greater than 500"))
##"101-500"
Python Built-In Functions
Now, let’s look at basic Python functions.
What Are Python Functions?
A function in Python is a block of reusable code that is used to perform a single specific task, and only runs when it is called.
How to Use Python Functions?
To use Python function, use call the function by its name making sure to add the parentheses to the function name and then add the required arguments for that function.
print('argument of the print() function')
Basic Python Functions
Python has a number of built-in functions.
print()
sum()
len()
input()
type()
Print()
The print()
function lets you print a specific result on a screen.
x = "I love SEO"
print(x)
## I Love SEO
Sum() and Len()
When we want to calculate the average of our maths results, we could have passed the variable “maths” to the function sum()
, that added the values, and to the len()
function, that returns the length of the string.
maths = [89.5,92.1,32.5,75.6]
mathsavg = sum(maths)/len(maths)
print(mathsavg)
##72.425
##This is equivalent to
##mathsavg =(maths[0]+maths[1]+maths[2]+maths[3])/4
Input()
The input()
function in Python shows a message to the user and prompts him to do an action.
user_answer = input("What is your favorite SEO programming language?")
#Message in the console
What is your favorite SEO programming language?
At this point, the console will wait for the user to answer before running the code.
Type()
The type()
function simply let you know what is the type of a selected variable.
type("data science is fun!")
##str
Get Help
To see how a function works, all you have to do is to call the function using help()
or ?
before the function in IPython.
help(len)
?len
#Help on built-in function len in module builtins:
#len(obj, /)
# Return the number of items in a container.
Full List of Python Built-in Functions
- abs()
- aiter()
- all()
- any()
- anext()
- ascii()
- bin()
- bool()
- breakpoint()
- bytearray()
- bytes()
- callable()
- chr()
- classmethod()
- compile()
- complex()
- delattr()
- dict()
- dir()
- divmod()
- enumerate()
- eval()
- exec()
- filter()
- float()
- format()
- frozenset()
- getattr()
- globals()
- hasattr()
- hash()
- help()
- hex()
- id()
- input()
- int()
- isinstance()
- issubclass()
- iter()
- len()
- list()
- locals()
- map()
- max()
- memoryview()
- min()
- next()
- object()
- oct()
- open()
- ord()
- pow()
- print()
- property()
- range()
- repr()
- reversed()
- round()
- set()
- setattr()
- slice()
- sorted()
- staticmethod()
- str()
- sum()
- super()
- tuple()
- type()
- vars()
- zip()
- import()
User-Defined Functions
A function has four parts: the def statement, the function name, your arguments passed in the parameters, the program statement.
# The part where you pass your arguments is known as "parameters"
def yourFunction(arg1, arg2, arg3):
## Pass your function's statements
statement1
statement2
statement3
##End Function
return;
#Call the Function
yourFunction()
Here is a great video by Datacamp to understand the basics to define your own function.
How to Create Python Functions?
To create python functions, use the def keyword, along with the function name, the arguments and the program statement in this format:
def fun_name(arguments):
# program statement
All we need to do is:
- Define your function using Def
- Give the program statement
- End your function
- Call your function
- Pass your arguments to your
function()
Define the Function
To define a function in Python, you need to use the def
keyword.
def myPythonFunction():
Give the Program a Statement
def myPythonFunction():
#statement
y = 3*4
print(y)
End the Function
To end a function, you can use the return
statement.
Note that return
does not print out the value, it returns when the function is called.
def myPythonFunction():
y = 3*4
print(y)
return; #End Function
#If you run it this way nothing happens.
Call The Python Function
To call the Python function that you have defined, just add the name of the function after the return statement.
def myPythonFunction():
y = 3*4
print(y)
return;
myPythonFunction()
##12
Pass Arguments to the Function Parameters
See that we have not passed any arguments to the parameter of the function… So far.
What if we don’t know the first value yet?
def myPythonFunction(x):
y = x*4
print(y)
return;
myPythonFunction(5)
##20
Python Methods
A method in python is similar to a function, except it is associated with an object or a class. Depending on the type of an object, the available methods are different.
Methods are functions that belong to Python objects.
The two major differences between methods and functions in python are:
- The method is called on an object (like str, float or int) and is dependant on that object.
- The method can operate on the data that is contained within the class.
How to Use Methods in Python?
There are a lot of built-in methods that you can use. Remember, a method is dependant to an object. So, to use a method, you need to use dot (.
) notation.
object.method(arguments)
Example
In this example, we have an object of type “list”. Thus, we need to find methods that can be applied to a list.
Then, we need to call the object, followed by a dot (.
) and the name of the method.
maths = ["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6]
maths.insert(8,"Q5")
maths.insert(9,78.9)
print(maths)
##["Q1",89.5,"Q2",92.1,"Q3",32.5,"Q4",75.6,"Q5",78.9]
Useful methods
index()
: returns the index of the 1st element of a list that matches the argument.count()
: counts the number of times an element occurs in a list.replace()
: searches a string for a specified value (or Regex), and replace the specified values with the ones provided.sort()
: sorts the elements of an array in place and returns the sorted array.remove()
: Removes the first element from a string that matches the specified arguments.append()
: Adds a specified element to a string.upper()
: Capitalize all letters.lower()
: Convert all letters to lowercase.split()
: Split a string into several elements.is_integer()
: Find if a number is an integer or not.
Python Packages
Packages are really helpful because they are directories of Python Scripts that specify functions and methods so you can make advanced operations without even understanding what is under the hood.
To use a package, you need to:
- Install the package
- Import the library
- Learn how to use the package
What are the Most Commonly Use Python Packages?
For all practical uses, there are more Python packages that you can use. So, where to start?
Some of the most popular Python libraries are:
Python Package Name | How is it used |
---|---|
Pandas | Data analysis and manipulation |
NumPy | Array processing |
Matplotlib | Data visualization |
Seaborn | Data visualization |
Requests | HTTP requests |
Scikit-learn | Machine learning library |
Keras | Machine learning library |
Tensorflow | Machine learning library |
re | Regular expression matching operation |
datetime | Manipulates dates and times |
csv | Manipulates CSV files |
random | Pseudo-random number generators |
json | Manipulates JSON files |
argparse | Interacts with command-line |
multiprocessing | Enables to run multiple processes in parallel |
os | Uses the operating systems’ functionalities |
threading | Enables to run multiple processes in parallel |
urllib | Breaks URL strings up into components |
sqlite3 | Allows interactions with a database of the SQL query language |
collections | Specialized container datatypes |
pickle | Implements binary protocols for serializing and de-serializing a Python object structure |
time | Provides time-related functions |
1. How to Install Python Packages
To install Python packages, you need to have a package manager such as pip
or conda
installed in your working environment and use that package manager to installed the required packages.
If you have installed Python using Anaconda, you might already have installed some of the packages you want. Both conda
and pip
are installed natively when you install Python using Anaconda.
Install Python Packages Using Pip
To install Python packages with pip, use the pip install
command using either pip
or pip3
keyword depending on the version of pip
installed on your environment.
pip3 install scipy
It is good practice to verify if the package is already installed prior the installation process. Do so with the pip show
command.
pip3 show scipy
Here are a few tutorials too learn how to use pip to install Python packages.
- How to use pip in Python (Install, Update, Uninstall Packages)
- Pip install requirements.txt in Python (Install Packages)
- How to List Installed Python Packages (with/without Pip)
Install Python Packages Using Conda
To install Python packages using conda
, use the conda install
command in your Anaconda prompt.
conda install scipy
It is good practice to verify if the package is already install prior the installation process. Do so with the conda search
command.
conda search scipy
2. Import Python Packages
Before you can start using a package, you need to import the package. You can do this either in your Python Script or straight into the IPython Shell using the “import” command.
import pandas
Alternatively, you could import a package using a shortened name to make it faster to use your code later. To do this, use the “as” command.
import pandas as pd
3. How to Use The Package?
So, to call a function for a package, you need to call the package first (as we have done earlier calling a variable), and then call the function.
package.function(variable)
Example
A common operation that you’ll need is to import a CSV. To do this, there is a function in the pandas package called read_csv().
If you try to use only read.csv() to call your file, you’ll end-up with an error. Instead, call the package first with one of these appellations.
import pandas
pandas.read_csv("youfile.csv")
Or, use the package under a different name.
import pandas as pd
pd.read_csv("youfile.csv")
For, While Loops
You might want at some point to create action over and over without manually typing the function all the time.
The python loops will help you repeat the action until a condition is met.
This is what we call an iteration.
Python has two native loop commands:
while
loopsfor
loops
Python While Loops
The while
loop in Python states that you need to execute a set of instructions for as long as the condition is True
.
#What user input
x = 3
#Conditions
while x < 10:
print("I love SEO")
#Increase by 1 at each iterations
x = x+1
##I love SEO
##I love SEO
##I love SEO
##I love SEO
##I love SEO
##I love SEO
##I love SEO
Python For Loops
When you know in advance the number of iteration your loop should perform, you can use the favor for
loop over the while
loop.
Let’s say that you deeply love learning python for SEO and you want to shout it out three times.
To do this, use a for
loop.
for x in range(3):
print("I Love Using Python for SEO")
##I Love Using Python for SEO
##I Love Using Python for SEO
##I Love Using Python for SEO
Python Errors and Exceptions
There are two different kinds of errors in Python: syntax errors and exceptions.
Syntax Errors
Syntax errors are parsing errors that are raised when something does not follow the required Python syntax, such as indentation for instance.
Exceptions
Python Exceptions are errors detected during the execution even when the syntax is correct. Examples of exceptions are the ZeroDivisionError, the TypeError and the NameError.
How to Handle Python Exceptions
To handle Python exceptions, use the try
and except
keywords to surround your Python code.
try:
1 / 0
except:
print('error')
error
Python Features
Python is a programming language that supports object-oriented programming, structured programming (if/then) and functional programming.
Python Standard Library
Python has a standard library that contains built-in modules that provide access to system functionalities and is often with the Python installers.
The Python standard library has built-in:
- Built-in functions (print(), range(), etc.)
- Built-in constants (True, False, etc.)
- Built-in Types (numeric, boolean, iterators, etc.)
- Built-in Exceptions (ImportError, ModuleNotFoundError, etc.)
It also has other features such as:
- Defined data types (datetime, array, etc.)
- Text processing services: (
re
andstring
modules, etc.) - Mathematical modules: (random, math, etc.)
- Functional programming tools (itertools, functools)
- File access modules (pathlib, os, shutil, etc.)
- Data persistence modules (pickle, gzip, etc.)
- File formats (csv, json, etc.)
- and much more.
Python Functional Programming
Functional programming decomposes a problem into functions that take input and produce output.
Example of functional programming in Python
# Define a function that takes an argument and returns its square
def square(x):
return x ** 2
# Use the map function to apply the square function to a list of numbers
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(square, numbers))
# Print the result
print(squared_numbers)
Output
[1, 4, 9, 16, 25]
In this is example of functional programming, we define a function (e.g. square
function) and we use another function (e.g. map
) to apply the function to a list of numbers.
Python Object-Oriented Programming (OOP)
Object-oriented programming decomposes a problem into objects instead of functions and logics.
Example of Object-Oriented Programming in Python
# Define a class called Person
class Person:
# Define a constructor that sets the name and age of the person
def __init__(self, name, age):
self.name = name
self.age = age
# Define a method that prints the name and age of the person
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# Create an instance of the Person class
person = Person("Jean-Christophe", 32)
# Call the the method on the object
person.say_hello()
Output
Hello, my name is Jean-Christophe and I am 32 years old.
In this is example of object-oriented programming, we have defined a class with properties and methods. The class (e.g. Person) is used to create an instance of object on which we can call the methods (e.g. say_hello()).
What is a Python Class
A class in Python is a way used in object-oriented programming to combine data and functionality to organize Python code.
There are two operations supported by Python classes: attribute references and instantiation.
The instantiation uses the function notation to create a class object:
obj = MyClass()
The attribute reference follow the conventional Python syntax to access attributes of the class.
obj.attributename
Method objects can be called on the Class object.
obj.my_method()
The Zen of Python
The Zen of Python was written by Tim Peters and represents the core philosophy of Python programming.
To view the zen of Python, import this package.
import this
The Zen of Python, by Tim Peters
- Beautiful is better than ugly.
- Explicit is better than implicit.
- Simple is better than complex.
- Complex is better than complicated.
- Flat is better than nested.
- Sparse is better than dense.
- Readability counts.
- Special cases aren’t special enough to break the rules.
- Although practicality beats purity.
- Errors should never pass silently.
- Unless explicitly silenced. In the face of ambiguity, refuse the temptation to guess.
- There should be one– and preferably only one –obvious way to do it. Although that way may not be obvious at first unless you’re Dutch.
- Now is better than never.
- Although never is often better than *right* now.
- If the implementation is hard to explain, it’s a bad idea.
- If the implementation is easy to explain, it may be a good idea.
- Namespaces are one honking great idea — let’s do more of those!
History of Python
Python, was created by Guido van Rossum in the late 1980s and succeeded the ABC programming language. It gained features like exception handling.
- Python 2.0 (2000) introduced list comprehensions and Unicode support,
- Python 3.0 (2008) backported major features to Python 2.6.x and 2.7.x.
- Python 3.7 and later versions are currently supported.
- Recent expedited releases, such as Python 3.10.4 and 3.9.12, addressed security issues,
- Python 3.11, released in November 2022, brought improvements in execution speed and error reporting.
Why Use Python for Data Science
Python programming is one of the two most popular programming languages for data science: Python and R. Python is general-purpose programming language that is used not only by data scientists, but also software developers. This quality makes it a prime candidate as it allows data science work to be more easily integrated into the Software engineering infrastructure. Python offers various libraries that can be used by data scientists in their workflow such as NumPy, Pandas, Matplotlib, Seabon and Statsmodels. Most data science programs will start with some introduction to a programming language.
Why Use Python in Machine Learning
Python should be used in machine learning projects for three reasons:
- it is easily integrable with softwares,
- it has a large community and finding support is easy
- it has extensive series of Machine Learning libraries such as Scikit-learn, spaCy, NLTK, SciPy, PyTorch and Tensorflow.
Python Programming Examples
- How to Use VSCode with Python
- Python Libraries For Beginners (Pandas, NumPy, Matplotlib and more…)
- Web Scraping with Python Requests
- How to Use Selenium With Python
- Python Script Automation Using Task Scheduler (windows)
- Python Script Automation Using CRON (Mac)
- Reddit API with Python
- Linkedin API with Python
- Facebook Graph API with Python
- Deploy a Flask App on Heroku
- Get Started With Django
- Create Feature Image With Python (Pillow)
- Read RSS Feed with Python and Beautiful Soup
- Uncompress Multiple GZip files with Python
- How to Use Anaconda Environments
- if __name__ == ‘__main__’: What does it mean (Python)
- Create and Run a Python Script Using the Command-line Only
Python Programming FAQs
What is Python?
?How to install Python?
⭐What are the Best Python Tools?
❤️What should you learn first?
Conclusion
At last, you now understand everything you need to get started using Python for SEO and Data Science. Congratulations, you completed this absolute guide for Python Beginners.
In order to learn Python for SEO, you need to understand why you should learn Python in the first place. Then, you need to cover the basics.
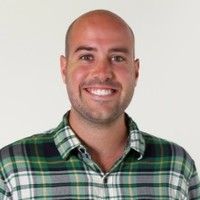
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.