This post is part of the complete Guide on Python for SEO
This easy tutorial will show you how to send an email using python and the Gmail API.
Learning this will be really valuable to create automated tasks or send weekly SEO reports to yourself, your boss or your clients.
Basic Steps to Send an Email With Python
This tutorial is really the easiest way to send an email via Python. It is not, however, the best way. It is preferable to use the Gmail API.
Import Libraries and Set Gmail
import smtplib
from email import encoders
from email.header import Header
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email.mime.image import MIMEImage
from email.mime.multipart import MIMEMultipart
To set-up Gmail ready to send an email, allow less secure app in your Gmail Account.
Set-up your email credentials
to_addr = ["email@example.com","email2@example.com"]
from_addr = "youremail@gmail.com"
password = "your_gmail_password"
smtp='smtp.gmail.com'
Create your email body with HTML
Here I created an example using images, links and {dynamic variables}
.
html=('<html><body><h1>Latest Backlink Report From GSC</h1>' +
'<p>The number of links have had a variation of <b><i>{latest_variation}</i></b> compared to <b><i>last week</i></b>.<br>'+
'and of <b><i>{all_time_variation}</i></b> compared to <b><i>2019-10-30</i></b>.</p>'+
'<img src="cid:image1"><br>'+
'<h2>Latest Link Extractions</h2>'+
'<p>These are the 10 latest link extraction. <a href="https://drive.google.com/file/d/xxxxxxxxxxxx">View the entire report</a></p>'+
'{latest_links}'+
'<h2>Top Five Values</h2>'+
'{topval}'+
'</body></html>').format(latest_variation=latest_variation,all_time_variation=all_time_variation,latest_links=links_backlog.tail(10).to_html(),topval=topval.to_html())
Send the Email
for i in range(len(to_addr)):
msgRoot = MIMEMultipart('related')
msgRoot['Subject'] = 'Latest Backlink Report From GSC'
msgRoot['From'] = from_addr
msgRoot['To'] = to_addr[i]
msgRoot.preamble = 'This is a multi-part message in MIME format.'
# Encapsulate the plain and HTML versions of the message body in an
# 'alternative' part, so message agents can decide which they want to display.
msgAlternative = MIMEMultipart('alternative')
msgRoot.attach(msgAlternative)
msgText = MIMEText('This email was sent from Python')
msgAlternative.attach(msgText)
# We reference the image in the IMG SRC attribute by the ID we give it below
msgText = MIMEText(html, 'html')
msgAlternative.attach(msgText)
# This example assumes the image is in the current directory
fp = open(imgpath, 'rb')
msgImage = MIMEImage(fp.read())
fp.close()
# Define the image's ID as referenced above
msgImage.add_header('Content-ID', '<image1>')
msgRoot.attach(msgImage)
#Send Email
s = smtplib.SMTP(smtp, 587)
s.starttls()
s.login(from_addr, password)
s.sendmail(from_addr, to_addr[i], msgRoot.as_string())
s.quit()
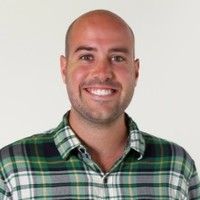
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.