The teleport API allows to gather data about a geographical areas for free.
Teleport allows you to gather data from cities such as:
- Population
- Geographical area
- Salaries
- Job market
- Cost of living
- Images
- and more
In this tutorial, we will learn how to use the teleport API to gather geographical data.
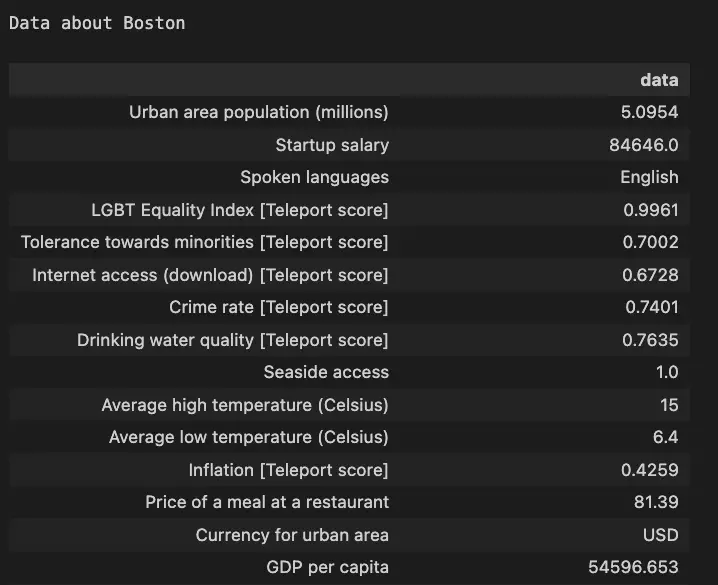
What is the Teleport API
The teleport API is an open-source application programming interface that allows you to gather data from geographical regions.
Using The Teleport API with Python
Information about the API can be found on the official Teleport API Documentation.
How the API works?
The Transport API is an open API for which you can simply call the endpoint.
For instance, if you open the following URL in your browser, you will see that no authentication is required to use the API.
https://api.teleport.org/api/urban_areas/slug:boston/
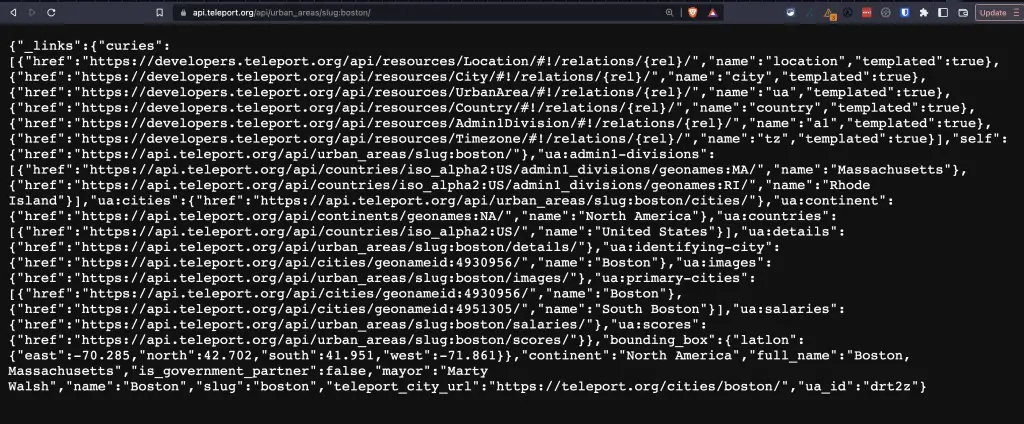
In order to use the API with Python, we simply need to use the Python Requests library.
Create the Functions to Get the Data
import requests
def get_teleport_data(city_name):
ua_endpoint = get_urban_area_endpoint(city_name)
results = {}
if ua_endpoint:
details = get_area_details(ua_endpoint)
results['details'] = details
images = get_area_images(ua_endpoint)
results['images'] = images
scores = get_area_scores(ua_endpoint)
results['scores'] = scores
return results
def get_urban_area_endpoint(city_name):
try:
cities_endpoint = 'https://api.teleport.org/api/cities/'
url = f'{cities_endpoint}?search={city_name}'
r = requests.get(url)
r = r.json()
r['_embedded']
geoname_url = r['_embedded']['city:search-results'][0]['_links']['city:item']['href']
r2 = requests.get(geoname_url)
r2 = r2.json()
ua_endpoint = r2['_links']['city:urban_area']['href']
return ua_endpoint
except:
return None
def get_area_details(ua_endpoint):
try:
r = requests.get(ua_endpoint + 'details/')
r = r.json()
result = {}
for cat in r['categories']:
result[cat['label']] = {}
for i in cat['data']:
name = i['label']
key = [k for k in i.keys() if 'value' in k][0]
score = i[key]
# result[name] = score
result[cat['label']][name] = score
return result
except:
return 'not_found'
def get_area_images(ua_endpoint):
try:
r = requests.get(ua_endpoint + 'images/')
r = r.json()
return r['photos']
except:
return 'not_found'
def get_area_scores(ua_endpoint):
try:
r = requests.get(ua_endpoint + 'scores/')
r = r.json()
result = {}
for i in r['categories']:
name = i['name']
score = i['score_out_of_10']
result[name] = score
return result
except:
return 'not_found'
Return the Result
city_name = 'Boston'
results = get_teleport_data(city_name)
Get Images for the City
results['images']
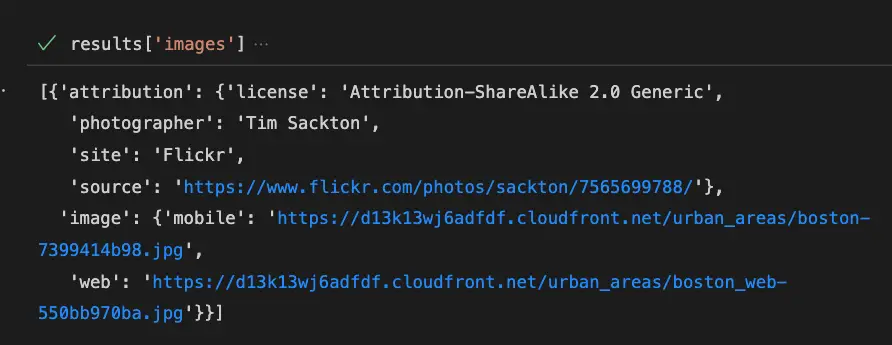
Show the City Scores
results['scores']
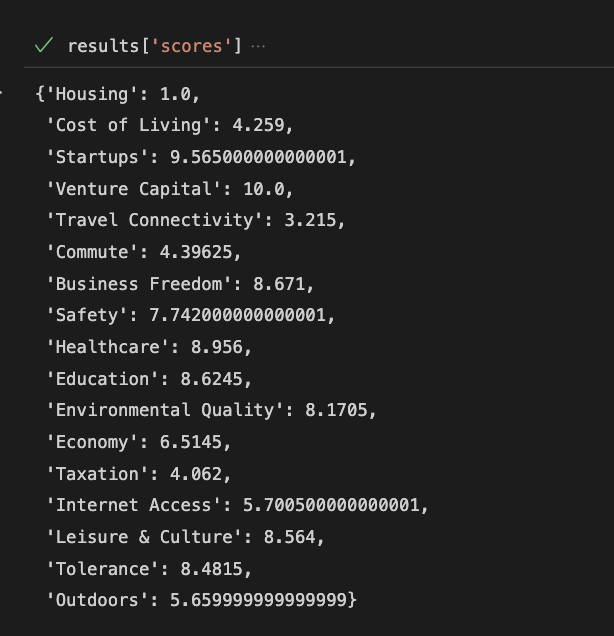
Conclusion
This is it, for more examples on how to use the Transport API with Python, just go to the official documentation website.
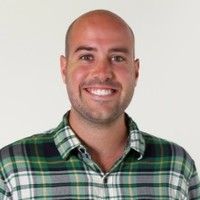
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.