If you use the pip
package manager to install and manage your Python libraries, you can use the requirements.txt
configuration file to specify all the packages, and their versions, to be installed for a Python project.
Follow this article for those interested in learning how to use pip to install and manage Python packages. For those interested in how to use the command prompt.
What are the Requirements Files
The requirements configuration files are files that contain a list of items to be installed when using pip.
The requirements.txt files looks something like this:
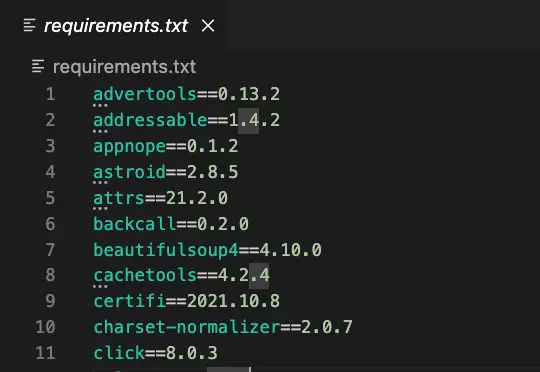
Install Python Package (pip install -r requirements.txt)
To install Python packages using the requirements.txt configuration file, use the pip install -r requirements.txt
command:
$ pip install -r requirements.txt
Although not obligatory, the convention is to name the requirements file requirements.txt
.
If the requirements.txt
file is not in your working directory, specify the path instead:
$ pip install -r path/to/requirements.txt
What is the -r Flag in pip install -r requirements.txt
In the pip install -r requirements.txt
command, the -r
flag allows pip install
to open the requirements.txt file and install the packages inside of it.
How to Write your requirements.txt Configuration File
The requirements.txt
Configuration File is structured in a way that each line of the requirements file represents something to be installed.
- Lines starting with a
#
are ignored. - A package can be specified with its name only
- A package can be specified with the name and the version
- A package can have options
- A package can have multiple conditions
# this is a comment line
# Requirements without Version
nose
nose-cov
beautifulsoup4
# Requirements with Version
docopt == 0.6.1
# Requirements with conditions
requests [security] >= 2.8.1, == 2.8.* ; python_version < "2.7"
Check out the official doc on the requirements.txt file formats.
Requirements.txt Version Specifiers
You can specify the version of a package using one of the following specifiers ==
, >
, >=
, <
, <=
, ~=, !=
described in full on PEP 508.
Here is a few examples of valid requirements.txt
specifiers.
package
package == 1.3
package >= 1.2, < 2.0
package[foo, bar]
package ~= 1.4.2
package == 5.4 ; python_version < '3.8'
package ; sys_platform == 'win32'
requests [security] >= 2.8.1, == 2.8.* ; python_version < "2.7"
Check out the official doc on the requirements.txt specifiers.
Specify Two Versions of a Package in Requirements.txt
You can specify two versions of a package in requirements.txt
by using the comma with the requirements specifiers.
package >= 1.2, < 2.0
In the example above, pip
will select the best match that is at least 1.2 and less than 2.0.
Export Python Environment Configuration (pip freeze)
To export all the installed packages of your current Python environment, along with their versions, use the pip freeze
command.
The pip freeze command is used to output the installed packages and their version.
$ pip freeze
Everything listed here can be added to the requirements.txt
file by using the pip freeze > requirements.txt command
.
advertools==0.13.2
pandas==2.0.1
Read the following article to learn more about how to list installed packages in Python.
pip freeze > requirements.txt
You can use pip freeze
to create a requirements.txt
file that can be used to install a series of packages in one go.
$ pip freeze > requirements.txt
Then, all the packages listed in the requirements.txt file will be accessible for installation using pip install -r requirements.txt
.
Official Pip Documentation
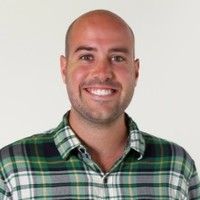
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.