In this extensive guide, you will learn everything that you need to use the Twitter API with Python. I will cover the most important points (and missing points) from Twitter API documentation.
You will learn:
- How to apply for a Twitter Developer account
- How to get Twitter API credentials
- How to authenticate the Twitter API
- How to make Twitter API requests with examples
- How to get tweets from Twitter API
- How to post a Tweet using the Twitter API and Tweepy
Getting Started
For this tutorial, you will need to:
- Install Python
- Have an understanding of Python basics
- Have a Twitter Account. Preferably not your main account as you will experiment a few things.
Get Access to the Twitter API
Apply for an API Access
To use the Twitter API, you will need to: apply for a Twitter Developer Account.
To apply for a Twitter developer account, go to the Twitter Developer console and “Apply for access”, fill out the forms.
This step can take a while.
Thus, I have shared examples of the answers that I used to apply for the Twitter API.
Create Twitter API Credentials
Most partner APIs require credentials to interact with them. They are like the username and password for the API.
You will need to get your Twitter API credentials.
To do so:
- Go to the Twitter Developer Portal
- Create a project
- Get the API Keys
- Generate your access token and secrets
- Set the permission to read and post
- Save the credentials somewhere safe.
Great, now let’s authenticate the API using Tweepy
Introduction to Tweepy
Tweepy is a free Python wrapper that makes it easier to authenticate and interact with the Twitter API.
To use Tweepy in Python, you will need to install the library.
$ pip install tweepy
or with Anaconda:
$ conda install -c conda-forge tweepy
Authenticate the Twitter API with Tweepy
Now that you have access to the Twitter API, we will learn to use it. There is a complete tutorial on how to Authenticate the Twitter API with Python that I will summarize here.
There are many different variables that can be used when authenticating, whether you are building an application or simply running a Python script.
Below is a simple authentication with Python:
import tweepy
# API keyws that yous saved earlier
api_key = "..."
api_secrets = "..."
access_token = "..."
access_secret = "..."
# Authenticate to Twitter
auth = tweepy.OAuthHandler(api_key,api_secrets)
auth.set_access_token(access_token,access_secret)
api = tweepy.API(auth)
try:
api.verify_credentials()
print('Successful Authentication')
except:
print('Failed authentication')
Read my post for more detail on advanced Twitter API authentication.
How to make Twitter API requests (with examples)
There are tons of things that you can do with the Twitter API:
- List a user’s latest posts
- Show followers of a users
- Add users to lists
- Follow other users
- Post tweets
- Get tweets’ statistics
- Update your profie
- And more
I have a tutorial on the basic Twitter API request examples that you may need.
Below is an overview:
user = api.get_user('ChouinardJC') # Store user as a variable
# Get user Twitter statistics
print(f"user.followers_count: {user.followers_count}")
print(f"user.listed_count: {user.listed_count}")
print(f"user.statuses_count: {user.statuses_count}")
# Show followers
for follower in user.followers():
print('Name: ' + str(follower.name))
print('Username: ' + str(follower.screen_name))
# Follow a user
api.create_friendship('ChouinardJC')
# Get tweets from a user tmeline
tweets = api.user_timeline(id='ChouinardJC', count=200)
tweets_extended = api.user_timeline(id='ChouinardJC', tweet_mode='extended', count=200)
print(tweets_extended)
# Like or retweet tweets
id = 'id_of_tweet' # tweet ID
tweet = api.get_status(id) # get tweets with specific id
tweet.favorite() # Like a tweet
tweet.retweet() # Retweet
How to Post Tweets using Twitter API
Posting tweets with the Twitter API can be done in different ways:
- Tweeting
- Retweeting
- Quoting a tweet
- Mention users
- Tweeting an image
- …
I have a complete tutorial on posting tweets to the Twitter API with Tweepy and Python, below is an overview.
Remember, you will be posting to Twitter. Make sure that you are aware that your followers may see your posts.
Text Post
# Text Post
status = "This is my first post to Twitter using the API. I am still learning, please be kind :)"
api.update_status(status=status)
Mention a user
# Post with Mention
mention = 'ChouinardJC'
post_title = 'Post on Twitter API using Tweepy and Python'
status = f"I managed to use the Twitter API thanks to @{mention} tutorial!!!\n\n{post_title}"
api.update_status(status=status)
Link post
# Link post
link = 'https://www.jcchouinard.com/twitter-api/'
mention = 'ChouinardJC'
status = f'I am learning how to post to the Twitter API using #python by reading @{author} post. Ths post was done with the #api!\n {link}"
api = authpy(credentials)
api.update_status(status=status)
Quote a Tweet
author = 'ChouinardJC'
tweet_id = 'XXXXX'
link = f'https://twitter.com/{author}/status/{tweet_id}'
status = f"With this tutorial, I learned how to quote a tweet using the API and #python! @{author}\n{link}"
api.update_status(status=status)
Get Tweets and Understand the JSON Response
You can query a lot of data with the Twitter API. I have a complete post to help you make sense of the Twitter API JSON’s response.
Below is an overview:
# Get a list of tweets
tweets = api.user_timeline(id='ChouinardJC', count=200)
tweets_extended = api.user_timeline(id='ChouinardJC', tweet_mode='extended', count=200)
# Show one tweet's JSON
tweet = tweets[0]
tweet._json
Here are a few functions to parse Twitter API’s JSON.
# Get tweet data
print(f'Created at:{tweet.created_at}')
print(f'Tweet id:{tweet.id}')
# Get tweet text
try:
print(f'Tweet text:{tweet.text}')
except:
# Get tweet text in extended mode
print(f'Tweet text:{tweet.full_text}')
# Get tweet status
print(f'Is Retweeted:{tweet.retweeted}')
print(f'Is favorited:{tweet.favorited}')
print(f'Is quote:{tweet.is_quote_status}')
# Get Tweet statistics
print(f'Retweet count:{tweet.retweet_count}')
print(f'Favorite count:{tweet.favorite_count}')
# Get tweet lang
print(f'Tweet lang:{tweet.lang}')
# Get replied to
print(f'In reply to status id:{tweet.in_reply_to_status_id}')
print(f'In reply to user id:{tweet.in_reply_to_user_id}')
Conclusion
This is the end of this tutorial on using the Twitter API with Python and Tweepy. If you enjoyed it, please share the link, if you haven’t already done it through the API :)!
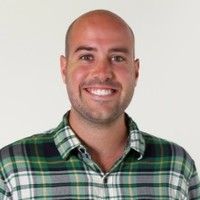
SEO Strategist at Tripadvisor, ex- Seek (Melbourne, Australia). Specialized in technical SEO. Writer in Python, Information Retrieval, SEO and machine learning. Guest author at SearchEngineJournal, SearchEngineLand and OnCrawl.