In this guide, I will show you how to use Python and the Facebook Graph API to post to Facebook Groups.
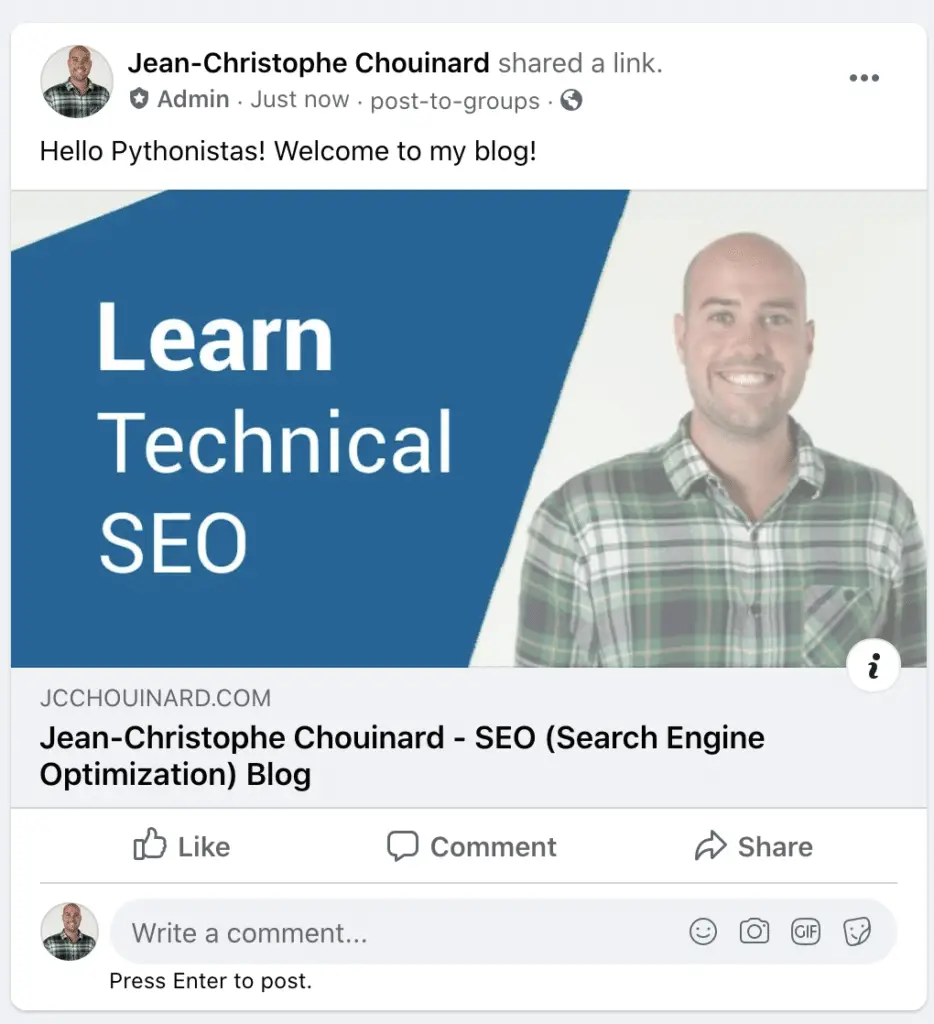
This tutorial is very simple and will give you the basics to learn how to use the Facebook API with Python.
If you know nothing about Python, you should start by reading my complete guide on Python for SEO.
Let’s get going, shall we?
What is an API?
An Application Programming Interface, or API, is a method of communication in which applications give access to their data in a structured way, without the need of interacting with the user interface.
Read This Before Posting to Facebook Groups
I created a Facebook group specifically for this tutorial so you can try the API without spamming some random group.
Please, please stick to posting in that Facebook group!
You are allowed to post in that group! Please do.
https://www.facebook.com/groups/744128789503859
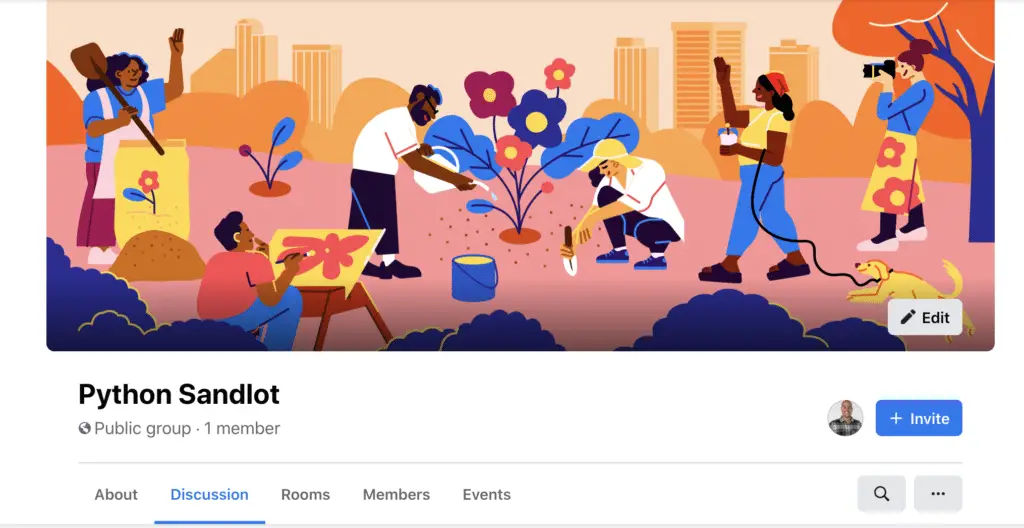
Let’s look at the steps to post to a Facebook Group using the Graph API.
1. Create a Facebook API App
The first step is to create an App in the Facebook Developers Console and get an access token.
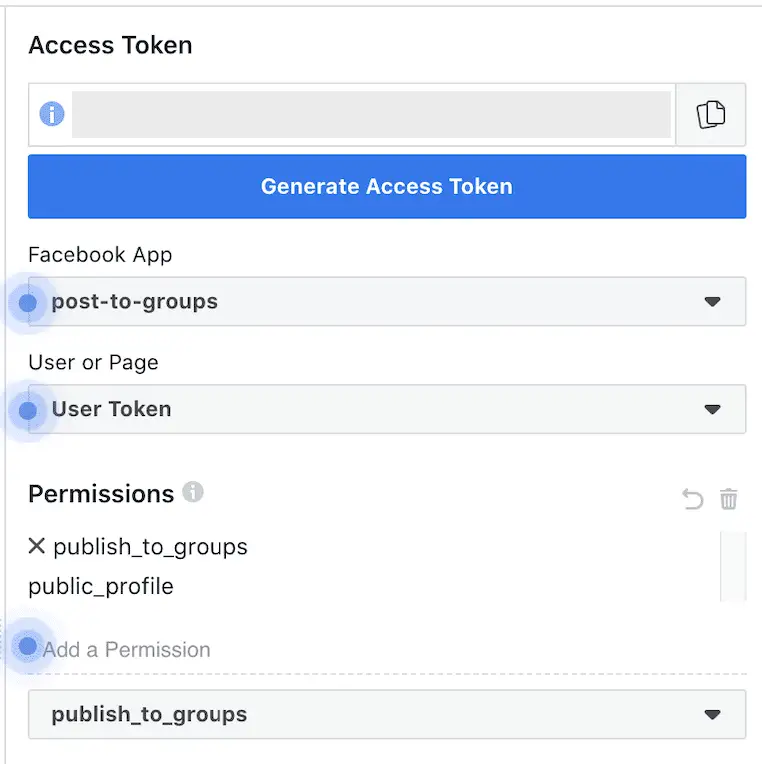
If you don’t know how, just check this guide on how to get Facebook Graph API access token.
2. Save Your Credentials
Now, you will need to add your access token to a credentials.json
file.
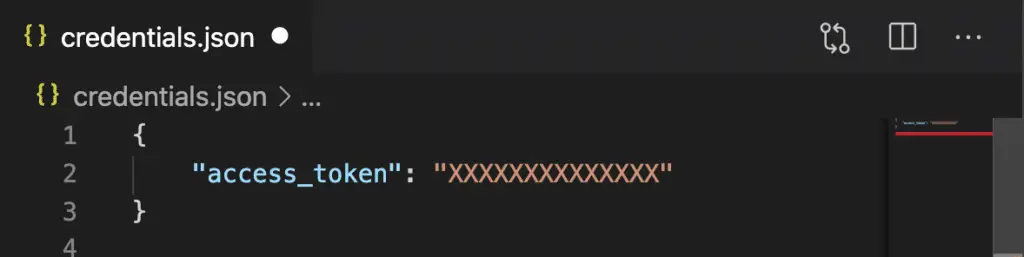
3. Read the credentials
Now, let’s create a function to read the credentials file.
import json
def read_creds(filename):
'''
Store API credentials in a safe place.
If you use Git, make sure to add the file to .gitignore
'''
with open(filename) as f:
credentials = json.load(f)
return credentials
credentials = read_creds('credentials.json')
4. Authorize the App
from facebook import GraphAPI
graph = GraphAPI(access_token=credentials['access_token'])
5. Post Your Message to All Groups
Here add your groups ID.
The group ID can be found in the URL of the Group.

message = '''
Read the guide on how to post to Facebook groups using the Facebook API.
'''
link = 'https://www.jcchouinard.com/post-to-groups-using-facebook-graph-api-python/'
groups = ['744128789503859']
for group in groups:
graph.put_object(group,'feed', message=message,link=link)
print(graph.get_connections(group, 'feed'))
Full Code
import json
from facebook import GraphAPI
def read_creds(filename):
'''
Store API credentials in a safe place.
If you use Git, make sure to add the file to .gitignore
'''
with open(filename) as f:
credentials = json.load(f)
return credentials
credentials = read_creds('credentials.json')
graph = GraphAPI(access_token=credentials['access_token'])
message = '''
Add your message here.
'''
link = 'https://www.jcchouinard.com/'
groups = ['744128789503859']
for group in groups:
graph.put_object(group,'feed', message=message,link=link)
print(graph.get_connections(group, 'feed'))
This is it!
You now know how to post to Facebook groups using the Graph API and Python.
If you want more Social Media Automation tutorials, be sure to read the complete guides on the Reddit API and the Linkedin API with Python.
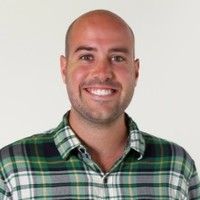
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.