This post is part of the Guide on Google Search Console API
In this tutorial, we will use Python and the sitemaps resource from the Google Search Console API to upload new sitemaps to Google Search Console.
Get Credentials
Google Search Console is a partner api. You’ll need to get credentials to gain access to it.
The first step is to store your credentials in a client_secrets.json
file. Check out how you can get Google Search Console OAuth Credentials.
Import OAuth Module
Then, you will need to create a oauth.py
file to handle the connection with the API. See “Authorise Requests to GSC API Using OAuth 2.0” blog post, for more details.
# oauth.py
import argparse
import httplib2
import requests
from collections import defaultdict
from dateutil import relativedelta
from googleapiclient.discovery import build
from oauth2client import client
from oauth2client import file
from oauth2client import tools
def authorize_creds(creds,authorizedcreds='authorizedcreds.dat'):
'''
Authorize credentials using OAuth2.
'''
print('Authorizing Creds')
# Variable parameter that controls the set of resources that the access token permits.
SCOPES = ['https://www.googleapis.com/auth/webmasters']
# Path to client_secrets.json file
CLIENT_SECRETS_PATH = creds
# Create a parser to be able to open browser for Authorization
parser = argparse.ArgumentParser(
formatter_class=argparse.RawDescriptionHelpFormatter,
parents=[tools.argparser])
flags = parser.parse_args([])
# Creates an authorization flow from a clientsecrets file.
# Will raise InvalidClientSecretsError for unknown types of Flows.
flow = client.flow_from_clientsecrets(
CLIENT_SECRETS_PATH, scope = SCOPES,
message = tools.message_if_missing(CLIENT_SECRETS_PATH))
# Prepare credentials and authorize HTTP
# If they exist, get them from the storage object
# credentials will get written back to the 'authorizedcreds.dat' file.
storage = file.Storage(authorizedcreds)
credentials = storage.get()
# If authenticated credentials don't exist, open Browser to authenticate
if credentials is None or credentials.invalid:
credentials = tools.run_flow(flow, storage, flags) # Add the valid creds to a variable
# Take the credentials and authorize them using httplib2
http = httplib2.Http() # Creates an HTTP client object to make the http request
http = credentials.authorize(http=http) # Sign each request from the HTTP client with the OAuth 2.0 access token
webmasters_service = build('searchconsole', 'v1', http=http)#build('webmasters', 'v3', http=http) # Construct a Resource to interact with the API using the Authorized HTTP Client.
print('Auth Successful')
return webmasters_service
# Create Function to execute your API Request
def execute_request(service, property_uri, request):
return service.searchanalytics().query(siteUrl=property_uri, body=request).execute()
if __name__ == '__main__':
creds = 'client_secrets.json'
webmasters_service = authorize_creds(creds)
Get a List of Sitemaps
Then, create a get_sitemap.py
file.
from oauth import authorize_creds, execute_request
creds = 'client_secrets.json' #path to the credentials file.
webmasters_service = authorize_creds(creds)
site = 'https://example.com/'
sitemap_list = ['sitemap1.xml','sitemap2.xml','sitemap3.xml']
print (f'Uploading sitemaps to {site}')
for sitemap in sitemap_list:
feedpath = site + sitemap
print(feedpath)
webmasters_service.sitemaps().submit(siteUrl=site, feedpath=feedpath).execute()
Debugging
Your sitemap is showing “Sitemap could not be read“.
Conclusion
The sitemaps resource is super useful for those who manage multiple sites, or large sites and are new to remove or upload multiple sitemaps at once.
Hope you managed to use this script to upload your sitemaps to Google Search Console with Python.
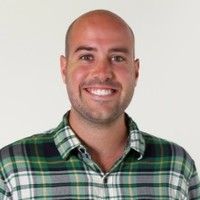
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.