To be able to use Selenium, you will need to install a browser and the browser driver. Selenium supports multiple web browsers, but since Chrome has by far the greatest market share, this tutorial will show you how to install the ChromeDriver.
There are two ways to install a web driver in Selenium:
- Manually
- In Python, with
webdriver_manager
(simplest)
Install Selenium Web Driver Manually
Here are the steps to install the Chrome Selenium web driver manually:
- Go to selenium.dev
- Clicks on Downloads next to Chromium/Chrome
- Select the version that matches your browser in chrome://settings/help
- Unzip the folder
- Run Selenium with Python
Step 1: To install Selenium Webdriver, just go to selenium.dev and go to “Third Party Browser Drivers” section.
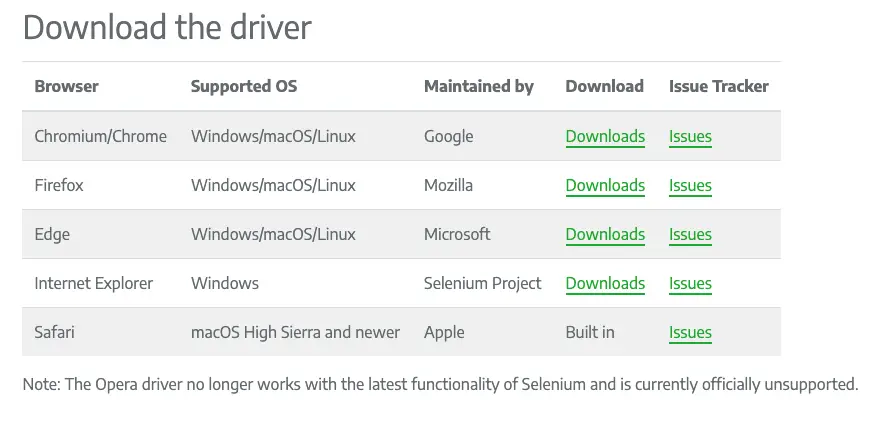
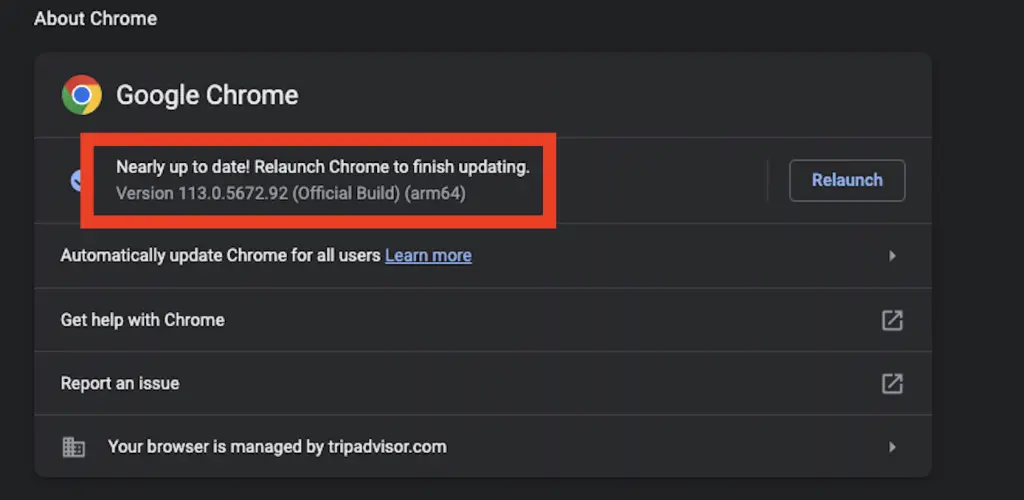
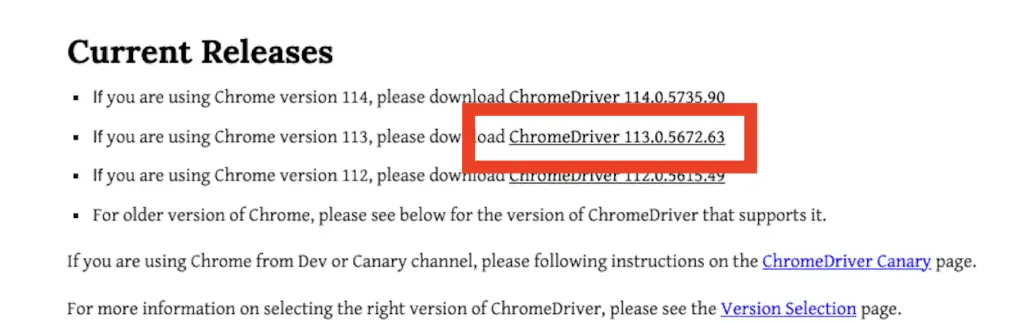
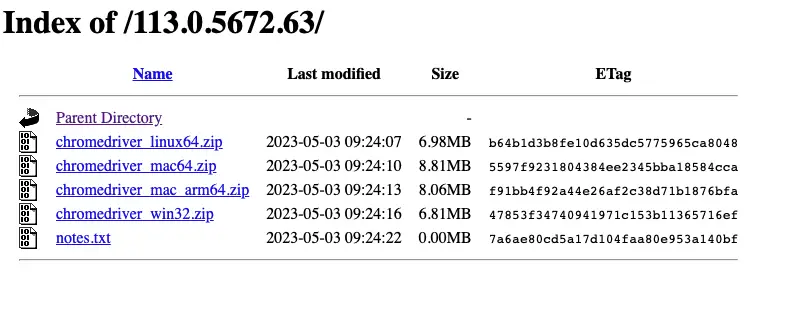
Once the above steps are done, open Python and import webdriver from Selenium.
from selenium import webdriver
If everything was properly installed, you should get something like this.

Next, we’ll set-up our browser.
After, you will need to find the executable path of your chrome browser.
To find it just chrome://version
in your browser.
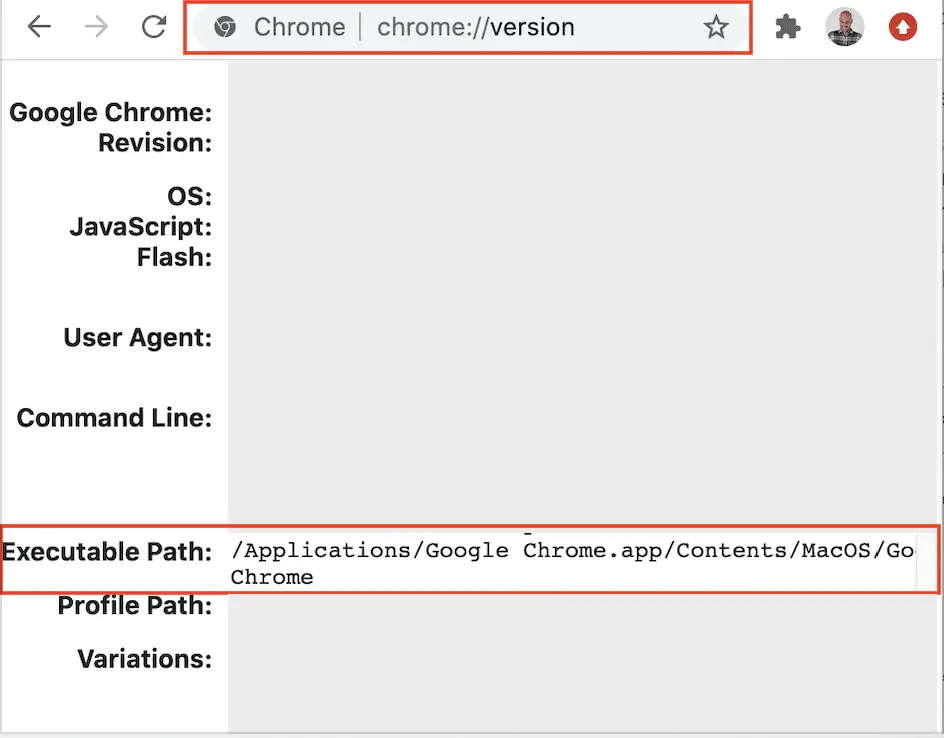
To run Selenium that was installed manually you will need to pass the executable path as the value of the webdriver.Chrome()
executable_path
keyword.
import time
from selenium import webdriver
# Define executable path
executable_path = r"C:\Users\j-c.chouinard\Downloads\chromedriver_win32\chromedriver.exe" #Windows
executable_path = '/path/to/ChromeDriver' # mac
# Add Path to Chrome Driver
driver = webdriver.Chrome(executable_path=executable_path)
time.sleep(3) # Wait for 3 seconds, so that you can see something.
driver.close()
Install Selenium Web Driver with Python webdriver_manager
The simplest way to install the Selenium Chromedriver is to install the webdriver_manager
in Python.
The webdriver_manager
supports multiple browsers.
ChromeDriver
,GeckoDriver
,EdgeChromiumDriver
,IEDriver
,OperaDriver
The webdriver_manager
will manage your browsers.
Use pip
to install webdriver_manager
.
$ pip3 install webdriver-manager
Once webdriver_manager
installed, you can install the chrome driver using ChromeDriverManager
. This operation will take some time.
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
# Install Webdriver
service = Service(ChromeDriverManager().install())
Once the web driver installed, you can start using Selenium.
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
import time
# Install Webdriver
service = Service(ChromeDriverManager().install())
# Create Driver Instance
driver = webdriver.Chrome(service=service)
# Get Web Page
driver.get('https://www.crawler-test.com')
time.sleep(1)
driver.quit()
If you have successfully installed and run Selenium, you can skip straight away to learning how to use Selenium.
Conclusion
Now that you have installed your Chrome Driver Manager, head back to our tutorial on Web Scraping with Selenium in Python.
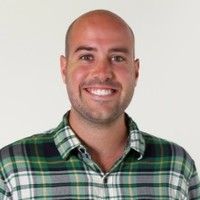
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.