In this tutorial, you will learn how to use the WordPress API with Python.
The WP API is an Application Programming Interface that allows you to interact with your WordPress’s database using your preferred programming language.
It allows you to automate WordPress publishing using Python.
Authenticate the API
keys = {
'wp_key': 'XXXX XXXX XXXX XXXX XXXX XXXX',
'user': 'username'
}
# Define your WP URL
url = 'https://www.example.com/wp-json/wp/v2'
# Define WP Keys
user = keys['wp_key']
password = keys['user']
# Create WP Connection
wp_connection = user + ':' + password
# Encode the connection of your website
token = base64.b64encode(wp_connection.encode())
# Prepare the header of our request
headers = {
'Authorization': 'Basic ' + token.decode('utf-8')
}
Get First 10 Posts
# Define Function to Call the Posts Endpoint
def get_posts(url, headers):
api_url = url + f'/posts'
response = requests.get(api_url, headers=headers)
return response.json()
# Call API
r = get_posts(url, headers)
# Print Number of Results Return
print(len(r))
# output: 10
# Show the First Result Tile
r[0]['title']
WordPress Posts with API
Update Post Categories
def update_post_categories(post_id, categories, headers=headers, url=url):
# If multiple categories
if isinstance(categories,list):
categories = ','.join(categories)
# Add values
args = {
'categories': categories
}
# Make Request
r = requests.post(
url + '/posts/' + str(post_id),
headers=headers, json=args)
return r.json()
Show Post Data
def show_blog_post(post_id, headers=headers, url=url):
r = requests.get(
url + f'/posts/{str(post_id)}',
headers=headers)
return r.json()
Work with WordPress Medias
Show Media Data
def show_media(media_id, headers=headers, url=url):
r = requests.get(
url + f'/media/{str(media_id)}',
headers=headers)
return r.json()
Update Media Caption
def update_media_caption(media_id, caption, headers=headers, url=url):
args = {
'caption': caption
}
r = requests.post(
url + '/media/' + str(media_id),
headers=headers, json=args)
return r.json()
Work with WordPress Categories
Show a Specific Category Data
def show_category(id, headers=headers, url=url):
response = requests.get(
url + f'/categories/{str(id)}',
headers=headers)
return response.json()
r = show_category(id, headers=headers, url=url)
Show All Categories
Show number of pages. There is a maximum of 100 element per page.
def get_total_cat_count(per_page=100, headers=headers, url=url):
api_url = url + f'/categories?per_page={per_page}'
response = requests.get(api_url, headers=headers)
page_count = response.headers['X-WP-TotalPages']
return int(page_count)
num_category_pages = get_total_cat_count()
Create function to read WordPress categories.
# Function to read wordpress categories
def read_wordpress_categories(page, per_page=100, headers=headers, url=url):
r = requests.get(url + f'/categories?page={page}&per_page={per_page}', headers=headers)
return r.json()
Loop through each page:
categories = []
for i in range(1,num_category_pages+1):
page_results = read_wordpress_categories(i)
categories.extend(page_results)
Delete a Category
def delete_category(id, headers=headers, url=url):
r = requests.delete(
url + f'/categories/{str(id)}?force=True',
headers=headers)
return r.json()
Delete All Categories
for category in categories:
id = category['id']
if str(id) != '1': # Skip Uncategorized
r_json = delete_category(id)
Conclusion
This is it, you now have learned my favourite WordPress automation commands with Python and WordPress REST API.
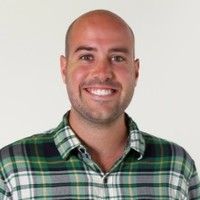
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.