Welcome to this tutorial where we will learn how to use the Flickr API with Python.
What is the Flickr API
Flickr has an API (short for Application Programming Interface) that allows to access Flickr data in a structured way, without the need of interacting with the user interface.
How to Get a Flickr API Key
In order to interact with the Flickr API, you will need to get the Flickr API Keys.
You can view the API keys as the username and password to the API.
To create your Flickr API keys, go to flickr.com/services/api/keys/.
- Create an Application
You will first need to create an application. Click on “create your first”. - Request an API Key
Then, make a request for an API key by clicking on “Request an API Key”
Then, click on “Request an API Key”.
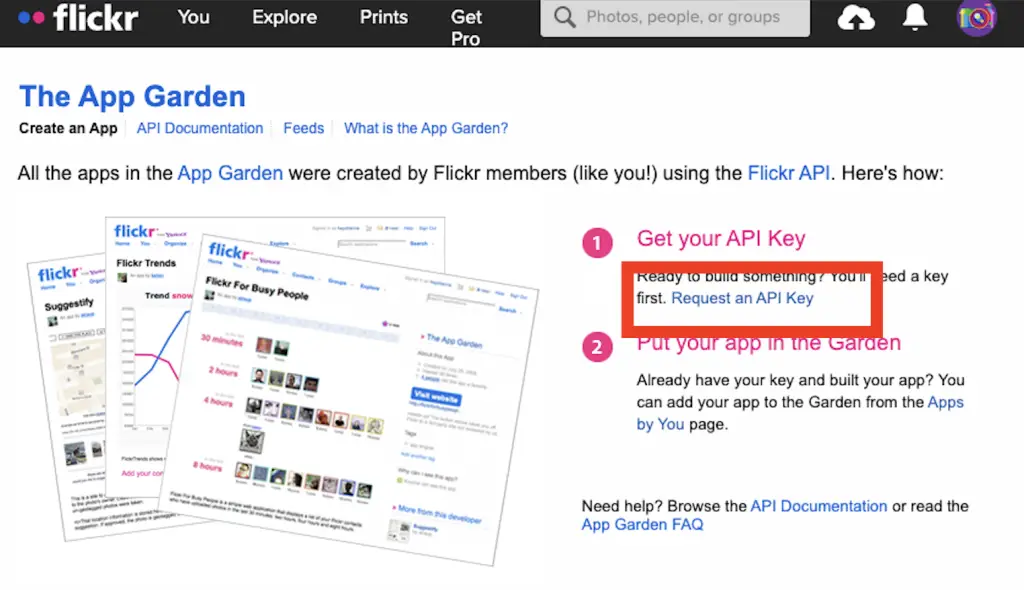
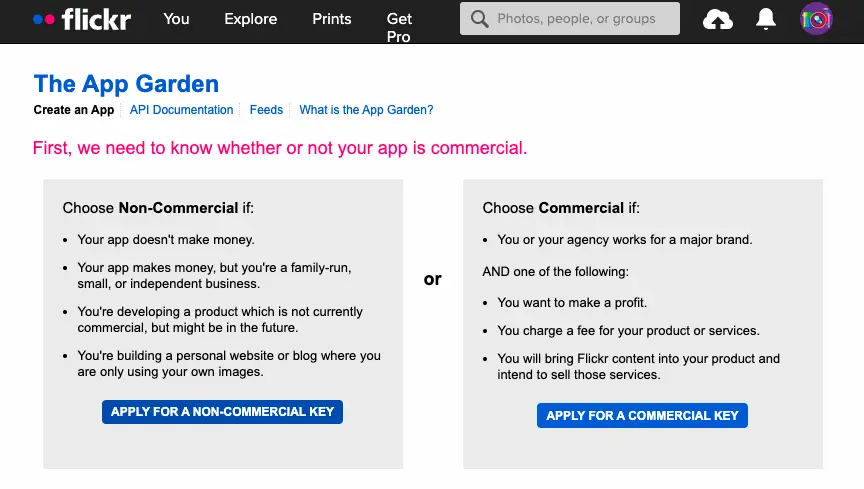
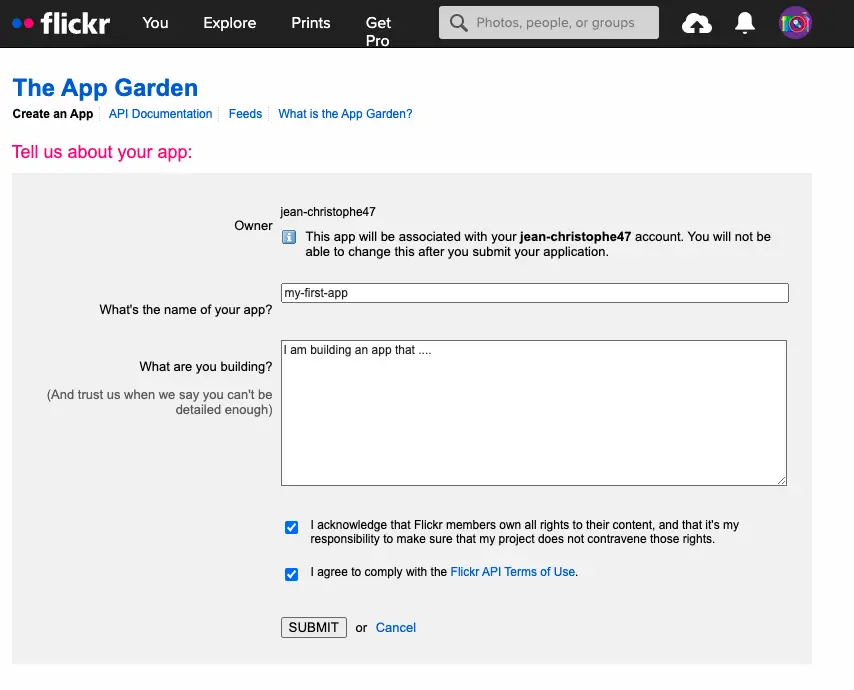
Flickr will create two API keys:
key
: A public key that can be viewed as the username of your applicationsecret
: A private key that can be viewed as the password of your application
Keep both in a safe place and do not share it to anyone.
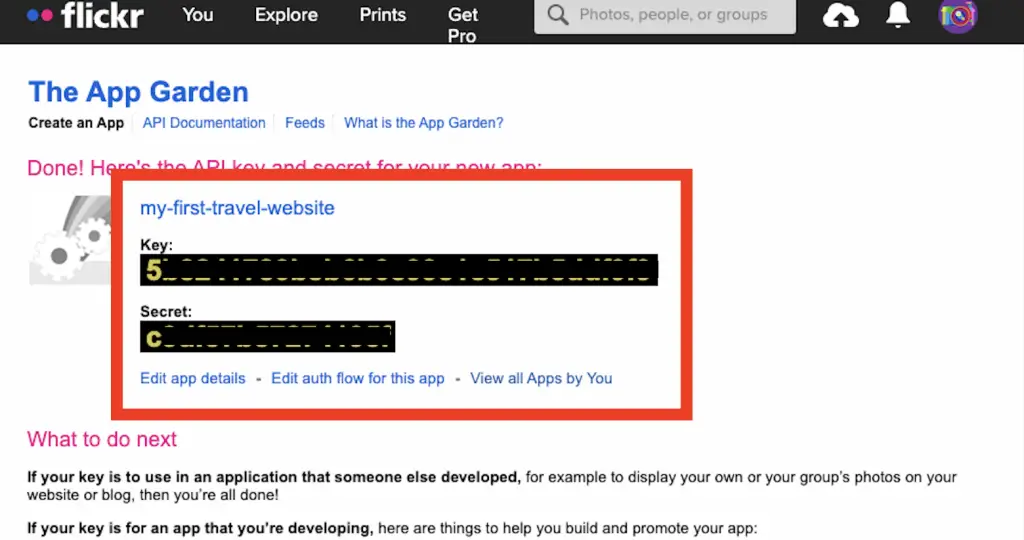
You can find your API keys by going to the App Garden and selecting “Apps by You” on the right-hand side.
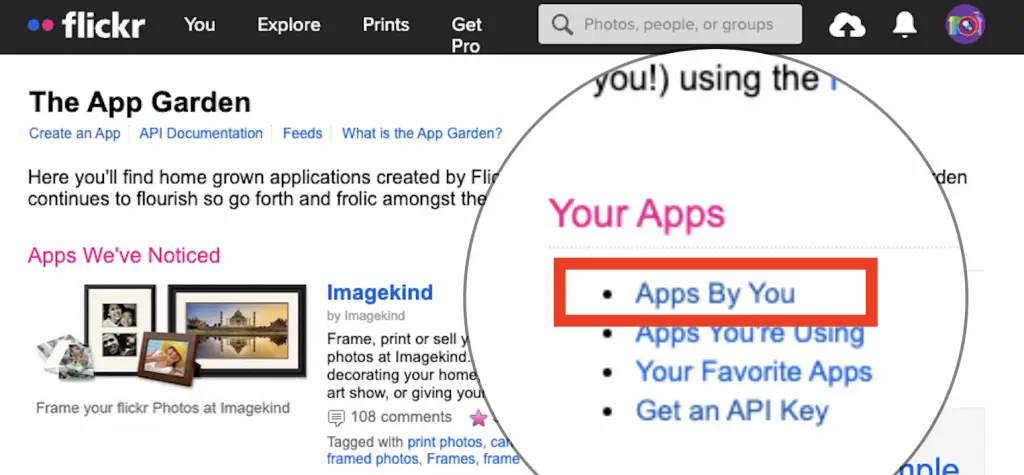
Install The Flickr API Python Library
$ pip install flickrapi
Store API Keys in a Separate File
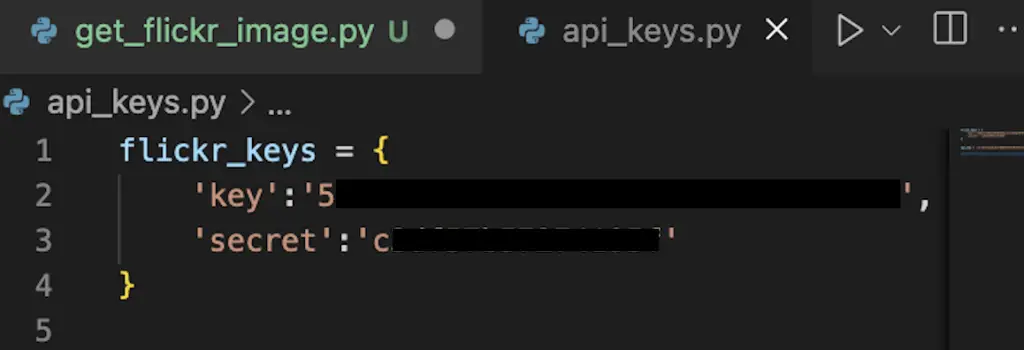
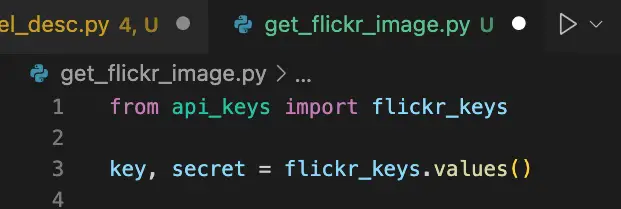
Full Python Code
from flickrapi import FlickrAPI
from api_keys import flickr_keys
key, secret = flickr_keys.values()
flickr = FlickrAPI(
key,
secret,
format='parsed-json'
)
# All the extra data that I want to have
extras='owner_name,license,geo,description,url_sq,url_t,url_s,url_q,url_m,url_n,url_z,url_c,url_l,url_o'
photos = flickr.photos.search(
text='Quebec City', # Search term
per_page=5, # Number of results per page
license='4,5,6,7,8,9,10', # Attribution Licenses
extras=extras,
privacy_filter=1, #public photos
safe_search=1 # is safe
)
Licenses
To know which licenses that you want, look here.
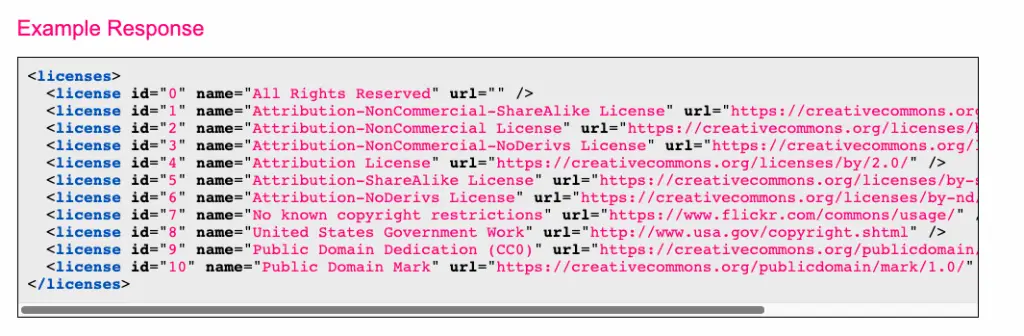
{'0': {'name': 'All Rights Reserved', 'url': ''},
'1': {'name': 'Attribution-NonCommercial-ShareAlike License',
'url': 'https://creativecommons.org/licenses/by-nc-sa/2.0/'},
'2': {'name': 'Attribution-NonCommercial License',
'url': 'https://creativecommons.org/licenses/by-nc/2.0/'},
'3': {'name': 'Attribution-NonCommercial-NoDerivs License',
'url': 'https://creativecommons.org/licenses/by-nc-nd/2.0/'},
'4': {'name': 'Attribution License',
'url': 'https://creativecommons.org/licenses/by/2.0/'},
'5': {'name': 'Attribution-ShareAlike License',
'url': 'https://creativecommons.org/licenses/by-sa/2.0/'},
'6': {'name': 'Attribution-NoDerivs License',
'url': 'https://creativecommons.org/licenses/by-nd/2.0/'},
'7': {'name': 'No known copyright restrictions',
'url': 'https://www.flickr.com/commons/usage/'},
'8': {'name': 'United States Government Work',
'url': 'http://www.usa.gov/copyright.shtml'},
'9': {'name': 'Public Domain Dedication (CC0)',
'url': 'https://creativecommons.org/publicdomain/zero/1.0/'},
'10': {'name': 'Public Domain Mark',
'url': 'https://creativecommons.org/publicdomain/mark/1.0/'}}
Photo Sizes
parameter | image size |
---|---|
url_o | Original size of image |
url_c | Medium-size image 800×800 |
url_q | Square image 150×150 |
url_s | Square image 75,75 |
url_t | Thumbnail image 100px on largest width |
url_n | Small image 320px on largest width |
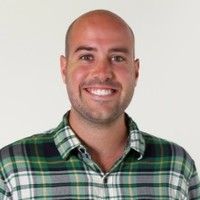
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.